Rust Programming: Mastering Vectors
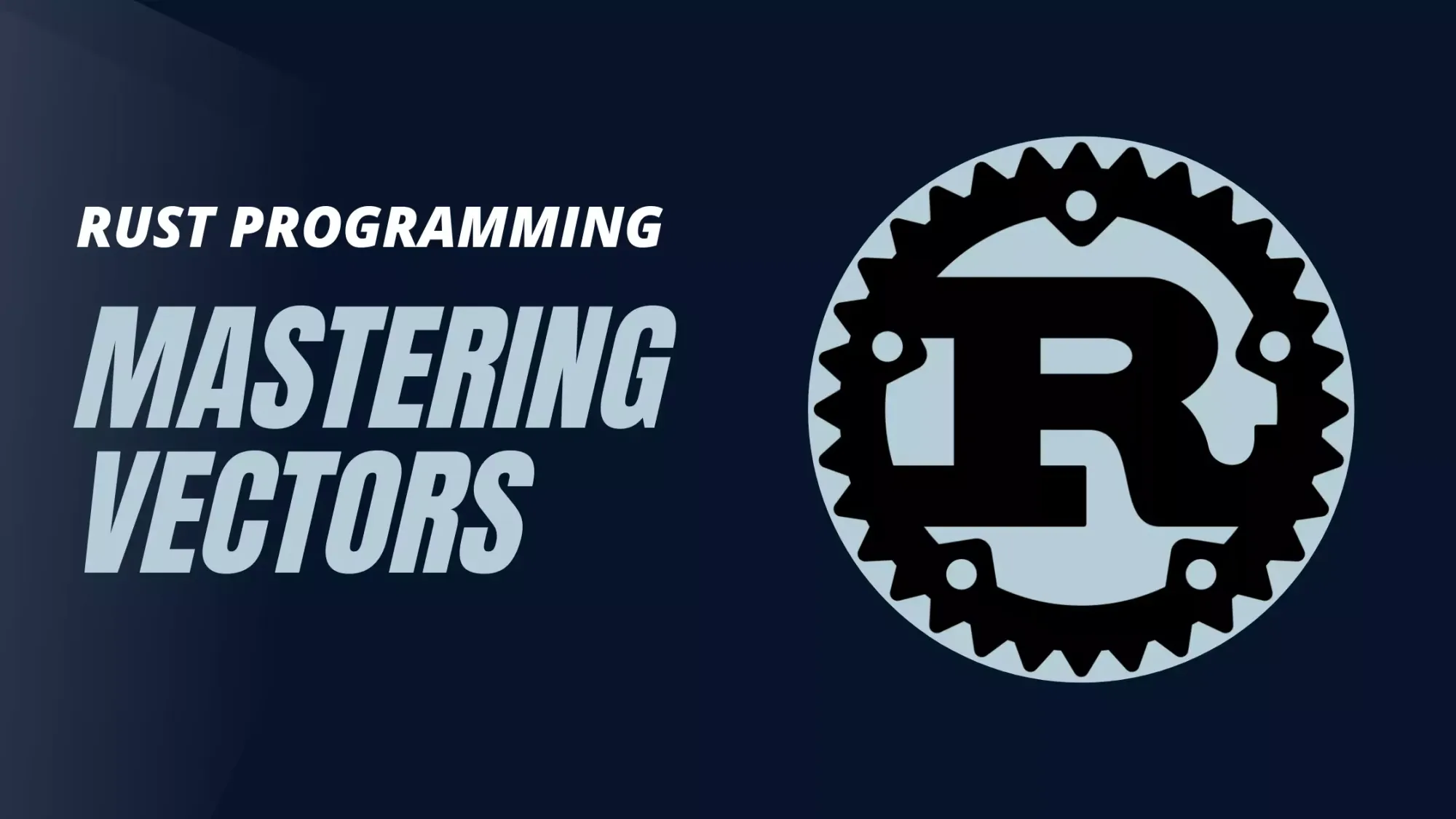
Rust is a modern, highly concurrent, and safe systems programming language that offers high performance with minimal overhead. One of the essential data structures provided by Rust are vectors.
Vectors, in Rust, are dynamic arrays, akin to List in Python or ArrayList in Java. They allow you to have a variable-sized list of elements of the same type. While arrays in Rust are fixed-size collections, vectors offer more flexibility by growing and shrinking their size dynamically.
