Mastering CSV File Operations with Pandas
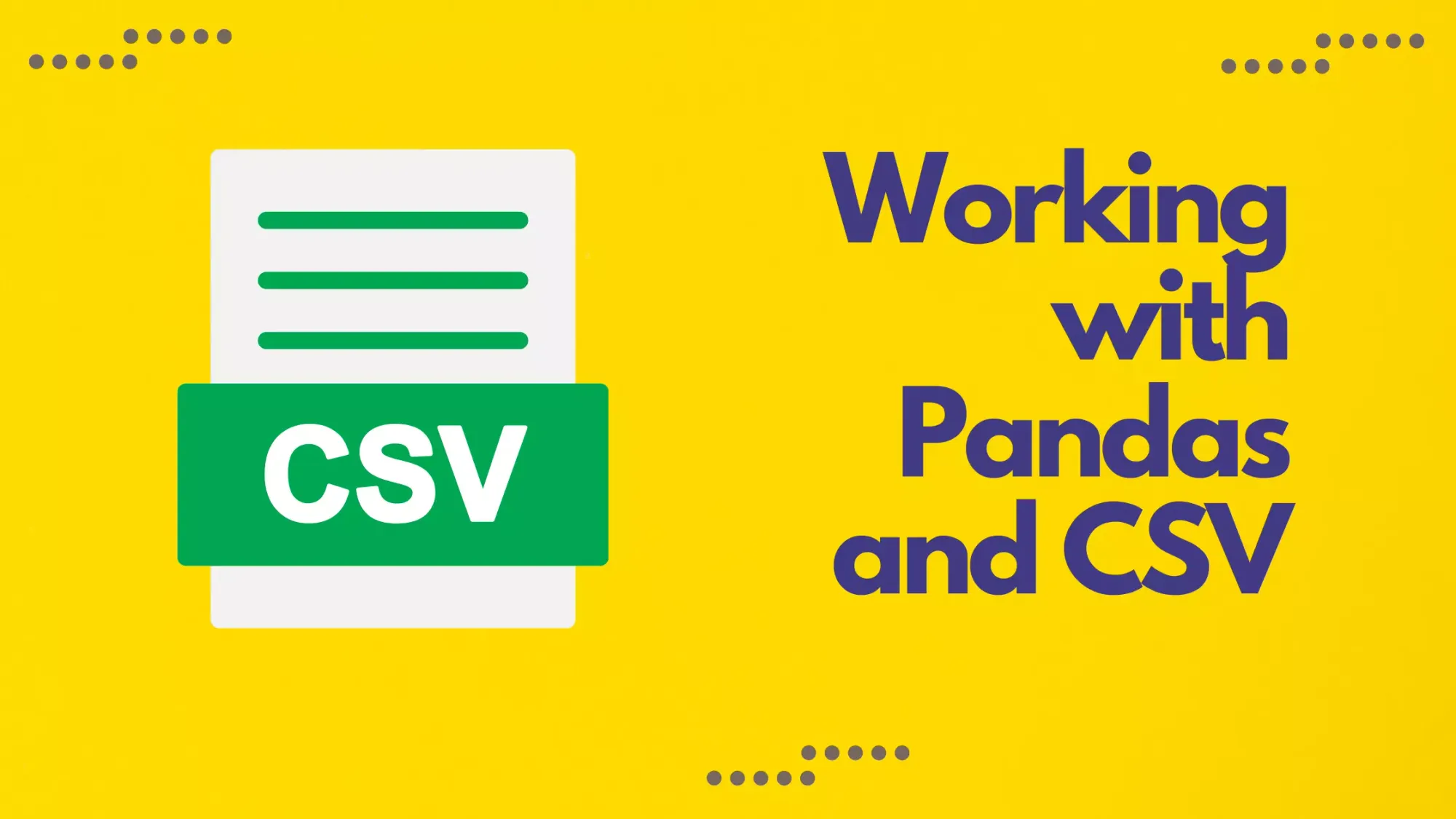
Pandas, short for "Python Data Analysis Library," is one of the most potent and flexible tools available for data manipulation and analysis. At its core, Pandas provides two primary data structures: the Series and the DataFrame. While the Series represents a one-dimensional labeled array, the DataFrame is a two-dimensional labeled data structure with columns that can be of different types, similar to a spreadsheet or SQL table. This versatility makes the DataFrame especially suitable for working with structured data, such as CSV files.
