Guide to Combining Dataframes using pandas.concat()
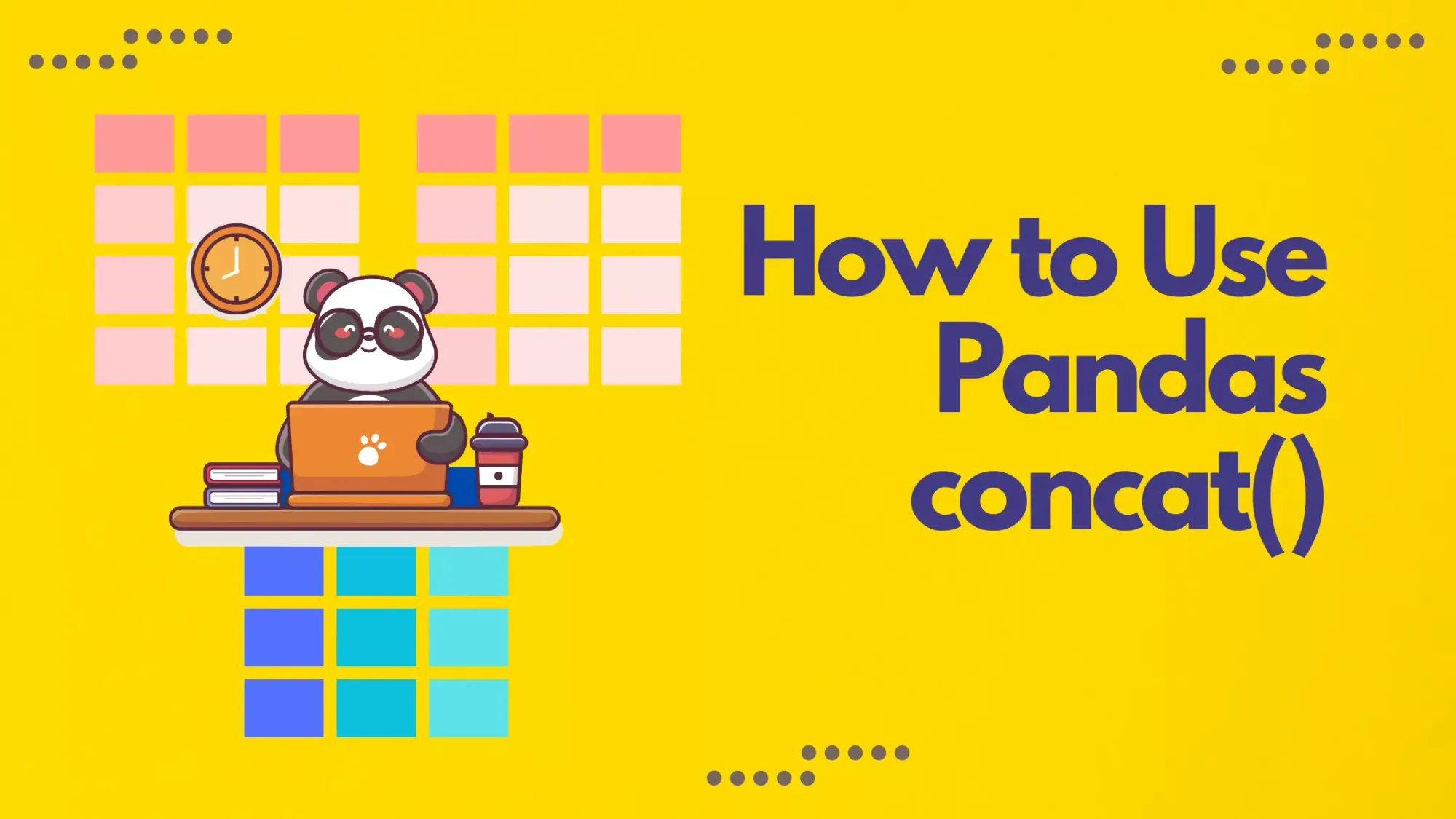
In the realm of data science and analysis, the ability to efficiently manipulate and transform data is paramount. The Python ecosystem, renowned for its vast array of libraries tailored for data tasks, boasts Pandas as one of its crown jewels. Pandas streamlines the process of data wrangling, making the journey from raw data to insightful visualizations and analyses smoother. At the heart of this library, functions like concat()
play a pivotal role, offering flexibility and power in handling data structures.
