Pandas in Action: A Deep Dive into DataFrame Arithmetics
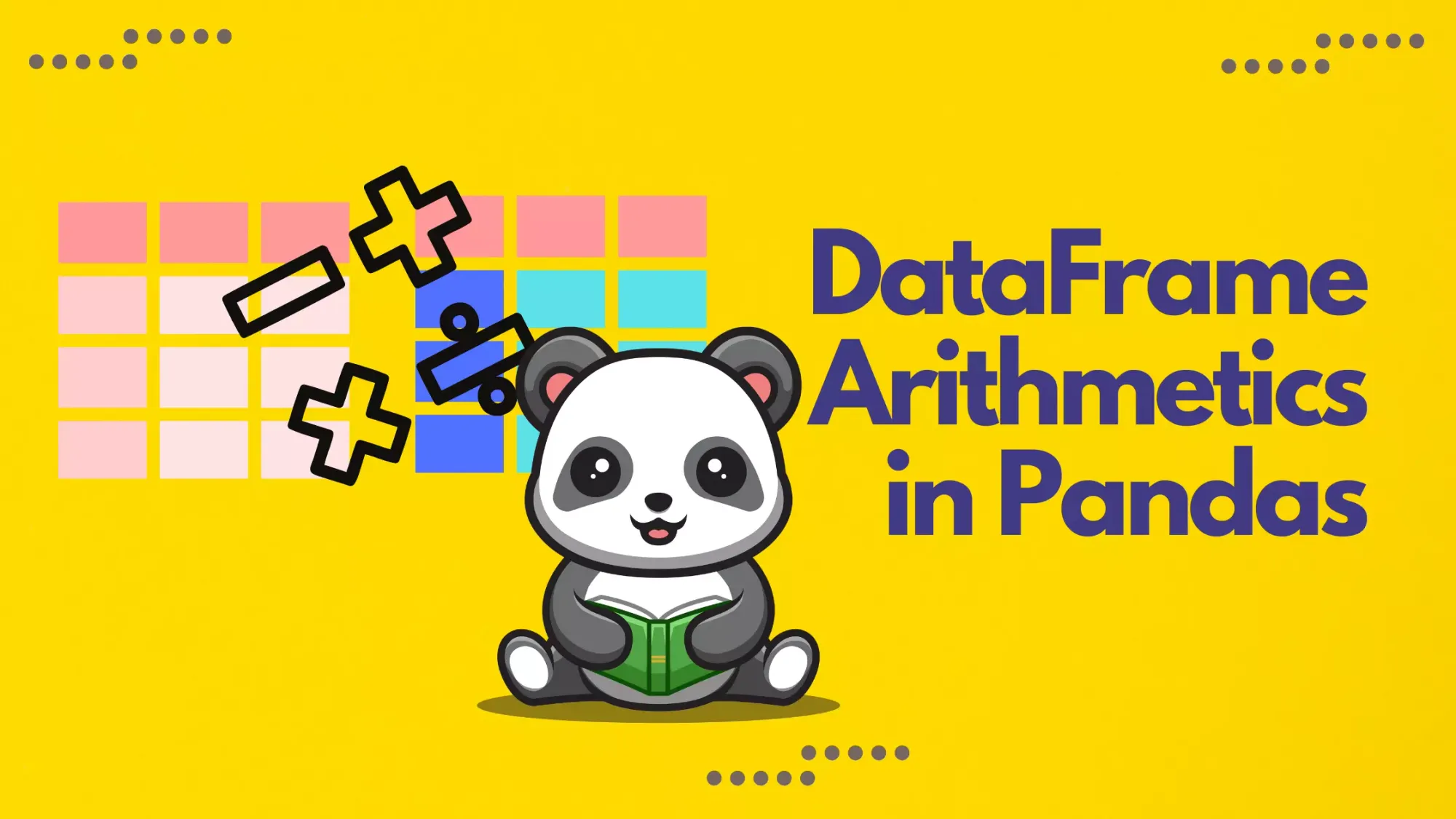
Pandas, the popular Python data analysis library, has become an indispensable tool for data scientists and analysts across the globe. Its robust and flexible data structures, combined with its powerful data manipulation capabilities, make it a go-to solution for diverse data processing needs. One of the foundational objects within Pandas is the DataFrame, a two-dimensional, size-mutable, and potentially heterogeneous tabular data structure with labeled axes (rows and columns).