CSV, standing for "Comma-Separated Values," is a simple and widespread format for storing tabular data. It represents data in plain text, with each row corresponding to a line and columns separated by a specific delimiter, commonly a comma. The straightforward nature of CSV makes it a popular choice among data analysts and scientists for data storage and exchange. When combined with the power of Pandas, working with CSV becomes not only efficient but also intuitive, allowing users to focus on data analysis rather than the intricacies of file parsing.
Reading CSV Files
The ability to read CSV files seamlessly is one of the most utilized features of the Pandas library. The function that facilitates this is pd.read_csv()
. With a plethora of parameters, it offers flexibility in handling various CSV structures and intricacies.
Basic CSV Reading
For our most basic demonstration, let's use a small sample CSV data:
name,age,job
Alice,28,Engineer
Bob,32,Doctor
Charlie,24,Artist
At its most basic, you can read a CSV file into a DataFrame just by passing the file's path.
import pandas as pd
df = pd.read_csv('sample.csv')
print(df)
name age job
0 Alice 28 Engineer
1 Bob 32 Doctor
2 Charlie 24 Artist
Specifying Column Names
There might be cases where the CSV file does not contain header information, or you might want to override the existing headers. You can specify column names using the names
parameter and set header=None
if the file doesn't have a header row.
df_no_header = pd.read_csv('no_header.csv', header=None, names=['Name', 'Age', 'Profession'])
print(df_no_header)
For this example, let's use the following sample data without headers:
Daisy,27,Teacher
Edward,35,Lawyer
We'll load this sample, assign column names, and display the result.
Name Age Profession
0 Daisy 27 Teacher
1 Edward 35 Lawyer
Remember, the pd.read_csv()
function offers a myriad of options, making it a versatile tool in your data handling toolkit. When in doubt or looking for more advanced capabilities, the official Pandas documentation is a valuable resource.
Writing DataFrames to CSV
Pandas provides an intuitive method, to_csv()
, for DataFrame objects, allowing users to easily write DataFrames to CSV files. This method is highly customizable with various parameters to fine-tune the output according to specific needs.
Basic DataFrame Writing
The simplest way to write a DataFrame to a CSV file is to call the to_csv()
method and specify the desired output filename.
df.to_csv('output.csv')
For our demonstration, let's use a small DataFrame and write it to a CSV. We'll then read it back to display the result.
After writing our DataFrame to output.csv
and reading it back, we obtained:
Name Age Occupation
0 Anna 24 Engineer
1 Brian 32 Doctor
2 Catherine 29 Artist
Specifying Delimiters and Excluding Headers
CSV, by definition, implies comma-separated values, but you might want to use another delimiter, like a tab or a semicolon. Additionally, there might be instances where you'd want to exclude headers. You can achieve this using the sep
and header
parameters respectively.
df.to_csv('output.tsv', sep='\t', header=False)
We'll write our DataFrame to a TSV (Tab Separated Values) format and exclude the headers. After writing, we'll read it back to show the result.
For the TSV format without headers (output.tsv
), we received:
0 1 2
0 Anna 24 Engineer
1 Brian 32 Doctor
2 Catherine 29 Artist
Note: The numbers 0, 1, and 2 are the default column indices since we excluded headers.
The to_csv()
method is incredibly versatile, allowing for a wide range of customizations in writing DataFrames to various delimited formats. As always, when looking for more advanced capabilities or clarifications, the official Pandas documentation is an invaluable resource.
Handling Different Delimiters
Delimiters play a crucial role in distinguishing between different data points in a file. While the term "CSV" stands for "Comma-Separated Values," in practice, many files use delimiters other than commas. Pandas provides robust support for handling various delimiters, ensuring flexibility in reading and writing data.
Reading with Different Delimiters
Not all files with structured data use the comma as a delimiter. Common alternatives include tabs (TSV for Tab-Separated Values), semicolons, and even spaces.
df_tsv = pd.read_csv('sample.tsv', delimiter='\t')
For our demonstration, let's consider a sample TSV data:
name age job
David 34 Lawyer
Ella 29 Scientist
Frank 45 Architect
We'll load this TSV data and display the result.
Upon loading, we obtained the following DataFrame:
name age job
0 David 34 Lawyer
1 Ella 29 Scientist
2 Frank 45 Architect
Writing with Different Delimiters
When writing a DataFrame to a file, you might also want to use a delimiter other than a comma. This is easily done using the sep
parameter of the to_csv()
method.
df.to_csv('output_with_semicolon.csv', sep=';')
Let's write our DataFrame using a semicolon as a delimiter and then read it back to show the result.