The pandas.concat()
method is not merely a tool to stitch data together; it's a testament to the library's commitment to versatility. Whether one is piecing together fragments of a dataset, consolidating multiple data sources, or restructuring data for further analysis, concat()
emerges as the go-to function. Its ability to concatenate objects, be they Series or DataFrames, along a specific axis, makes it an indispensable tool for beginners and seasoned professionals. This article aims to shed light on the intricacies of pandas.concat()
, offering insights into its parameters, use cases, and best practices.
What is pandas.concat()
?
The pandas.concat()
function is a foundational tool within the Pandas library that facilitates the combination of two or more Pandas objects. These objects can be Series, DataFrames, or a mix of both. The primary strength of concat()
is its versatility in handling both row-wise (vertical) and column-wise (horizontal) concatenations, offering users a dynamic way to merge data structures based on their needs.
When you invoke the concat()
function, you're essentially "stacking" data structures together. The manner in which they stack—whether they stack vertically or side by side—depends on the specified axis. This is controlled by the axis
parameter, where axis=0
denotes a vertical stack (row-wise) and axis=1
denotes a horizontal stack (column-wise).
Example 1: Row-wise Concatenation
Let's consider two simple DataFrames:
- DataFrame
df1
A | B |
---|---|
A0 | B0 |
A1 | B1 |
- DataFrame
df2
A | B |
---|---|
A2 | B2 |
A3 | B3 |
Concatenating them row-wise using pd.concat([df1, df2])
results in:
A | B |
---|---|
A0 | B0 |
A1 | B1 |
A2 | B2 |
A3 | B3 |
Example 2: Column-wise Concatenation
Using the same DataFrames df1
and df2
, if we concatenate them column-wise using pd.concat([df1, df2], axis=1)
, the result is:
A | B | A | B |
---|---|---|---|
A0 | B0 | A2 | B2 |
A1 | B1 | A3 | B3 |
Note: When concatenating column-wise, it's essential to be aware of duplicate column names, as seen in the example above.
Syntax:
The basic syntax of concat()
is:
pandas.concat(objs, axis=0, join='outer', ignore_index=False, keys=None, sort=False)
objs
: A sequence or mapping of Series or DataFrame objects.axis
: The axis along which concatenation will happen. 0 for row-wise and 1 for column-wise.join
: Determines how to handle overlapping columns. Options include 'outer' and 'inner'.ignore_index
: If True, do not use the index values of the concatenated axis.keys
: Sequence to determine hierarchical indexing.sort
: Sort non-concatenation axis if it is not already aligned.
For an in-depth understanding and exploration of various parameters and examples, it's always a good practice to refer to the official Pandas documentation on concat()
.
Why Use pandas.concat()
?
The pandas.concat()
function stands as one of the cornerstones of the Pandas library, particularly when it comes to combining multiple data structures. It provides a straightforward yet powerful way to concatenate two or more pandas objects along a particular axis, whether they are Series or DataFrames.
Benefits of Using pandas.concat()
- Versatility: It allows for both row-wise (vertical) and column-wise (horizontal) concatenations, making it adaptable to various scenarios.
- Flexibility with Indices: It can handle different indices and provides options to ignore, retain, or create hierarchical indices.
- Join Options: Similar to SQL, it offers 'inner' and 'outer' joins, enabling users to decide how to handle overlapping columns.
- Multiple Object Handling: Unlike some functions that merge or join two DataFrames,
concat()
can handle a list of multiple pandas objects, making batch concatenations simpler.
Row-wise Concatenation
Row-wise concatenation, often referred to as vertical concatenation, involves adding the rows of one DataFrame to another. When performing this type of concatenation, it's essential to ensure that the DataFrames have the same columns or handle any mismatches appropriately.