In this article, we will delve deep into the arithmetic operations you can perform on DataFrames. These operations, ranging from basic addition to advanced broadcasting techniques, play a pivotal role in data transformation and analysis. Accompanied by practical examples, this guide will offer a holistic understanding of DataFrame arithmetics, empowering you to harness the full potential of Pandas in your data endeavors.
Basics of DataFrame Arithmetic
In Pandas, arithmetic operations between DataFrames are element-wise, much like operations with NumPy arrays. When you perform arithmetic between two DataFrames, Pandas aligns them on both row and column labels, which can lead to NaN values if labels are not found in both DataFrames.
Addition (+
)
Addition between two DataFrames will sum up the values for each corresponding element.
Example:
Given the DataFrames:
A | B | |
---|---|---|
0 | 1 | 2 |
1 | 3 | 4 |
A | B | |
---|---|---|
0 | 5 | 6 |
1 | 7 | 8 |
Performing addition will result in:
A | B | |
---|---|---|
0 | 6 | 8 |
1 | 10 | 12 |
- Subtraction (
-
): Subtraction between two DataFrames will subtract the values of the second DataFrame from the first for each corresponding element. - Multiplication (
*
): Multiplication is element-wise, multiplying corresponding elements from two DataFrames. - Division (
/
): Division operates similarly, dividing elements in the first DataFrame by the corresponding elements in the second. - Floor Division (
//
): This operation divides and rounds down to the nearest integer. - Modulus (
%
): Returns the remainder after dividing the elements of the DataFrame by the elements of the second. - Exponentiation (
**
): Raises the elements of the DataFrame to the power of the corresponding elements in the second DataFrame.
Note: For operations that might result in a division by zero, Pandas will handle such cases by returning inf
(infinity).
For more details and nuances, it's always a good idea to refer to the official Pandas documentation on arithmetic operations.
Broadcasting in DataFrames
Broadcasting refers to the ability of NumPy and Pandas to perform arithmetic operations on arrays of different shapes. This can be particularly handy when you want to perform an operation between a DataFrame and a single row or column.
Example:
Given the DataFrame:
A | B | |
---|---|---|
0 | 1 | 2 |
1 | 3 | 4 |
Let's add the series:
A | 5 |
B | 6 |
to the DataFrame above.
The resulting DataFrame after broadcasting addition is:
A | B | |
---|---|---|
0 | 6 | 8 |
1 | 8 | 10 |
Here, each row in the DataFrame df1
was added to the Series s
.
Broadcasting in DataFrames
Broadcasting is a powerful mechanism that allows Pandas to work with arrays of different shapes when performing arithmetic operations. The term originates from NumPy, and Pandas builds on this concept, especially when dealing with DataFrames and Series.
In the context of DataFrames and Series, broadcasting typically involves applying an operation between a DataFrame and a Series. The default behavior is that Pandas aligns the Series index along the DataFrame columns, broadcasting down the rows.
Broadcasting a Series to a DataFrame
Given the DataFrame:
A | B | |
---|---|---|
0 | 1 | 2 |
1 | 3 | 4 |
And the Series:
A | 10 |
B | 20 |
When adding the Series to the DataFrame, each value in the Series will be added to its corresponding column in the DataFrame.
# Series for broadcasting examples
series_broadcast1 = pd.Series({'A': 10, 'B': 20})
series_broadcast_axis = pd.Series([100, 200])
# Performing broadcasting operations
result_broadcast1 = df1 + series_broadcast1
result_broadcast1
A | B | |
---|---|---|
0 | 11 | 22 |
1 | 13 | 24 |
Let's take a slightly different scenario. If the Series does not have the same index as the DataFrame columns, NaN values will be introduced.
Given the same DataFrame and the Series:
A | 10 |
C | 30 |
The result of the addition will contain NaN values for the unmatched column
# Series for broadcasting examples
series_broadcast2 = pd.Series({'A': 10, 'C': 30})
series_broadcast_axis = pd.Series([100, 200])
# Performing broadcasting operations
result_broadcast2 = df1 + series_broadcast2
result_broadcast2
A | B | C | |
---|---|---|---|
0 | 11 | NaN | NaN |
1 | 13 | NaN | NaN |
Broadcasting with axis
Argument
While the default behavior broadcasts across rows, we can also broadcast across columns using the axis
argument.
Given the DataFrame:
A | B | |
---|---|---|
0 | 1 | 2 |
1 | 3 | 4 |
And the Series:
0 | 100 |
1 | 200 |
By subtracting the Series from the DataFrame using axis=0
, each value in the Series will be subtracted from its corresponding row in the DataFrame.