After writing our DataFrame using a semicolon as a delimiter and reading it back, the result was:
name age job
0 David 34 Lawyer
1 Ella 29 Scientist
2 Frank 45 Architect
Pandas' flexibility in handling various delimiters ensures that you can seamlessly integrate with different data sources and formats. This functionality, combined with other features, makes Pandas an indispensable tool in data analysis. For more in-depth information or to explore additional parameters, the official Pandas documentation provides comprehensive insights and explanations.
Dealing with Large CSV Files
When working with very large CSV files, loading the entire dataset into memory might not be feasible or efficient. Pandas provides mechanisms to handle such large datasets effectively without overwhelming system resources.
# Simulating a large CSV with repetitive data for demonstration purposes
large_csv_data = "name,age\n" + "\n".join(["John,28"] * 5000 + ["Doe,45"] * 5000)
# Using StringIO to simulate a file object for the large CSV data
large_csv_file = StringIO(large_csv_data)
Reading in Chunks
Pandas allows you to read large CSV files in smaller chunks, so you can process a part of the dataset at a time, making it memory-efficient.
chunk_size = 1000
chunks = pd.read_csv('large_file.csv', chunksize=chunk_size)
for chunk in chunks:
process(chunk)
For our demonstration, let's consider a simulated large CSV dataset with repetitive data. We'll read it in chunks and display the shape of each chunk to show how the data is partitioned.
For our simulated large CSV dataset, by specifying a chunk size of 2,500 rows, the data was successfully read in four chunks, each containing 2,500 rows and 2 columns:
chunk_shapes = [chunk.shape for chunk in chunks]
[(2500, 2), (2500, 2), (2500, 2), (2500, 2)]
This allows you to process a portion of the dataset at a time, reducing memory consumption and potential processing bottlenecks.
Using dtype
to Optimize Memory Usage
By default, Pandas tries to infer the best datatype for each column, which can sometimes be memory-intensive, especially for large datasets. Specifying the datatype for columns using the dtype
parameter can help reduce memory usage.
optimized_types = {'name': 'category', 'age': 'int32'}
df_large_optimized = pd.read_csv('large_file.csv', dtype=optimized_types)
In our example, we'll use our simulated large CSV dataset again, read it without and with optimized datatypes, and compare the memory usage of both DataFrames.
By comparing the memory usage of the DataFrame read without and with optimized datatypes:
- Memory usage without optimization: 685,128 bytes
- Memory usage with optimization: 50,357 bytes
The memory consumption was significantly reduced by more than 10 times when using optimized datatypes. This optimization is particularly valuable when dealing with very large datasets as it can lead to substantial memory savings.
Handling large CSV files efficiently is essential in real-world data processing tasks. With Pandas' versatile tools, you can navigate the challenges posed by big datasets without compromising performance. For further insights and advanced capabilities, the official Pandas documentation is an excellent guide.
Additional CSV Parameters in Pandas
Pandas offers a rich set of parameters within the read_csv()
function, allowing for fine-tuned control over the CSV reading process. These parameters handle a variety of use-cases and help in dealing with peculiarities that can be encountered in real-world CSV files.
Handling Missing Values
CSV files might have missing or incomplete data. The na_values
parameter lets you specify additional strings to recognize as NaN (Not a Number).
missing_values = ["n/a", "not available"]
df_na = pd.read_csv('data_with_missing.csv', na_values=missing_values)
Consider a sample CSV data:
name,age
Alice,28
Bob,n/a
Charlie,not available
Using the na_values
parameter, we'll treat "n/a" and "not available" as missing values.
name age
0 Alice 28.0
1 Bob NaN
2 Charlie NaN
As seen, the values "n/a" and "not available" are recognized as NaN, indicating missing data.
Parsing Dates
If your CSV contains date columns, they might be read as strings by default. Using the parse_dates
parameter can help in converting specific columns to datetime objects.
df_dates = pd.read_csv('data_with_dates.csv', parse_dates=['birthdate'])
For this example, consider the CSV data:
name,birthdate
Anna,1995-08-15
Brian,1987-04-29
By specifying the parse_dates
parameter, the "birthdate" column will be parsed as a datetime object.
name birthdate
0 Anna 1995-08-15
1 Brian 1987-04-29
The "birthdate" column is now parsed as a datetime object, making date-based operations more straightforward.
Pandas' read_csv()
function is a powerhouse, providing extensive flexibility to cater to diverse data scenarios. These additional parameters ensure that you can fine-tune your data ingestion process, making data cleaning and preprocessing more efficient. For a deeper dive into the available parameters and their specific use-cases, the official Pandas documentation is a comprehensive and invaluable resource.
