Pandas Merge: The Ultimate Tool for DataFrame Joining
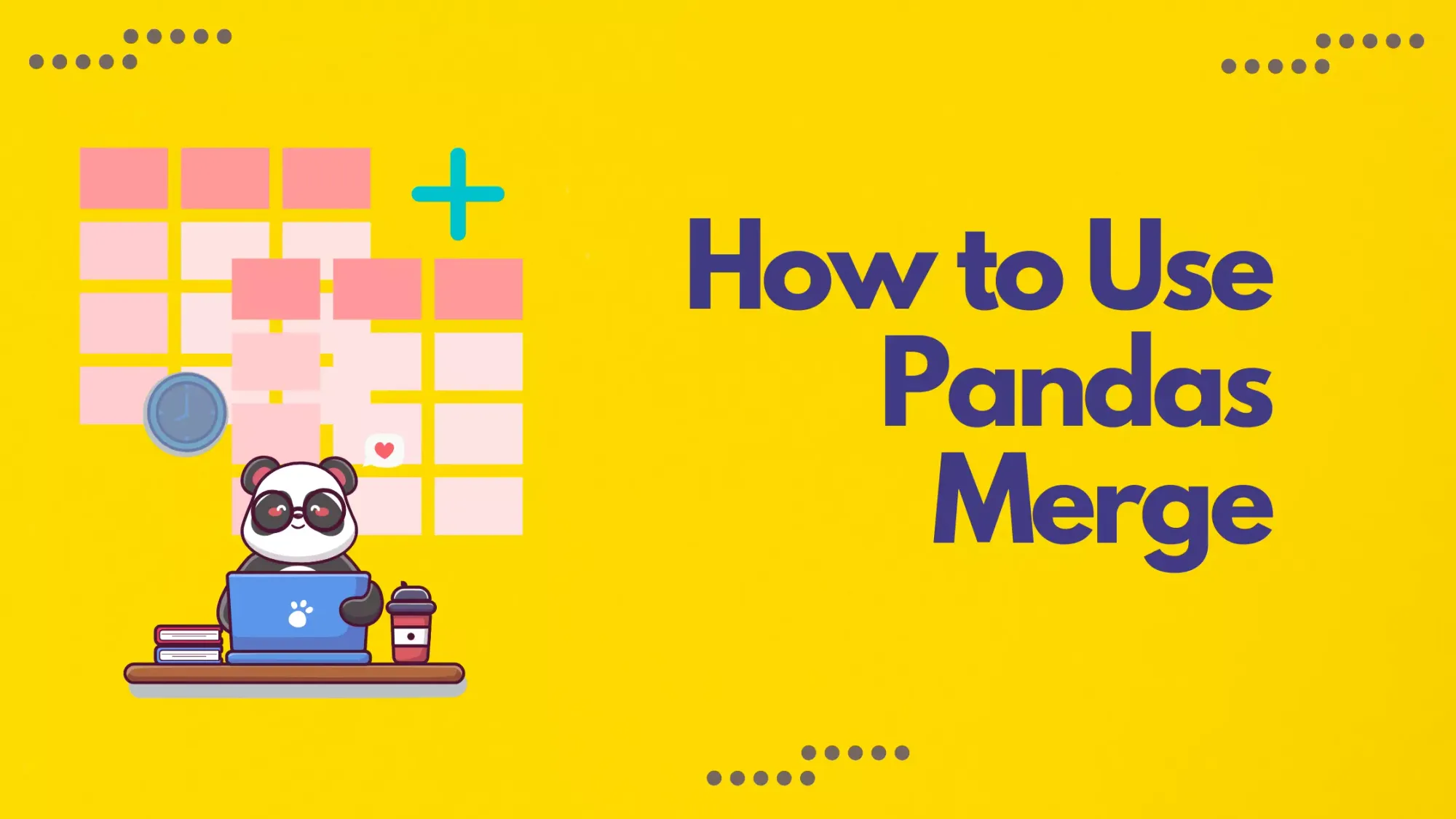
The ability to combine and juxtapose datasets is an invaluable skill. Analysts, researchers, and data enthusiasts frequently encounter scenarios where information is spread across multiple tables or datasets. This dispersion necessitates techniques to collate this data into a coherent and analyzable form.
