How to Filter Data Frames in R
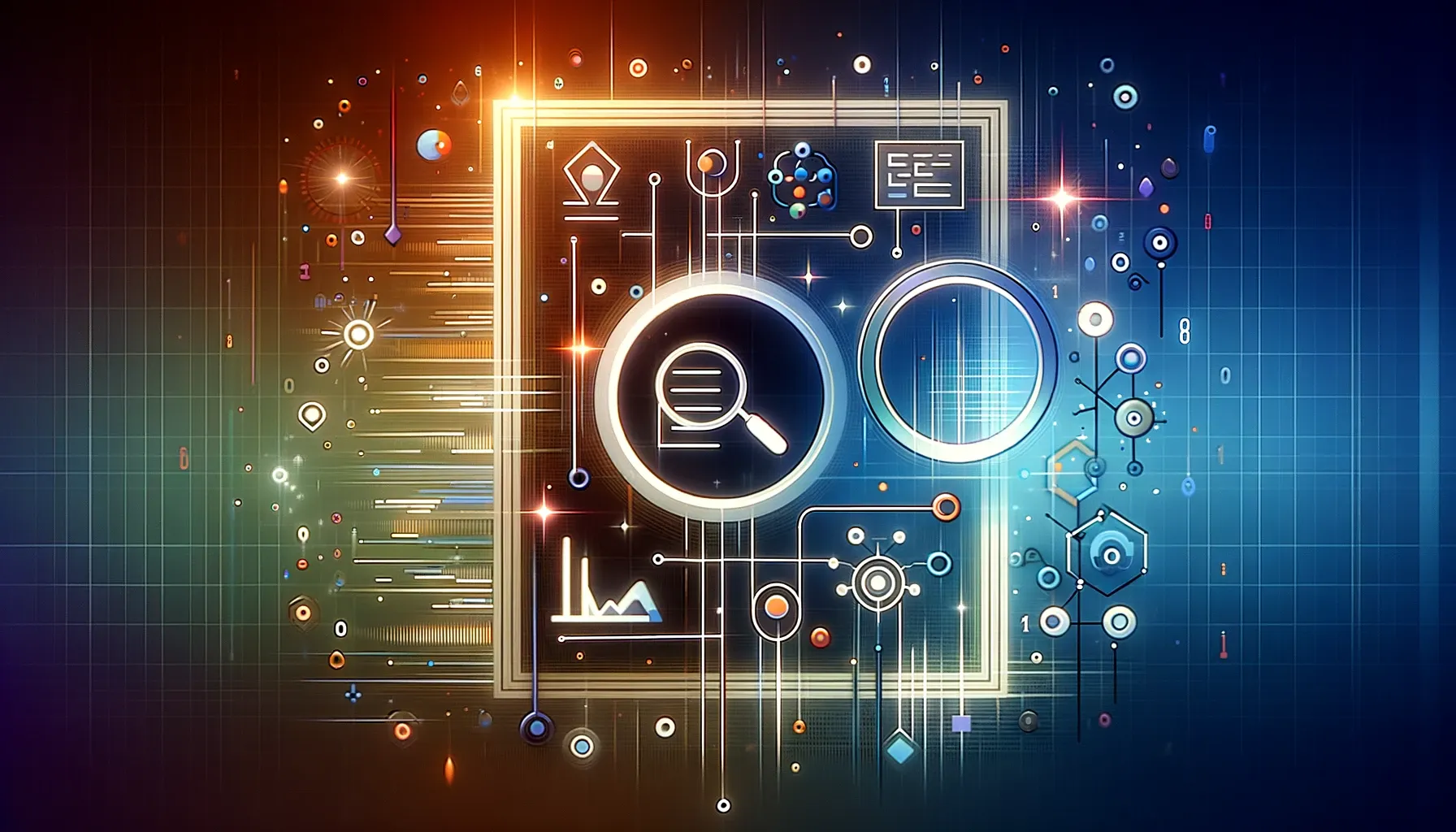
Data frames in R are fundamental components for data analysis, serving as the cornerstone for most data manipulation tasks. Imagine you have a vast dataset, like a spreadsheet with thousands of rows and columns. You want to examine specific subsets based on certain criteria – maybe you’re looking at sales data and want to focus on a particular region or time period. This is where filtering comes in, allowing you to hone in on specific segments of your data for more targeted analysis.
Filtering is indispensable in various scenarios. For instance, a biologist might need to filter experimental data to analyze results from a specific group of samples. A financial analyst, on the other hand, could use filtering to extract stock market data for companies exceeding a certain market cap. By mastering the art of filtering data frames in R, you empower yourself to conduct more efficient, accurate, and insightful data analysis.
Basic Filter Function Usage
The basic filtering in R can be performed using the subset()
function. This function is part of base R, meaning it's built into the R environment and doesn't require any additional packages. The subset()
function takes a data frame and returns a subset of that data frame based on specified conditions.