Conclusions
In the realm of data analysis and manipulation, the synergy between Pandas and CSV files proves to be indispensable. The intuitive functions and methods provided by Pandas simplify the once-tedious tasks of reading, writing, and processing CSV files. By understanding and harnessing the power of Pandas, users can efficiently handle large datasets, specify data types, manage different delimiters, and perform numerous other tasks with ease and precision.
As we move forward in the age of data, the importance of tools that facilitate seamless data operations cannot be overstated. This guide serves as a testament to the capabilities of Pandas when working with CSV files. Whether you're a seasoned data scientist or just starting your journey, mastering the techniques outlined here will undoubtedly elevate your data handling prowess, making your analysis more effective and insightful.
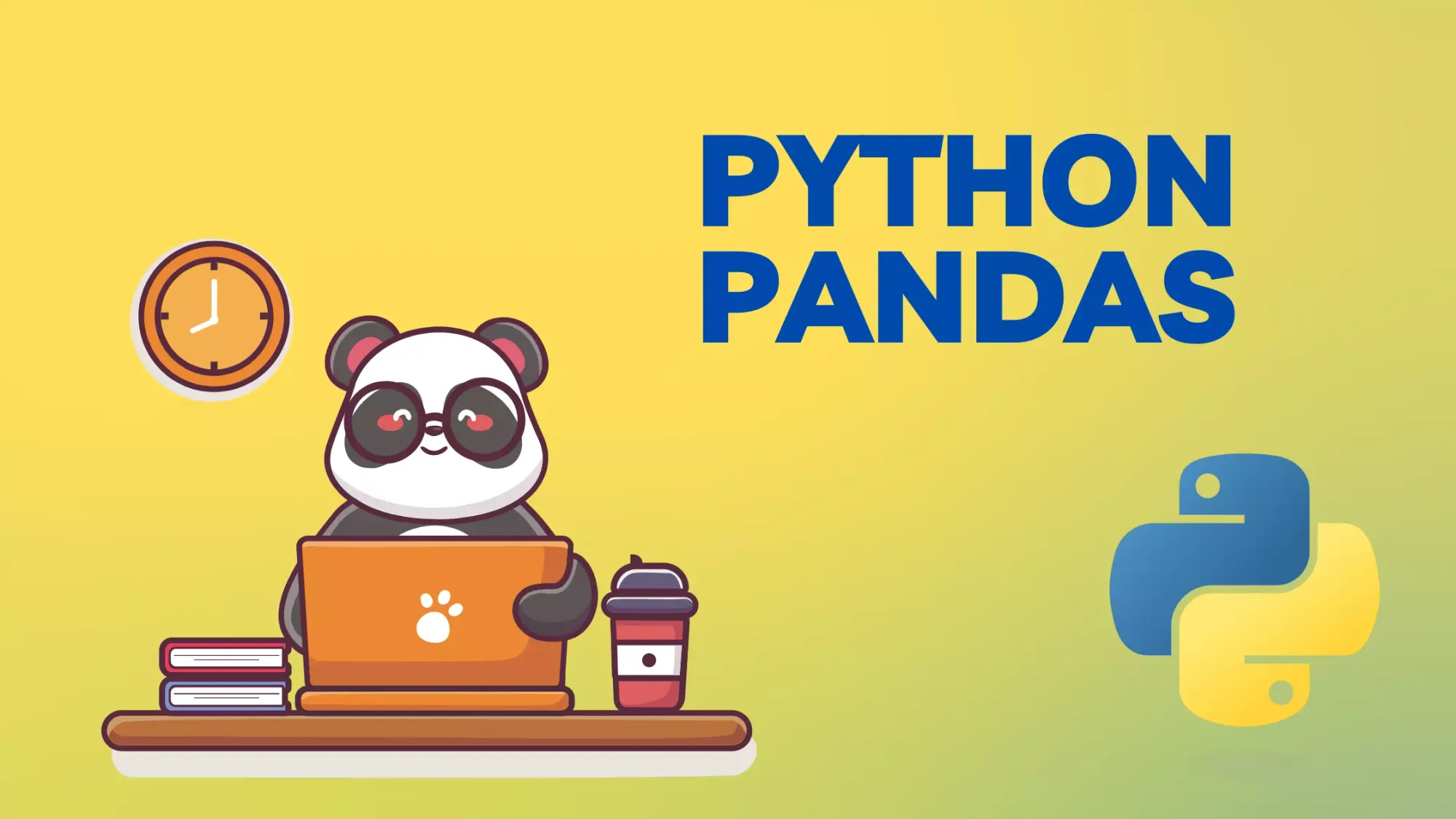