The Pandas library in Python, revered for its comprehensive suite of data manipulation tools, addresses this need with the merge
function. This function not only facilitates the merging of datasets but also offers a degree of flexibility and precision that is unmatched in the realm of data combination. This article aims to guide readers through the intricacies of the merge
function, ensuring a robust understanding of its capabilities and applications.
Basics of Pandas Merge
The merge
function in Pandas, inspired by database join operations, facilitates the combination of two DataFrames based on one or more keys. The keys can be column names, index levels, or both.
The basic syntax is:
df_merged = pd.merge(df1, df2, on='common_column')
Key Parameters of Pandas Merge
- on: This is the column or index level names to join on. If not passed and
left_index
andright_index
are False, the intersection of the columns in the DataFrames will be inferred as the join keys. - how: This determines the type of merge to be performed. It has options like 'left', 'right', 'outer', and 'inner'.
- left_on: Columns or index levels from the left DataFrame to use as keys.
- right_on: Columns or index levels from the right DataFrame to use as keys.
- left_index: Use the index from the left DataFrame as the join key.
- right_index: Use the index from the right DataFrame as the join key.
- suffixes: Suffix to apply to overlapping column names.
Use Case 1: Basic Merge with 'on' Parameter
We have two DataFrames, df1
and df2
, and we wish to merge them on a common column named key
.
import pandas as pd
df1 = pd.DataFrame({'key': ['A', 'B', 'C', 'D'],
'value_df1': [1, 2, 3, 4]})
df2 = pd.DataFrame({'key': ['C', 'D', 'E', 'F'],
'value_df2': [5, 6, 7, 8]})
merged_df = pd.merge(df1, df2, on='key')
The merged DataFrame looks like this:
key | value_df1 | value_df2 |
---|---|---|
C | 3 | 5 |
D | 4 | 6 |
As observed, the merge operation, by default (an 'inner' join), only includes rows with keys present in both DataFrames.
Use Case 2: Specifying the Type of Join with the 'how' Parameter
We can specify the type of join we want using the how
parameter. Let's perform an 'outer' join on the same DataFrames.
merged_outer = pd.merge(df1, df2, on='key', how='outer')
Executing this will give us a DataFrame that includes all keys from both df1
and df2
. The result of the 'outer' join is:
key | value_df1 | value_df2 |
---|---|---|
A | 1.0 | NaN |
B | 2.0 | NaN |
C | 3.0 | 5.0 |
D | 4.0 | 6.0 |
E | NaN | 7.0 |
F | NaN | 8.0 |
Here, the 'outer' join includes all keys from both DataFrames. When a key is present in one DataFrame but not the other, the missing value is filled with NaN
.
Use Case 3: Merging on Different Columns with left_on
and right_on
Suppose our DataFrames have different column names that we wish to merge on. For instance, df1
has a column named key1
, and df2
has key2
. We can use the left_on
and right_on
parameters for this purpose.
df1 = pd.DataFrame({'key1': ['A', 'B', 'C', 'D'],
'value_df1': [1, 2, 3, 4]})
df2 = pd.DataFrame({'key2': ['C', 'D', 'E', 'F'],
'value_df2': [5, 6, 7, 8]})
merged_diff_cols = pd.merge(df1, df2, left_on='key1', right_on='key2')
The merged result, using different columns from each DataFrame, is:
key1 | value_df1 | key2 | value_df2 |
---|---|---|---|
C | 3 | C | 5 |
D | 4 | D | 6 |
Notice how the resulting DataFrame contains both key1
and key2
columns. An 'inner' join is performed by default, so only keys present in both DataFrames are included.
Use Case 4: Handling Overlapping Column Names with suffixes
When the DataFrames to be merged have overlapping column names, we can distinguish them using the suffixes
parameter.
Let's consider two DataFrames, df1
and df2
, both containing a 'value' column.
df1 = pd.DataFrame({'key': ['A', 'B', 'C', 'D'],
'value': [1, 2, 3, 4]})
df2 = pd.DataFrame({'key': ['C', 'D', 'E', 'F'],
'value': [5, 6, 7, 8]})
merged_suffixes = pd.merge(df1, df2, on='key', suffixes=('_df1', '_df2'))
We'll run this code and observe how the suffixes
parameter helps differentiate overlapping column names.
The merged result, using the suffixes
parameter, is:
key | value_df1 | value_df2 |
---|---|---|
C | 3 | 5 |
D | 4 | 6 |
The suffixes
parameter helps in appending suffixes _df1
and _df2
to the overlapping column names from df1
and df2
, respectively.