Example 1: Basic Row-wise Concatenation
Consider two DataFrames with the same columns:
- DataFrame
df3
Name | Age |
---|---|
John | 28 |
Doe | 30 |
- DataFrame
df4
Name | Age |
---|---|
Alice | 24 |
Bob | 22 |
Concatenating them row-wise using pd.concat([df3, df4])
would result in:
Name | Age |
---|---|
John | 28 |
Doe | 30 |
Alice | 24 |
Bob | 22 |
Example 2: Row-wise Concatenation with Different Columns
Now, let's consider two DataFrames with different columns:
- DataFrame
df5
Name | Age |
---|---|
Charlie | 35 |
David | 40 |
- DataFrame
df6
Name | Occupation |
---|---|
Eve | Engineer |
Frank | Doctor |
Concatenating them row-wise using pd.concat([df5, df6], ignore_index=True)
would result in:
Name | Age | Occupation |
---|---|---|
Charlie | 35 | NaN |
David | 40 | NaN |
Eve | NaN | Engineer |
Frank | NaN | Doctor |
Since the DataFrames have different columns, the resulting DataFrame will have NaN values for the missing data.
Row-wise concatenation is a powerful tool to combine datasets, especially when aggregating similar data from different sources or time periods. However, attention to column alignment is crucial to ensure data consistency.
Column-wise Concatenation
Column-wise concatenation, often referred to as horizontal concatenation, involves adding the columns of one DataFrame to another. It's particularly useful when you have data split across multiple sources or files and you want to merge them based on a common index or row order.
Example 1: Basic Column-wise Concatenation
Consider two DataFrames:
- DataFrame
df7
Name | Age |
---|---|
John | 28 |
Doe | 30 |
- DataFrame
df8
Occupation | Salary |
---|---|
Engineer | 70000 |
Doctor | 75000 |
Concatenating them column-wise using pd.concat([df7, df8], axis=1)
would result in:
Name | Age | Occupation | Salary |
---|---|---|---|
John | 28 | Engineer | 70000 |
Doe | 30 | Doctor | 75000 |
Example 2: Column-wise Concatenation with Different Number of Rows
Now, let's consider two DataFrames with different numbers of rows:
- DataFrame
df9
Name | Age |
---|---|
Charlie | 35 |
David | 40 |
Eve | 33 |
- DataFrame
df10
Occupation | Salary |
---|---|
Engineer | 65000 |
Doctor | 68000 |
Concatenating them column-wise using pd.concat([df9, df10], axis=1)
would result in:
Name | Age | Occupation | Salary |
---|---|---|---|
Charlie | 35 | Engineer | 65000 |
David | 40 | Doctor | 68000 |
Eve | 33 | NaN | NaN |
Since the DataFrames have a different number of rows, the resulting DataFrame will have NaN values for the missing data in the additional rows.
Column-wise concatenation is a powerful mechanism when you have datasets that share a common index or row order. However, attention to the number of rows and handling potential mismatches is essential to maintain data integrity.
Hierarchical Indexing
Hierarchical indexing, also known as multi-level indexing, allows for the arrangement of data in a multi-dimensional fashion, using more than one level of index labels. This becomes particularly useful when you're dealing with complex datasets where a single-level index might not suffice. Hierarchical indexing provides a structured form to the data, making it easier to perform operations on subsets of the data.
Example 1: Hierarchical Indexing using Keys
Consider two simple DataFrames:
- DataFrame
df11
A | B |
---|---|
A0 | B0 |
A1 | B1 |
- DataFrame
df12
A | B |
---|---|
A2 | B2 |
A3 | B3 |
By using the keys
parameter in pd.concat()
, we can achieve hierarchical indexing on rows:
result = pd.concat([df11, df12], keys=['x', 'y'])
This would result in:
A | B | |
---|---|---|
x 0 | A0 | B0 |
1 | A1 | B1 |
y 0 | A2 | B2 |
1 | A3 | B3 |
Example 2: Hierarchical Indexing on Columns
Consider two more DataFrames:
- DataFrame
df13
A | B |
---|---|
A0 | B0 |
A1 | B1 |
- DataFrame
df14
C | D |
---|---|
C0 | D0 |
C1 | D1 |
We can achieve hierarchical indexing on columns using the same keys
parameter, but with axis=1
:
result = pd.concat([df13, df14], axis=1, keys=['df13', 'df14'])
This results in:
| | df13 | | df14 | |
| | A | B | C | D |
|-----|-------|-----|-------|-----|
| 0 | A0 | B0 | C0 | D0 |
| 1 | A1 | B1 | C1 | D1 |
Hierarchical indexing provides a structured and organized view of the data, making it easier to perform operations on specific levels or subsets of the data. It's a powerful tool, especially for complex datasets where multi-dimensional indexing becomes a necessity.
