Mastering Iteration: An In-Depth Guide to Looping Techniques in Rust
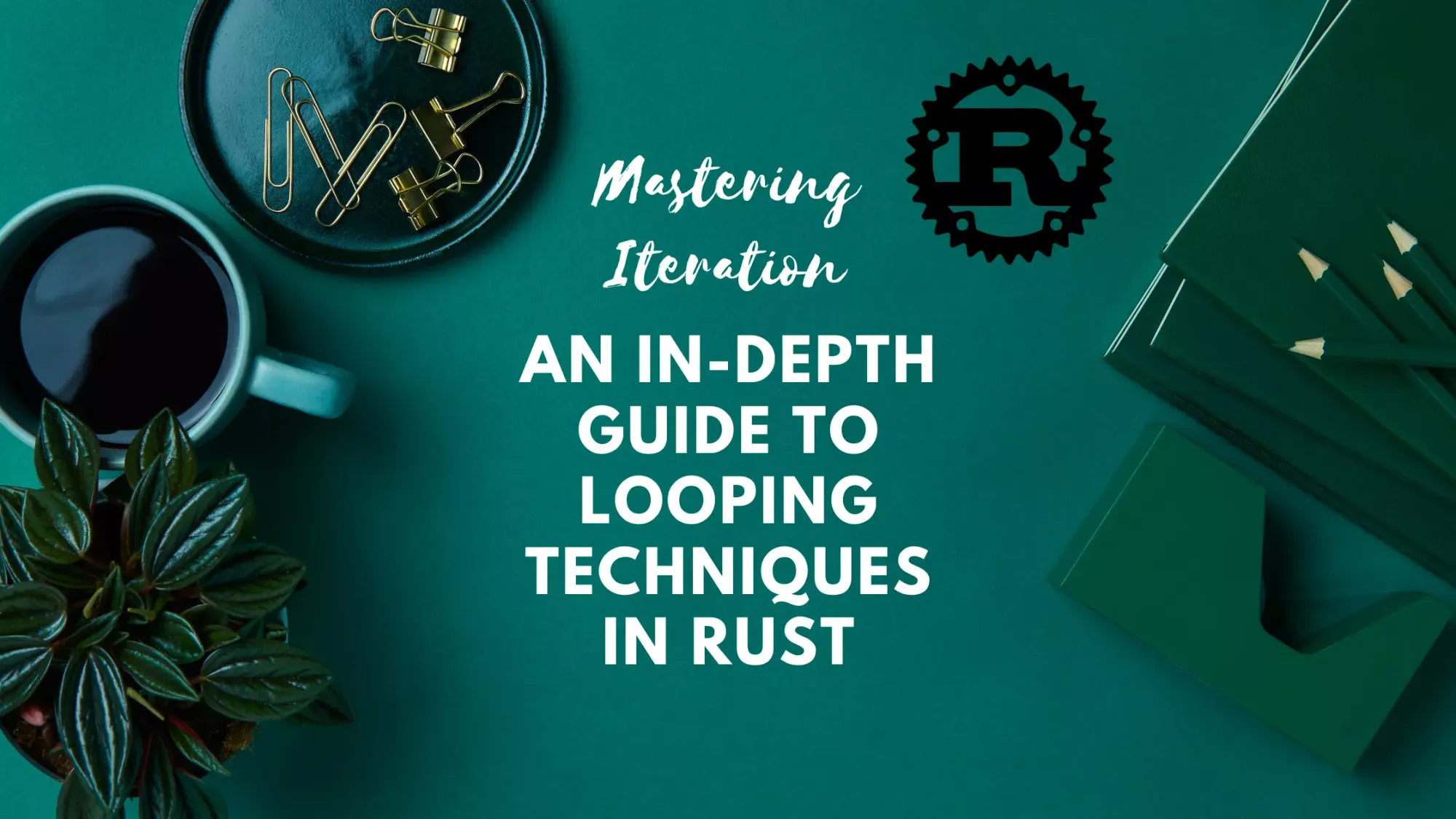
When it comes to programming, iteration or looping is a fundamental building block. Just like many other languages, Rust offers a variety of ways to do loops. In this article, we will dive deep into the various methods of looping in Rust, exploring for loops, while loops, and the loop keyword, as well as more nuanced tools like iterators and functional looping constructs.
Loop
Rust's simplest loop is loop
. It executes the code within the block over and over indefinitely until the code execution is explicitly stopped with a break
.
loop {
println!("This will print forever!");
}
In addition to straightforward, infinite loops, loop
can be combined with the break
keyword to end execution on-demand, and the continue
keyword to skip the remainder of the current iteration and move directly to the next. Here's an example:
let mut counter = 0;
loop {
counter += 1;
if counter == 3 {
continue; // skips printing for 3
}
if counter > 5 {
break; // ends the loop after 5
}
println!("{}", counter);
}
loop
also supports returning values. The value to return is specified after the break
keyword.
let mut counter = 0;
let result = loop {
counter += 1;
if counter == 10 {
break counter * 2; // loop returns 20
}
};
println!("{}", result); // prints 20
Pros:
- Infinite Repetition: It's great when you need a task to run indefinitely until a certain condition is met.
- Returning Values:
loop
supports returning values, which can be useful in various scenarios.