The merge
function in Pandas offers a plethora of options to cater to various merging scenarios. Its flexibility, as demonstrated by the use cases above, ensures that users can efficiently merge DataFrames irrespective of their structure or column names. For those keen on diving deeper, the official Pandas documentation offers comprehensive details on all parameters and functionalities of the merge
function.
Handling Non-Matching Column Names
When merging DataFrames, it's common to encounter scenarios where the columns you want to merge on have different names in each DataFrame. This can arise from varied data sources or inconsistent naming conventions. Thankfully, Pandas provides a solution using the left_on
and right_on
parameters.
According to the official Pandas documentation, left_on
and right_on
are used to specify the columns from the left and right DataFrames that should be used as keys for merging.
Let's explore some use cases to better understand how to handle non-matching column names.
Use Case 1: Basic Merge with left_on
and right_on
Consider two DataFrames, df1
with a column named key1
, and df2
with key2
. We want to merge them based on these columns.
df1 = pd.DataFrame({'key1': ['A', 'B', 'C', 'D'],
'value_df1': [1, 2, 3, 4]})
df2 = pd.DataFrame({'key2': ['C', 'D', 'E', 'F'],
'value_df2': [5, 6, 7, 8]})
merged_diff_cols = pd.merge(df1, df2, left_on='key1', right_on='key2')
Let's execute this code to see the merged result.
The merged result, using the left_on
and right_on
parameters, is:
key1 | value_df1 | key2 | value_df2 |
---|---|---|---|
C | 3 | C | 5 |
D | 4 | D | 6 |
As observed, the merged DataFrame retains both key1
and key2
columns. This can be helpful for verification, but in many cases, you might want to drop one of the redundant columns post-merge.
Use Case 2: Merging on Multiple Non-Matching Columns
At times, the merge operation may need to be performed based on multiple columns. Let's consider two DataFrames, df1
with columns key1
and key1_2
, and df2
with key2
and key2_2
.
df1 = pd.DataFrame({
'key1': ['A', 'B', 'C', 'D'],
'key1_2': ['W', 'X', 'Y', 'Z'],
'value_df1': [1, 2, 3, 4]
})
df2 = pd.DataFrame({
'key2': ['C', 'D', 'E', 'F'],
'key2_2': ['Y', 'Z', 'A', 'B'],
'value_df2': [5, 6, 7, 8]
})
merged_multi_cols = pd.merge(df1, df2, left_on=['key1', 'key1_2'], right_on=['key2', 'key2_2'])
Running this code will provide a DataFrame merged on both sets of columns. The merged result, using multiple non-matching columns, is:
key1 | key1_2 | value_df1 | key2 | key2_2 | value_df2 |
---|---|---|---|---|---|
C | Y | 3 | C | Y | 5 |
D | Z | 4 | D | Z | 6 |
The DataFrames are merged based on the combination of key1
with key1_2
and key2
with key2_2
.
Handling non-matching column names in Pandas merge operations can initially seem daunting but with the left_on
and right_on
parameters, it becomes a breeze. These tools allow for flexibility in merging DataFrames that may not have been curated with consistent column naming. By understanding how to use these parameters effectively, one can ensure the integrity and correctness of the merged data. For those keen on diving deeper, the official Pandas documentation provides comprehensive details on these and other functionalities of the merge
function.
Dealing with Overlapping Column Names
When merging DataFrames in Pandas, it's not uncommon to encounter columns other than the join keys that have the same name in both DataFrames. In such scenarios, Pandas provides a mechanism to handle these overlapping column names using the suffixes
parameter.
According to the official Pandas documentation, the suffixes
parameter is used to specify a tuple of string suffixes to append to overlapping column names in the left and right DataFrames, respectively.
Let's explore various use cases to better understand how to handle these overlapping column names.
Use Case 1: Basic Overlapping Column Names with suffixes
Consider two DataFrames, df1
and df2
, both containing a column named value
. We want to merge them based on a key
column but need to differentiate the 'value' columns in the merged DataFrame.
df1 = pd.DataFrame({'key': ['A', 'B', 'C', 'D'],
'value': [1, 2, 3, 4]})
df2 = pd.DataFrame({'key': ['C', 'D', 'E', 'F'],
'value': [5, 6, 7, 8]})
merged_suffixes = pd.merge(df1, df2, on='key', suffixes=('_df1', '_df2'))
The merged result, using the suffixes
parameter to handle overlapping column names is:
key | value_df1 | value_df2 |
---|---|---|
C | 3 | 5 |
D | 4 | 6 |
Here, the overlapping 'value' columns from df1
and df2
are differentiated using the suffixes _df1
and _df2
, respectively.