For detailed information on the subset()
function, you can refer to the official R documentation: R Documentation - subset.
Here's the test data created for use in all the examples:
Name | Age | City | Salary | |
---|---|---|---|---|
0 | Alice | 25 | New York | 70000 |
1 | Bob | 30 | Los Angeles | 80000 |
2 | Charlie | 35 | Chicago | 90000 |
3 | David | 40 | Houston | 100000 |
4 | Eva | 45 | Phoenix | 110000 |
This data frame consists of five rows and four columns: 'Name', 'Age', 'City', and 'Salary'. It represents a simple dataset with varied data types suitable for demonstrating various filtering techniques in R.
# Creating a data frame
df <- data.frame(
Name = c('Alice', 'Bob', 'Charlie', 'David', 'Eva'),
Age = c(25, 30, 35, 40, 45),
City = c('New York', 'Los Angeles', 'Chicago', 'Houston', 'Phoenix'),
Salary = c(70000, 80000, 90000, 100000, 110000)
)
# Display the data frame
print(df)
Basic Examples
Filtering Based on One Condition:
To select rows where a specific column meets a condition:
filtered_data <- subset(your_dataframe, column_name == 'desired_value')
For example, if we wanted to choose only results from New York
, we would write
filtered_data <- subset(df, City == 'New York')
print(filtered_data)
Which would give us
Name Age City Salary
1 Alice 25 New York 70000
Filtering with Numeric Conditions:
For example, to filter rows where a numeric column is greater than a certain value
Let's try it by choosing people with salary more than 90000.
filtered_data <- subset(df, Salary > 90000)
print(filtered_data)
This should give us the following
Name Age City Salary
4 David 40 Houston 100000
5 Eva 45 Phoenix 110000
Combining Conditions:
You can also combine multiple conditions using logical operators
filtered_data <- subset(your_dataframe, column1 == 'value' & column2 > 50)
We can combine the two previous examples by search for people from Houston earning more than 90000.
filtered_data <- subset(df, City == 'Houston' & Salary > 90000)
This yields
Name Age City Salary
4 David 40 Houston 1e+05
Advanced Examples with External Libraries
When it comes to more advanced filtering, external libraries like dplyr
and data.table
offer powerful and flexible options.
- dplyr Package: The
dplyr
package provides afilter()
function that is intuitive and user-friendly. It's part of the tidyverse, a collection of R packages designed for data science. Learn more aboutdplyr
here: dplyr documentation. - data.table Package: For large datasets,
data.table
offers fast and memory-efficient filtering. It's particularly useful for big data applications. Check thedata.table
documentation here: data.table documentation.
Examples with External Libraries
Filtering with dplyr
Choosing people from Houston would go like
library(dplyr)
filtered_data <- df %>% filter(City == 'Houston')
Filtering Multiple Conditions with dplyr
Choosing people from New York with salary less than 100k, would look something like
filtered_data <- df %>% filter(City == 'New York', Salary < 100000)
Using data.table for Fast Filtering
Choosing people from Phoenix with data.table
can be achieved by
library(data.table)
dt = as.data.table(df)
filtered_data <- dt[City == 'Phoenix']
Range Filtering with data.table
Choosing people with salary in between 80k and 100k, would go like