In this article, we'll cover:
- How to declare a vector
- How to add elements to a vector
- How to remove elements from a vector
- How to access vector elements
- How to iterate over vectors
- How to count the number of elements in vectors (vector length)
- How to sum elements in vectors
- How to add vectors together element-wise
- How to calculate the dot product between two vectors
- How to calculate the norm of a vector
Let's get started.
1. Declaring a Vector
Vectors are declared using Vec<T>
, where T
is the type of the elements. You can create an empty vector with the new
function or a pre-filled vector with the vec!
macro.
Here is how you can declare a vector:
let v: Vec<i32> = Vec::new(); // An empty vector of i32
let v = Vec::new(); // An empty vector, Rust can infer the type later
let v = vec![1, 2, 3]; // A vector containing the elements 1, 2, and 3
2. Adding Elements to a Vector
To add elements to a vector, you use the push
method. Let's add some elements to our previously empty vector.
let mut v = Vec::new(); // 'mut' is necessary for modifying the vector
v.push(5);
v.push(6);
v.push(7);
The vector v
now contains the elements 5, 6, and 7.
3. Removing Elements from a Vector
Elements can be removed from the end of the vector using the pop
method. This method returns Some(value)
if there is a value to pop and None
if the vector is empty.
let mut v = vec![1, 2, 3];
let last_element = v.pop(); // returns Some(3), v now equals to [1, 2]
4. Accessing Vector Elements
You can access vector elements using indexing or the get
method. Indexing is done using square brackets, [index]
. The get
method returns Some(&value)
if the value exists at the specified index and None
if it doesn't.
let v = vec![1, 2, 3];
let second = v[1]; // equals to 2, panic if index out of range
let second = v.get(1); // equals to Some(&2), return None if index out of range
5. Iterating Over Vectors
You can iterate over the elements of a vector using a for
loop. You can iterate over immutable references to preserve the original vector or mutable references to modify the vector elements.
Here is an example of each:
let v = vec![1, 2, 3];
// Immutable iteration
for i in &v {
println!("{}", i);
}
// Mutable iteration
let mut v = vec![1, 2, 3];
for i in &mut v {
*i += 50;
} // v now equals to [51, 52, 53]
Remember, when iterating over mutable references, you need to dereference the value with *
to get access to the value itself.
6. Counting Elements in Vectors (vector length)
Counting the elements in a vector is simple in Rust. You can use the len
method which returns the number of elements in the vector.
let v = vec![1, 2, 3, 4, 5];
let count = v.len();
println!("The vector has {} elements.", count); // Prints "The vector has 5 elements."
7. Summing Elements in Vectors
There are multiple ways to sum the elements in a vector in Rust. One of the easiest ways is to use the iter
method in conjunction with the sum
method. The iter
method returns an iterator over the elements of the vector, and the sum
method sums up the elements. Note that this only works with vectors that contain numbers.
Here's an example:
let v = vec![1, 2, 3, 4, 5];
let sum: i32 = v.iter().sum();
println!("The sum of the elements is {}.", sum); // Prints "The sum of the elements is 15."
It's important to specify the type of the sum
variable (i32
in this case), because Rust's type inference needs to know the numerical type you are summing into.
If you need more control during the summing operation, for example to perform a more complex operation on each element, you could use a for
loop:
let v = vec![1, 2, 3, 4, 5];
let mut sum = 0;
for i in &v {
sum += i;
}
println!("The sum of the elements is {}.", sum); // Prints "The sum of the elements is 15."
In this example, we're using a for
loop to iterate over the elements of the vector and add each element to sum
. Note the use of &v
instead of v
- this borrows the vector for iteration, instead of consuming it. This way, v
is still accessible after the loop.
8. Adding vectors together element-wise
To add two vectors together element-wise, you'll need to iterate over both of them simultaneously. Rust's iter
method, combined with the zip
function, is an efficient way to achieve this.
The zip
function takes two iterators and combines them into a single iterator over pairs of elements. You can then use this iterator in a for
loop or a map
function.
Here is an example:
let v1 = vec![1, 2, 3];
let v2 = vec![4, 5, 6];
let mut result = Vec::new();
for (a, b) in v1.iter().zip(v2.iter()) {
result.push(a + b);
}
println!("{:?}", result); // Prints "[5, 7, 9]"
In this code, we're creating a new vector, result
, to hold the results. We then iterate over v1
and v2
at the same time using zip
, adding corresponding elements together and pushing the result into the result
vector.