# Series for broadcasting examples
series_broadcast_axis = pd.Series([100, 200])
# Performing broadcasting operations
result_broadcast_axis = df1.sub(series_broadcast_axis, axis=0)
result_broadcast_axis
A | B | |
---|---|---|
0 | -99 | -98 |
1 | -197 | -196 |
These examples highlight the intuitive and flexible nature of broadcasting in Pandas. By understanding how broadcasting works, you can perform a wide range of operations on your data without the need for explicit loops or reshaping. As always, the official Pandas documentation offers a wealth of information for those looking to deepen their understanding.
Arithmetic with Series and DataFrames
Arithmetic between Series and DataFrames in Pandas is closely related to broadcasting mechanics. When you perform an arithmetic operation between a DataFrame and a Series, Pandas aligns the Series index on the DataFrame columns, broadcasting down the rows. If the Series index doesn't match the DataFrame columns, you'll get NaN values.
Row-wise Broadcasting
By default, operations between a DataFrame and a Series match the index of the Series on the columns of the DataFrame and broadcast across the rows.
Example:
Given the DataFrame:
A | B | |
---|---|---|
0 | 1 | 2 |
1 | 3 | 4 |
And the Series:
A | 1 |
B | 2 |
# Creating series for row-wise broadcasting
series_row = pd.Series({'A': 1, 'B': 2})
series_col = pd.Series([1, 2])
# Performing row-wise broadcasting subtraction
result_rowwise = df1 - series_row
result_rowwise
Subtracting the Series from the DataFrame will result in:
A | B | |
---|---|---|
0 | 0 | 0 |
1 | 2 | 2 |
Column-wise Broadcasting
To broadcast over the columns and align the Series index on the rows of the DataFrame, you can use methods like sub
and pass the axis
argument.
Example:
Given the DataFrame:
A | B | |
---|---|---|
0 | 1 | 2 |
1 | 3 | 4 |
And the Series:
0 | 1 |
1 | 2 |
# Creating series for column-wise broadcasting
series_row = pd.Series({'A': 1, 'B': 2})
series_col = pd.Series([1, 2])
# Performing column-wise broadcasting subtraction
result_colwise = df1.sub(series_col, axis=0)
result_colwise
Subtracting the Series from the DataFrame along axis=0
(i.e., column-wise) will result in:
A | B | |
---|---|---|
0 | 0 | 1 |
1 | 1 | 2 |
These examples highlight the flexibility that Pandas offers when it comes to arithmetic operations between Series and DataFrames. By understanding how broadcasting works, and being explicit about the axis when necessary, you can manipulate and transform your data structures with ease and precision. As always, consulting the official Pandas documentation can provide more insights and examples.
Handling Missing Data
Data often comes with missing or null values, and handling them appropriately is crucial for accurate analysis. Pandas provides various tools and methods to detect, remove, or replace these missing values. In the context of arithmetic operations with DataFrames and Series, missing data is represented as NaN
(Not a Number).
When performing arithmetic operations, Pandas ensures that the operations propagate NaN
values, which means that any operation that involves a NaN
will produce a NaN
.
Propagation of NaN in Arithmetic Operations
Given the DataFrames:
A | B | |
---|---|---|
0 | 1 | NaN |
1 | 3 | 4 |
A | B | |
---|---|---|
0 | 5 | 6 |
1 | NaN | 8 |
# Creating dataframes with missing values for examples
df_missing1 = pd.DataFrame({'A': [1, 3], 'B': [float('nan'), 4]})
df_missing2 = pd.DataFrame({'A': [5, float('nan')], 'B': [6, 8]})
# Performing addition operations
result_missing1 = df_missing1 + df_missing2
result_missing1
Performing addition on these DataFrames will propagate the NaN
values:
A | B | |
---|---|---|
0 | 6 | NaN |
1 | NaN | 12 |
Fill Missing Data
While the propagation of NaN
values can be useful, there are instances when you'd want to replace these missing values. The fillna()
function in Pandas is a versatile tool that allows you to replace NaN
values with a scalar value or another data structure like a Series or DataFrame.
For instance, you can replace all NaN
values in a DataFrame with zero using df.fillna(0)
.
These examples underscore the importance of being attentive to missing data when performing arithmetic operations in Pandas. Proper handling of NaN
values ensures the accuracy and integrity of your data analysis. The official Pandas documentation provides a wealth of techniques and best practices for dealing with missing values, ensuring you can navigate and manage such challenges effectively.
