The Power Iteration Algorithm in Practice
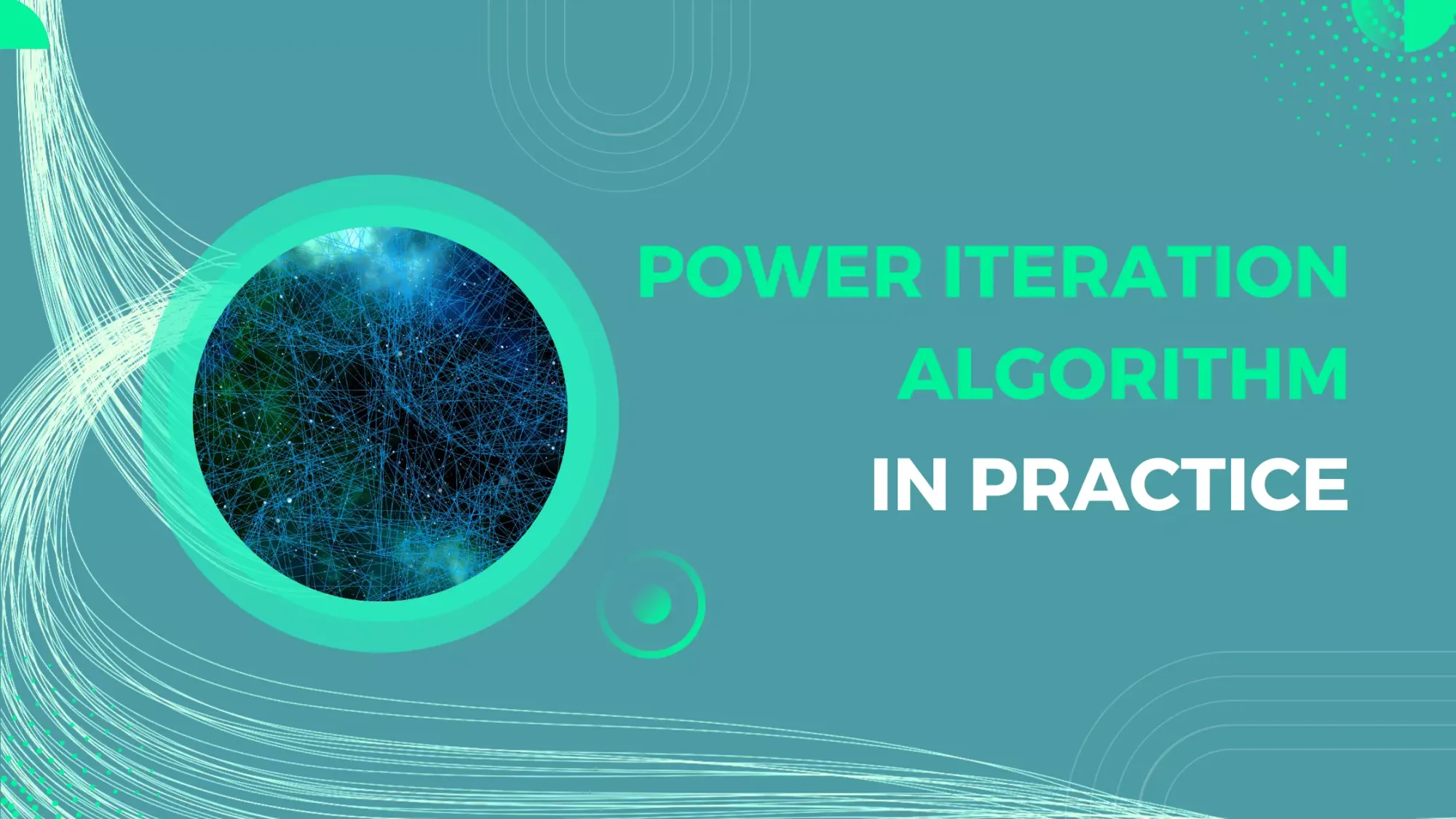
The Power Iteration Algorithm In Practice
The Power Iteration algorithm, also known as the Von Mises iteration, is a simple yet powerful tool in linear algebra. It is used to compute the dominant eigenvector of a matrix and its corresponding eigenvalue. The algorithm has a wide range of applications from physics and engineering to computer science, including image analysis, spectral graph theory, and Google's PageRank algorithm.