Cons:
- Risk of Infinite Loops: Since
loop
will run indefinitely unless stopped, there's a risk of creating an infinite loop if not carefully managed. - Explicit Exit Required: You must explicitly code for the conditions to exit the loop, which can be error-prone.
While Loop
Rust's while
loop executes a block of code as long as a specified condition is true.
let mut number = 5;
while number != 0 {
println!("{}", number);
number -= 1;
}
Like loop
, while
loops can be controlled with break
and continue
. Remember, however, that break
and continue
in a while
loop apply only to the innermost loop that encloses them.
Pros:
- Condition-Based Looping: Ideal for when you want to repeat an operation as long as a certain condition is true.
- Simplicity: It's straightforward and familiar for programmers coming from other languages.
Cons:
- Potential Performance Issues: If the condition you're checking involves a function call or complex computation, it may be inefficient since that operation is performed on each loop iteration.
For Loop
The for
loop is another looping construct in Rust. This loop is used to iterate over a collection of values, such as arrays, vectors, and ranges.
for number in 1..6 {
println!("{}", number);
}
In this example, 1..6
represents a range that includes 1
but excludes 6
. for
loop is often preferred over while
or loop
because the iterator does the work of encapsulating the termination condition and incrementing the loop counter.
Pros:
- Controlled Iteration: Excellent for iterating over a range of values or elements in a collection. It automatically handles incrementing the loop counter and termination conditions.
- Readability: It's often clearer and more readable than a
while
orloop
, especially for iterating over collections.
Cons:
- Limited Flexibility: It's less flexible than
while
orloop
because the number of iterations is fixed when the loop starts.
Looping Over Collections
You can use for
loop to iterate over collections such as arrays and vectors.
let array = [10, 20, 30, 40, 50];
for element in array.iter() {
println!("{}", element);
}
You can also use enumerate()
function with a for
loop to get the index of the current item along with its value.
let array = [10, 20, 30, 40, 50];
for (index, element) in array.iter().enumerate() {
println!("Value at index {} is {}", index, element);
}
Pros:
- Efficiency: It's a very efficient way to iterate over the elements in collections.
- Ease of Use: The syntax is clear, concise, and easy to use.
Cons:
- Limited to Collections: This method is limited to collections and does not support indefinite or condition-based looping.
Iterators
Rust also provides iterators, which offer a powerful way to process a sequence of elements. An iterator is any type that implements the Iterator
trait. Most collection types in Rust, like vectors and arrays, provide an iterator.
You can call .iter()
method on a collection to get an iterator. By default, this returns an immutable reference to each item. To
get mutable references, you can use .iter_mut()
. If you want to consume the collection and return the owned value, you can call .into_iter()
.
let numbers = vec![1, 2, 3, 4, 5];
let sum: i32 = numbers.iter().sum();
println!("{}", sum); // prints 15
Iterators can be combined with many other methods like map()
, filter()
, fold()
, etc., to perform more complex operations.
Pros:
- Powerful and Flexible: Iterators can be combined with other methods like
map()
,filter()
, etc., to perform complex operations on collections. - Lazy Evaluation: Many iterator methods use lazy evaluation, which can be more efficient as it only computes values as needed.
Cons:
- Complex Syntax: The syntax can be complex, especially for newcomers to Rust or functional programming.
- Learning Curve: Understanding and using iterators effectively can have a steep learning curve.
Functional Looping Constructs
Rust offers a functional approach to loops as well, through methods such as map()
, filter()
, fold()
, etc. These methods take a function or a closure as an argument and use it to perform operations on the elements of a collection.
Here is an example using map()
and collect()
to square all numbers in a vector and store them in a new vector:
let numbers = vec![1, 2, 3, 4, 5];
let squared: Vec<i32> = numbers.iter().map(|x| x * x).collect();
println!("{:?}", squared); // prints [1, 4, 9, 16, 25]
These functional-style methods make your code more concise and easy to understand.
Pros:
- Concise Code: Methods like
map()
,filter()
, etc., can make your code more concise and easy to understand. - Chainability: These methods can be chained together to perform multiple operations in a single line of code.
Cons:
- Performance: In some cases, functional looping constructs may be less performant than traditional loops, especially if the operations are not able to take advantage of optimizations like short-circuiting.
- Learning Curve: If you're not familiar with functional programming, there might be a learning curve to understand these constructs.
Conclusion
Rust provides an extensive range of looping constructs to cater to various scenarios, making it a highly flexible language for handling iteration. From the basic loop structures of loop
, while
, and for
to the more advanced constructs that allow looping over collections and iterators, Rust ensures that programmers have the appropriate tools at their disposal. Each of these constructs has its own strengths and drawbacks, and understanding these is crucial to leverage them most effectively. It's this diversity in approach that makes Rust powerful, as it accommodates different styles and requirements.
However, the notion of the "best" way to loop in Rust isn't set in stone. It heavily depends on the specific problem at hand, the performance needs of the application, and the personal comfort of the programmer with different coding styles. A simple for
loop might suffice in some cases, while in others, a more complex iterator or functional construct might be more suitable. The key is understanding the nuances of each looping method and applying them judiciously in your code. By doing so, you can harness the full potential of Rust's looping constructs, making your programs more efficient, readable, and robust.