Use Case 2: Handling Multiple Overlapping Columns
Suppose we have more than one overlapping column in our DataFrames. Let's consider df1
and df2
with columns value1
and value2
that overlap.
df1 = pd.DataFrame({'key': ['A', 'B', 'C', 'D'],
'value1': [1, 2, 3, 4],
'value2': [5, 6, 7, 8]})
df2 = pd.DataFrame({'key': ['C', 'D', 'E', 'F'],
'value1': [9, 10, 11, 12],
'value2': [13, 14, 15, 16]})
merged_multi_overlap = pd.merge(df1, df2, on='key', suffixes=('_df1', '_df2'))
The merged result, handling multiple overlapping columns, is:
key | value1_df1 | value2_df1 | value1_df2 | value2_df2 |
---|---|---|---|---|
C | 3 | 7 | 9 | 13 |
D | 4 | 8 | 10 | 14 |
Both value1
and value2
columns from df1
and df2
are differentiated using the suffixes _df1
and _df2
, respectively.
Handling overlapping column names is a frequent scenario when merging DataFrames with similar structures. The suffixes
parameter in Pandas merge
provides a straightforward and efficient way to distinguish between these columns, ensuring clarity in the merged data. As always, for a deeper dive into the options available and finer details, the official Pandas documentation serves as an invaluable resource.
Merging on Index
In Pandas, DataFrames have an index which is a unique identifier for rows. While it's common to merge DataFrames based on column values, there are scenarios where merging on the index is more appropriate. The Pandas merge
function supports this through the left_index
and right_index
parameters.
According to the official Pandas documentation, left_index
and right_index
are boolean parameters that can be used to indicate if the index should be used as the merge key for the left and right DataFrames, respectively.
Use Case 1: Basic Merge Using Index
Consider two DataFrames, df1
and df2
, and we want to merge them based on their indices.
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2', 'A3']},
index=['K0', 'K1', 'K2', 'K3'])
df2 = pd.DataFrame({'B': ['B0', 'B1', 'B2', 'B3']},
index=['K0', 'K1', 'K2', 'K3'])
merged_on_index = pd.merge(df1, df2, left_index=True, right_index=True)
Executing this code will merge the DataFrames based on their indices. The merged result, using the indices of both DataFrames, is:
A | B | |
---|---|---|
K0 | A0 | B0 |
K1 | A1 | B1 |
K2 | A2 | B2 |
K3 | A3 | B3 |
Both DataFrames are merged based on their shared indices.
Use Case 2: Merging with One DataFrame's Index and Another's Column
There might be situations where you want to merge on the index of one DataFrame and a column of another DataFrame. Let's see how this can be achieved.
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2', 'A3']},
index=['K0', 'K1', 'K2', 'K3'])
df2 = pd.DataFrame({'B': ['B0', 'B1', 'B2', 'B3'],
'key': ['K0', 'K1', 'K2', 'K3']})
merged_index_column = pd.merge(df1, df2, left_index=True, right_on='key')
The merged result, using the index of df1
and the key
column of df2
, is:
A | B | key | |
---|---|---|---|
0 | A0 | B0 | K0 |
1 | A1 | B1 | K1 |
2 | A2 | B2 | K2 |
3 | A3 | B3 | K3 |
The merged DataFrame contains both the index from df1
and the key
column from df2
.
Merging on index provides an alternative way to combine DataFrames when column-based merging isn't appropriate. Whether merging on the indices of both DataFrames or using a mix of indices and columns, Pandas offers the flexibility needed to handle these scenarios efficiently. The left_index
and right_index
parameters, as described in the official Pandas documentation, are instrumental in achieving these merge operations based on index.
Wrapping Up
The merge
function in Pandas stands as a testament to the library's commitment to providing tools that are both powerful and user-friendly. Its ability to seamlessly integrate data from various sources makes it an indispensable tool for anyone working in the data analysis domain. With the various options and parameters it offers, users can tailor their data merging process to fit specific requirements, ensuring that data integrity is maintained while also optimizing for efficiency.