Introduction to Eigenvalues and Eigenvectors
Before delving into the Power Iteration algorithm, let's briefly review what eigenvalues and eigenvectors are. Given a square matrix \(\mathbf{A}\), a non-zero vector \(\mathbf{v}\) is an eigenvector of \(\mathbf{A}\) if it satisfies the following equation:
\[\mathbf{A}\mathbf{v} = \lambda \mathbf{v}\]
Here, \(\lambda\) is a scalar known as the eigenvalue corresponding to the eigenvector \(\mathbf{v}\). The intuition is that multiplication by \(\mathbf{A}\) only changes the magnitude (by a factor of \(\lambda\)) and possibly the direction of the vector \(\mathbf{v}\) but does not change the space in which \(\mathbf{v}\) lies.
The Power Iteration Algorithm
Now that we've covered what eigenvectors and eigenvalues are, let's explore the Power Iteration method. The algorithm is based on a simple, yet profound, idea: if you start with a random vector, multiply it by the matrix repeatedly, and normalize it at each step, the vector will converge to the dominant eigenvector of the matrix.
In more formal terms, given a matrix \(\mathbf{A}\), the algorithm begins with a random vector \(\mathbf{b}_0\), and iteratively applies the following steps until convergence:
- Compute \(\mathbf{y} = \mathbf{A}\mathbf{b}_k\)
- Set \(\mathbf{b}_{k+1} = \frac{\mathbf{y}}{|\mathbf{y}|}\)
Here, \(|\mathbf{y}|\) denotes the norm (or length) of the vector \(\mathbf{y}\). Normalizing the vector at each step ensures that the algorithm doesn't diverge.
Once the algorithm converges, the corresponding eigenvalue can be calculated by rearranging the equation \(\mathbf{A}\mathbf{v} = \lambda \mathbf{v}\) to yield \(\lambda = \frac{\mathbf{v}^H\mathbf{A}\mathbf{v} }{\mathbf{v}^H \mathbf{v}}\).
The power iteration method is easy to implement and can be remarkably effective, especially for large, sparse matrices. However, it does have some limitations. It can only find the largest (in absolute value) eigenvalue, and it can be slow to converge for certain matrices.
Python Implementation
Let's see how to implement the power iteration algorithm in Python using the NumPy library:
import numpy as np
def power_iteration(A, num_simulations: int):
# Step 1: Initialize a random vector
b_k = np.random.rand(A.shape[1])
for _ in range(num_simulations):
# Step 2: Compute y = Ab_k
y = np.dot(A, b_k)
# Step 3: Normalize the vector
b_k1 = y / np.linalg.norm(y)
# Step 4: Check for convergence
if np.linalg.norm(b_k1 - b_k) < 1e-6:
break
b_k = b_k1
# compute the corresponding eigenvalue
lambda_k = np.dot(b_k1, np.dot(A, b_k1)) / np.dot(b_k1, b_k1)
return b_k1, lambda_k
This function takes as input a matrix A
and the number of simulations num_iterations
to be performed. It initializes a random vector b_k
, then enters a loop where it multiplies A
by b_k
to create y
, then normalizes y
to
form b_k1
. It checks for convergence by examining the norm of the difference between b_k1
and b_k
. If the difference is less than a small tolerance (in this case, 1e-6
), it breaks out of the loop, signaling that convergence has been achieved. Finally, it computes the corresponding eigenvalue lambda_k
and returns the dominant eigenvector b_k1
and its corresponding eigenvalue.
Step-by-step breakdown of the function
Let's break down the Power Iteration algorithm into smaller steps:
1. Import necessary library: We start by importing the NumPy library, which provides functions for working with arrays and matrices.
import numpy as np
2. Define the function: The function power_iteration
is defined to take in two arguments: a matrix A
and the number of iterations num_iterations
.
def power_iteration(A, num_iterations = 1000):
3. Initialize a random vector: We create a random vector b_k
using the np.random.rand()
function. The size of the vector is the same as the number of columns in matrix A
.