dt = as.data.table(df)
filtered_data <- dt[Salary >= 80000 & Salary <= 100000]
Note that the columns do not need to be the same. We could similarly search for people aged less than 50 with salary more than 50k
dt = as.data.table(df)
filtered_data <- dt[Salary >= 50 & Age < 50]
Complex Filtering with dplyr
Here's a bit more advanced query. Let's look for people aged more than 25 who live either in Los Angeles or Houston
filtered_data <- df %>%
filter(City %in% c('Houston', 'Los Angeles'), Age > 25)
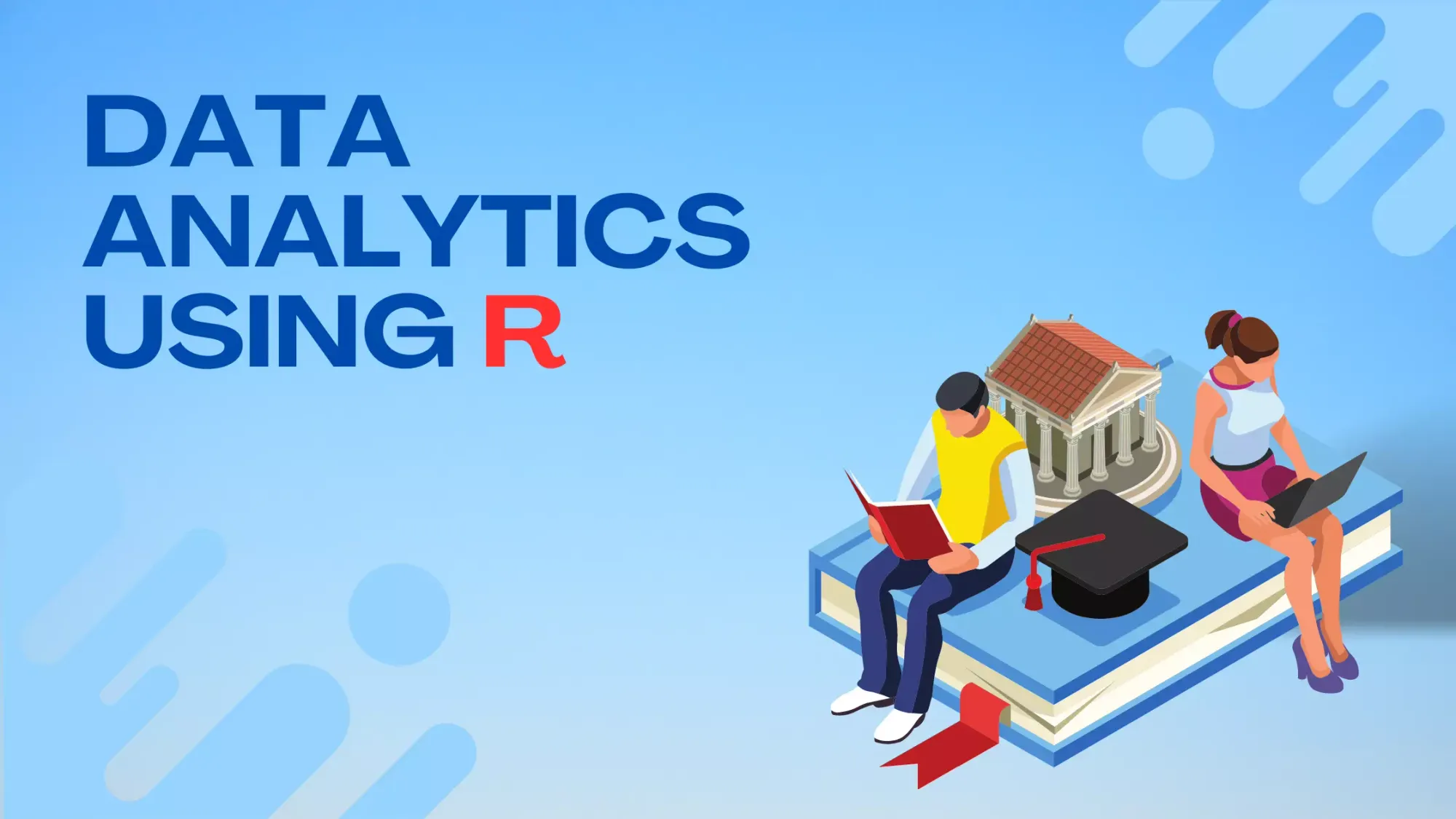
Tips & Tricks
Here are some tips and tricks for filtering data frames in R, which can make your data manipulation tasks more efficient and effective:
- Use Tidyverse Syntax for Clarity: When using
dplyr
, leverage its syntax to make your code more readable. The%>%
operator, known as the pipe, helps in creating a clear, logical flow of data manipulation steps. - Utilize the
slice()
Function: For quickly accessing rows by their position,dplyr
'sslice()
can be more intuitive than traditional indexing. It's especially handy when combined with sorting functions. - Speed Up Operations with
data.table
: If you're dealing with large datasets,data.table
can significantly enhance performance. Its syntax is different but offers faster processing for big data. - Combine
filter()
withselect()
: Indplyr
, usefilter()
andselect()
together to not only filter rows but also to choose specific columns, simplifying your dataset quickly. - Use
filter_if()
for Conditional Filtering: When you need to apply a filter condition across several columns,dplyr
'sfilter_if()
allows you to implement conditions dynamically. - Regular Expressions with
grepl()
: For filtering based on pattern matching in strings, usegrepl()
within your filter conditions. It's a powerful tool for complex string patterns. - Leverage Logical Operators Effectively: Don't forget to use logical operators (
&
,|
,!
) wisely. They can be combined to create complex filtering conditions. - Use
na.omit()
to Handle Missing Data: When your dataset contains NA values,na.omit()
can be used to quickly remove rows with missing data, ensuring your filters work on complete cases. - Benchmarking with
microbenchmark
: When performance matters, use themicrobenchmark
package to compare the speed of different filtering approaches. - Keep Learning with R Documentation: Always refer to R's extensive documentation and community forums for new functions and packages that can improve your data filtering techniques.
Remember, the more you practice and explore, the more proficient you'll become in manipulating and analyzing data in R!
Summary
Filtering data frames in R is a fundamental skill for data analysis. Starting with basic functions like subset()
, you can handle many common data filtering tasks. However, for more advanced and efficient operations, especially with large datasets, turning to external libraries like dplyr
and data.table
is highly beneficial. By mastering both basic and advanced filtering techniques, you can significantly enhance your data manipulation and analysis capabilities in R. Whether you're a beginner or an experienced R user, these tools are essential in your data science toolkit.