Furthermore, as data continues to play an increasingly critical role in decision-making across industries, the importance of tools like merge
cannot be overstated. Mastering such functions equips professionals with the ability to derive actionable insights from disparate data sources. Whether you're just starting your journey in data science or are a seasoned professional, a deep understanding of Pandas' merging capabilities will undoubtedly enhance your data manipulation and analysis prowess.
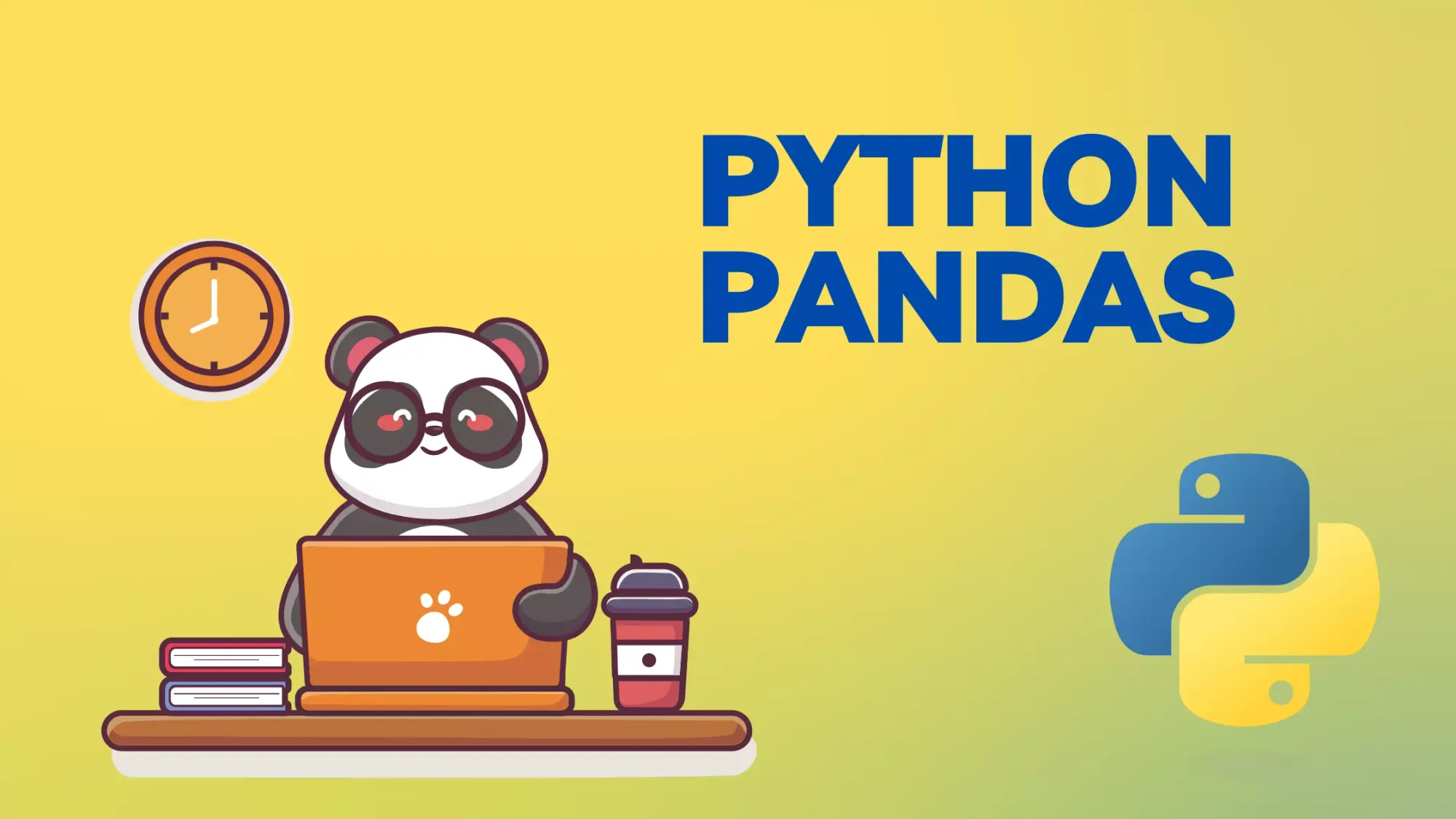