You can achieve the same with map
and collect
methods:
let v1 = vec![1, 2, 3];
let v2 = vec![4, 5, 6];
let result: Vec<_> = v1.iter().zip(v2.iter()).map(|(a, b)| a + b).collect();
println!("{:?}", result); // Prints "[5, 7, 9]"
In this version, we're using the map
function to apply a function to each pair of elements. This function just adds the elements together. The collect
function then gathers these results into a new vector.
Note: This code assumes that v1
and v2
have the same length. If they don't, the zip
function will stop at the end of the shorter vector.
9. Dot product between two Rust vectors
The dot product of two vectors is the sum of the products of their corresponding entries. For vectors v1
and v2
, the dot product is calculated as v1[0]*v2[0] + v1[1]*v2[1] + ... + v1[n]*v2[n]
.
Here's how you can implement the dot product in Rust:
let v1 = vec![1, 2, 3];
let v2 = vec![4, 5, 6];
let mut dot_product = 0;
for (a, b) in v1.iter().zip(v2.iter()) {
dot_product += a * b;
}
println!("The dot product is {}", dot_product); // Prints "The dot product is 32"
In this code, we're initializing dot_product
to 0 and then iterating over v1
and v2
at the same time using the zip
function. For each pair of elements, we multiply them together and add the result to dot_product
.
You can also use the map
function to perform the multiplication, and then the sum
function to add up the results:
let v1 = vec![1, 2, 3];
let v2 = vec![4, 5, 6];
let dot_product: i32 = v1.iter().zip(v2.iter()).map(|(a, b)| a * b).sum();
println!("The dot product is {}", dot_product); // Prints "The dot product is 32"
Note: This code assumes that v1
and v2
have the same length. If they don't, the zip
function will stop at the end of the shorter vector.
As of my knowledge cutoff in September 2021, the Rust standard library doesn't provide a built-in function to calculate the dot product of two vectors. If you frequently need to do this or other similar operations, you might want to consider using a numerical computing library like ndarray
or nalgebra
that provides higher-level abstractions for working with vectors and matrices.
10. Calculating the norm of a vector
The norm (or length) of a vector is calculated as the square root of the sum of the squares of its components. For a vector v
, the norm is calculated as sqrt(v[0]^2 + v[1]^2 + ... + v[n]^2)
.
Here's how you can calculate the norm of a vector in Rust:
use std::f64;
let v: Vec<f64> = vec![1.0, 2.0, 3.0, 4.0, 5.0];
let mut sum_of_squares = 0.0;
for &i in &v {
sum_of_squares += i.powi(2);
}
let norm = f64::sqrt(sum_of_squares);
println!("The norm of the vector is {}", norm); // Prints "The norm of the vector is 7.416198487095663"
In this code, we're initializing sum_of_squares
to 0.0 and then iterating over v
with a for
loop. For each element in v
, we square it and add the result to sum_of_squares
. After the loop, we take the square root of sum_of_squares
to get the norm.
You can also use the iter
method in combination with the map
and sum
methods to achieve the same result:
use std::f64;
let v: Vec<f64> = vec![1.0, 2.0, 3.0, 4.0, 5.0];
let sum_of_squares: f64 = v.iter().map(|&i| i.powi(2)).sum();
let norm = f64::sqrt(sum_of_squares);
println!("The norm of the vector is {}", norm); // Prints "The norm of the vector is 7.416198487095663"
In this version, we're using the map
function to apply a function to each element of v
. This function squares the element. The sum
function then adds up these squares. After that, we take the square root of sum_of_squares
to get the norm.
As of my knowledge cutoff in September 2021, the Rust standard library does not provide a built-in function to calculate the norm of a vector. If you frequently need to do this or other similar operations, you might want to consider using a numerical computing library like ndarray
or nalgebra
that provides higher-level abstractions for working with vectors and matrices.
Wrapping Up
In this guide, we have ventured deep into the realm of vectors in Rust, exploring the syntax and operations that make this dynamic data structure an indispensable tool in Rust programming. We have learned how to declare vectors, add and remove elements, access elements individually, and iterate through the contents. We've also tackled more advanced topics, like counting elements, summing values, performing element-wise addition, calculating the dot product, and determining the norm of a vector.
Vectors, with their ability to resize and hold elements of the same type, are key to many applications and systems. As such, the practicality of the operations covered in this guide transcends numerous fields in computing. While Rust may not have built-in functions for certain complex operations like calculating the dot product or norm, it offers the flexibility to easily implement these operations manually. Furthermore, external libraries like ndarray
and nalgebra
can be used for more extensive numerical computing. With this newfound knowledge and these tools, you are well on your way to mastering vectors in Rust.