Handling Overlapping Columns
When using pandas.concat()
, one might encounter situations where DataFrames have overlapping columns. The way in which these overlapping columns are managed can significantly influence the structure and content of the resulting DataFrame.
Example 1: Default Behavior (Outer Join)
By default, the concat()
function uses an outer join, which means it will include all columns from both DataFrames. For columns that exist in only one DataFrame, the resulting values will be filled with NaN for the missing rows.
Given the DataFrames:
- DataFrame
df15
A | B |
---|---|
A0 | B0 |
A1 | B1 |
- DataFrame
df16
A | C |
---|---|
A2 | C0 |
A3 | C1 |
The concatenated DataFrame using default behavior is:
A | B | C |
---|---|---|
A0 | B0 | NaN |
A1 | B1 | NaN |
A2 | NaN | C0 |
A3 | NaN | C1 |
Example 2: Inner Join
An "inner" join can be specified using the join
parameter. This means that only the columns present in both DataFrames will be retained in the result.
Using the same DataFrames df15
and df16
, and setting join='inner'
, the result is:
A |
---|
A0 |
A1 |
A2 |
A3 |
As seen, only the common column 'A' is retained, and columns 'B' and 'C' that were not common to both DataFrames are excluded.
It's crucial to be aware of how overlapping columns are treated when using pandas.concat()
. Depending on the desired outcome, the appropriate join
parameter should be selected. Always inspect the resulting DataFrame to ensure the data is structured as intended.
Common Pitfalls and Best Practices:
Using pandas.concat()
can simplify many data manipulation tasks, but it also comes with potential pitfalls that can lead to unexpected results or performance issues. Being aware of these pitfalls and following best practices can ensure that you harness the power of concat()
effectively and accurately.
Mismatched Columns or Indices:
Pitfall: When concatenating DataFrames row-wise, if the columns don't match, the resulting DataFrame will have columns filled with NaN values for missing data. Similarly, when concatenating column-wise, mismatched rows will lead to NaN-filled rows.
Best Practice: Always check the alignment of columns (for row-wise concatenation) or indices (for column-wise concatenation) before performing the operation. If mismatches are expected, consider handling NaN values post-concatenation using methods like fillna()
.
Duplicate Indices:
Pitfall: If the DataFrames being concatenated have overlapping indices and ignore_index
is set to False, the resulting DataFrame will have duplicate indices. This can lead to unexpected results in subsequent operations.
Best Practice: Use the ignore_index=True
parameter if the original indices aren't meaningful or necessary. Alternatively, consider using the reset_index()
method before concatenation.
Inefficient Memory Usage:
Pitfall: Concatenating large DataFrames can consume a significant amount of memory, especially if you're creating multiple intermediate concatenated DataFrames in a loop.
Best Practice: For memory-intensive operations, consider optimizing your workflow. Instead of multiple concatenations in a loop, try to concatenate in a single operation. Tools like Dask can be beneficial for very large datasets.
Overlooked join
Parameter:
Pitfall: By default, pandas.concat()
uses an outer join, which means all columns from all DataFrames are included in the result. If the DataFrames have different columns, this can lead to many NaN values.
Best Practice: If you're only interested in columns that are shared across all DataFrames, set join='inner'
. Always inspect the result to ensure no unintentional data loss.
Loss of Data Order:
Pitfall: When using the sort
parameter, the column order might change, leading to a DataFrame structure that's different from what you might expect.
Best Practice: Be cautious when using the sort
parameter. If preserving the original column order is essential, consider manually sorting post-concatenation.
By following best practices and always inspecting the results, you can ensure consistent, efficient, and accurate data manipulations.
Conclusion
The pandas.concat()
function is undeniably a powerhouse in the toolkit of anyone working with data in Python. Its capability to unify multiple data structures, combined with its adaptability across various scenarios, makes it an indispensable asset. As data grows increasingly complex and fragmented across various sources, the need for a robust tool to bring this data together becomes paramount. concat()
rises to this challenge, enabling analysts and data scientists to build comprehensive datasets that form the foundation of insightful analysis.