Conclusion
Arithmetic operations with Pandas DataFrames provide powerful and flexible tools for data analysis. By mastering the fundamentals of these operations, such as element-wise operations, broadcasting mechanics, and the handling of missing data, analysts can perform complex data manipulations with ease and precision. It's this versatility in handling various arithmetic operations that makes Pandas an indispensable tool in the toolkit of any data professional.
As you continue your journey in data analysis, it's crucial to practice and experiment with these operations to truly internalize their mechanics. Always remember to check the shape and alignment of your DataFrames and Series before performing operations to avoid unintended results. Beyond mere calculations, understanding DataFrame arithmetics is about crafting meaningful narratives from raw data, turning numbers into insights that drive informed decisions.
Happy analyzing!
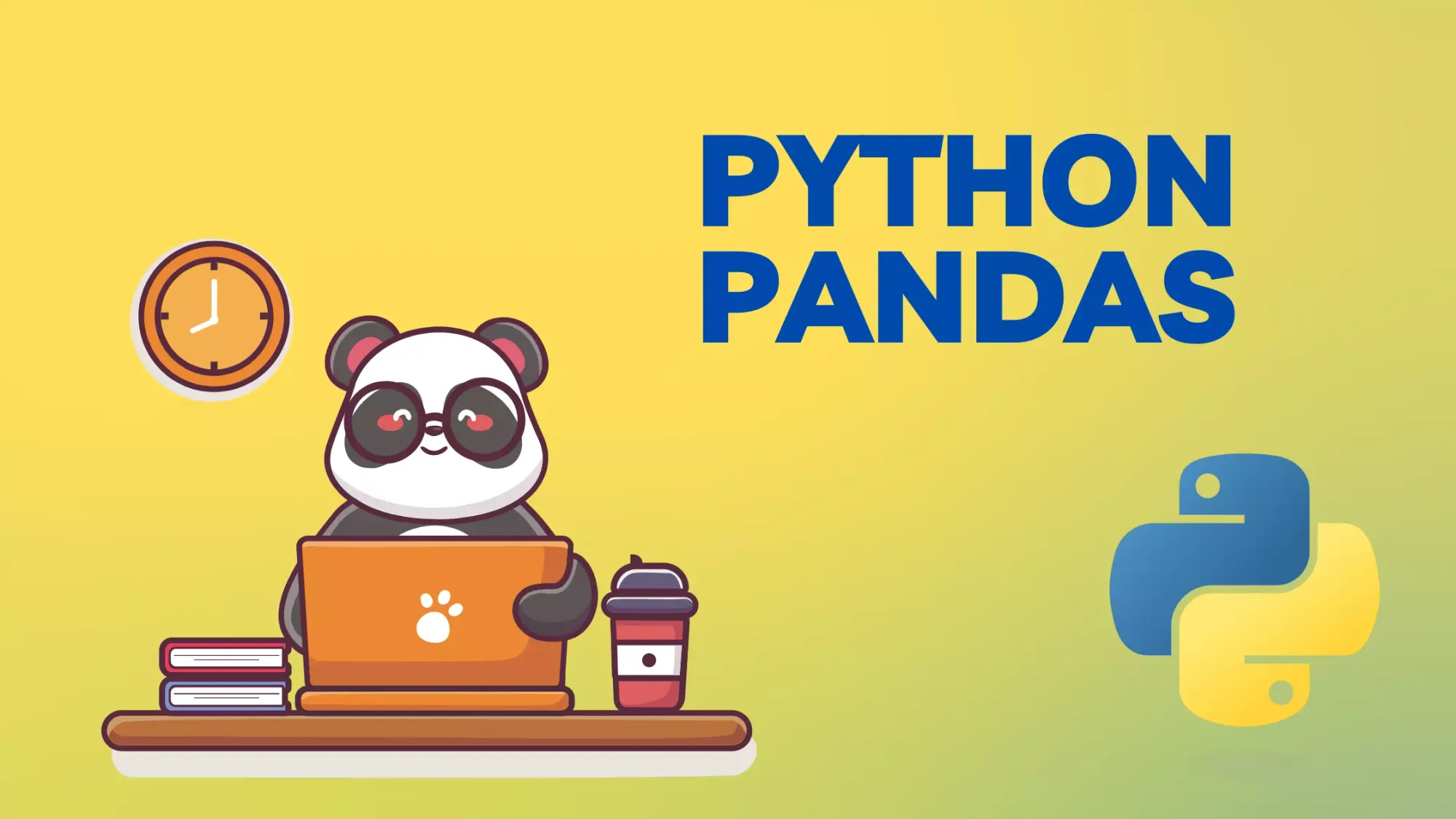