b_k = np.random.rand(A.shape[1])
4. Iteration Loop: The function then enters a loop that runs for the number of simulations specified.
for _ in range(num_simulations):
5. Multiply the matrix and the vector: Inside the loop, we first calculate the dot product of matrix A
and vector b_k
to get a new vector y
.
y = np.dot(A, b_k)
6. Normalize the vector: The vector y
is then normalized (i.e., its length is made to be 1) by dividing it by its norm. This gives us a new vector b_k1
.
b_k1 = y / np.linalg.norm(y)
7. Check for convergence: We check for convergence by comparing the norm (length) of the difference between b_k1
and b_k
to a small number (here, 1e-6
). If the difference is smaller than this number, we consider the algorithm to have converged and break out of the loop.
if np.linalg.norm(b_k1 - b_k) < 1e-6:
break
8. Update the vector: If the algorithm has not converged, we update b_k
to be the newly calculated b_k1
and continue to the next iteration.
b_k = b_k1
9. Compute the corresponding eigenvalue: After the loop has ended, the corresponding eigenvalue is computed using the dot product of b_k1
and the product of A
and b_k1
, divided by the dot product of b_k1
with itself. This value is stored in lambda_k
.
lambda_k = np.dot(b_k1, np.dot(A, b_k1)) / np.dot(b_k1, b_k1)
10. Return the dominant eigenvector and its corresponding eigenvalue: Finally, the function returns the dominant eigenvector b_k1
and its corresponding eigenvalue lambda_k
.
return b_k1, lambda_k
In summary, the power iteration function works by initializing a random vector and then repeatedly multiplying it by the given matrix, normalizing the result, and checking for convergence. Once convergence is achieved, it calculates the corresponding eigenvalue and returns both the dominant eigenvector and its eigenvalue.
Use Cases and Applications
As mentioned, the Power Iteration algorithm has a multitude of applications across several domains:
Physics and Engineering: The Power Iteration algorithm is commonly used to solve problems involving dynamics and vibrations. It can also be used in structural engineering to understand how a structure might react to certain forces, particularly when dealing with the structure's natural modes and frequencies.
Google's PageRank Algorithm: PageRank is a link analysis algorithm used by Google that assigns a weight to each element of a hyperlinked set of documents. The Power Iteration method is used to calculate this weight.
Spectral Clustering: In spectral clustering, data points are viewed as nodes of a graph, and the problem of clustering reduces to partitioning the graph into subgraphs. The Fiedler vector, which is the eigenvector corresponding to the second smallest eigenvalue of the Laplacian matrix of the graph, can be computed using Power Iteration and is used to partition the graph.
Principal Component Analysis (PCA): In PCA, one computes the eigenvectors of the covariance matrix of the data to find the directions (i.e., principal components) along which the variation in the data is maximized. The Power Iteration method can be used to compute these eigenvectors.
Conclusion
The Power Iteration algorithm is a simple yet powerful tool for finding the dominant eigenvector and its corresponding eigenvalue. Although it has its limitations, such as only being able to find the largest eigenvalue and potentially being slow to converge, its ease of implementation and use in a wide variety of fields makes it a versatile and valuable algorithm. This article has explored its theory, implementation, and applications, providing a comprehensive overview of this fascinating algorithm.
Further reading
"Linear Algebra Done Right" by Sheldon Axler
This book offers a clear and concise take on linear algebra, emphasizing the understanding of vector spaces over determinants. The book covers eigenvalues and eigenvectors in-depth.
"Introduction to Linear Algebra" by Gilbert Strang
Gilbert Strang's textbook provides an introductory and application-oriented approach to linear algebra with a focus on understanding. It includes excellent sections on eigenvalues and eigenvectors.
"Matrix Computations" by Gene H. Golub and Charles F. Van Loan
This book is a comprehensive resource on numerical linear algebra, including eigenvalue and singular value algorithms like the Power Iteration method. It's more focused on computations and algorithms.
"Numerical Linear Algebra" by Lloyd N. Trefethen and David Bau III
A more advanced book, this work dives into the numerical side of linear algebra, including practical algorithms like Power Iteration.
"PageRank: Beyond the Science of Search" by Amy N. Langville and Carl D. Meyer
This book discusses the mathematics of Google's PageRank algorithm, which uses Power Iteration to rank web pages. The book delves into the underlying linear algebra and the Power Iteration method in the context of this application.