However, with great power comes responsibility. As users harness the versatility of concat()
, it's crucial to remain vigilant about data integrity. Understanding the nuances of its parameters and being mindful of potential pitfalls will ensure that the merging process is seamless and accurate. Always remember, while tools like concat()
simplify processes, the onus of ensuring meaningful results rests on the user. A combination of the function's capabilities and an informed approach will lead to optimal outcomes in data manipulation tasks.
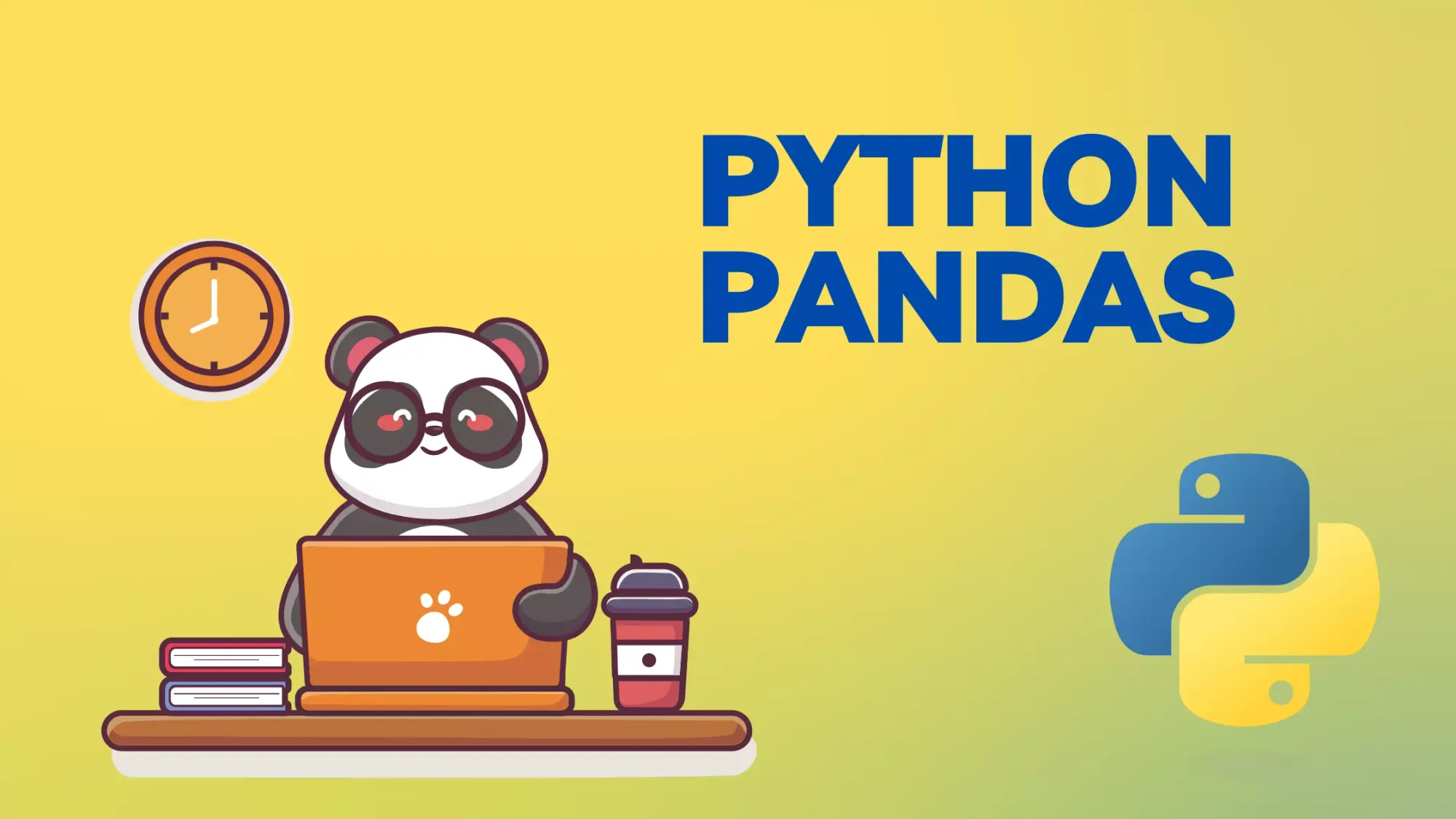