Is Rust Portable?
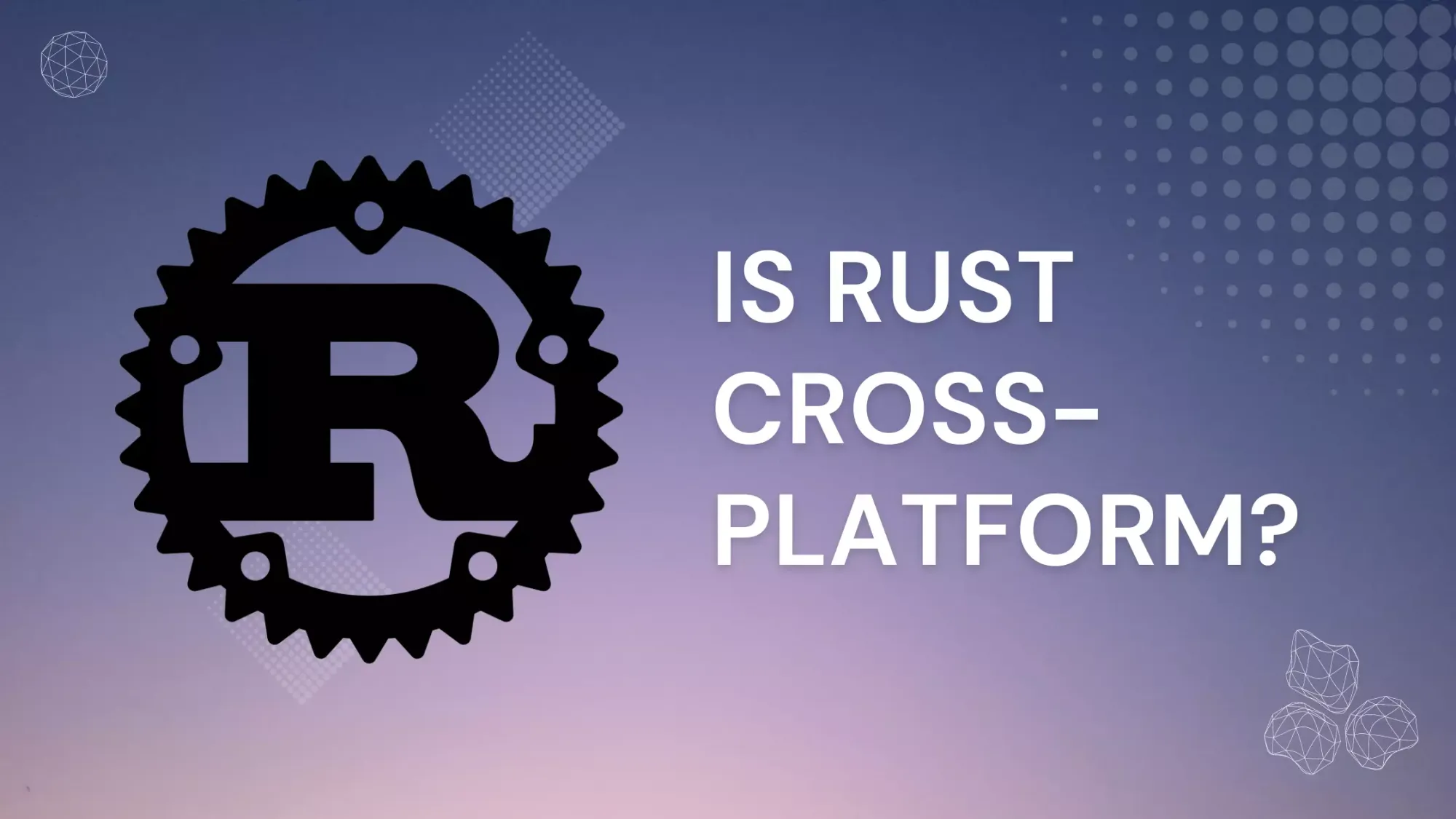
Rust, a programming language that prides itself on safety and performance, has been receiving substantial attention from both hobbyists and professionals. Its memory-safety guarantees and modern syntax make it a favorite among system programmers. But is Rust cross-platform? To answer this question, this article will explore Rust's platform compatibility, including its advantages, challenges, and relevant tools.
Cross-platform development is the practice of writing software that can run on more than one operating system or family of devices. The dream of write-once-run-anywhere has always appealed to developers. Cross-platform languages and tools can reduce development time and costs and make it easier to reach a broader audience.
In this context, Rust's cross-platform capability becomes a compelling subject of discussion.
Rust's Cross-Platform Capability
Architecture Support
Rust's compiler, rustc
, is designed to target a multitude of architectures. Whether it's x86, ARM, MIPS, or even WebAssembly, Rust's standard library is built with support for various platforms. It allows developers to write code that can run on Windows, macOS, Linux, and even some embedded systems without substantial modifications.
The Standard Library
Rust's standard library plays a vital role in its cross-platform capabilities. It abstracts over the underlying operating system's functionality, allowing developers to write code that works across different OSes without having to delve into system-specific details.
Cargo, the Build System
Cargo, Rust's package manager, also plays a role in Rust's cross-platform development story. It manages dependencies, compiles packages, and even assists in cross-compiling, making the task of building and running your Rust code on various platforms easier and more manageable.
Advantages of Using Rust for Cross-Platform Development
Rust has benefits for cross-platform development.
1. Performance
Rust's emphasis on zero-cost abstractions means that you can write high-level code without sacrificing performance. It enables developers to write efficient code that performs similarly across different platforms.
2. Safety
Rust's ownership model eliminates entire classes of bugs related to memory management. This safety guarantee is consistent across platforms, making it a reliable choice for cross-platform development.
3. Ecosystem
The Rust community actively supports cross-platform development, with many libraries and tools specifically designed to facilitate writing platform-independent code.
Challenges and Considerations
There are still various limitations in Rust portability that need to be taken into account before starting to develop truly cross-platform software.
1. GUI Development
While Rust provides robust support for core logic, GUI development is still an area where the language faces challenges. The lack of a unified, native GUI library makes writing cross-platform GUI applications more complex.
2. Platform-Specific Code
Although Rust's standard library abstracts many details, platform-specific requirements may still necessitate writing custom code for different operating systems.
Tools for Cross-Platform Development
Several tools and libraries are designed to aid in Rust's cross-platform development:
- Cross: A tool that simplifies the task of cross-compiling Rust projects.
- GTK, Qt, and other GUI libraries: Several bindings to popular GUI libraries facilitate cross-platform GUI development.
How to handle platform-specific code?
Handling platform-specific code in Rust is a common task, especially when dealing with cross-platform development. Fortunately, Rust provides mechanisms to achieve this in a clean and maintainable way. Here's how:
1. Conditional Compilation
Rust supports conditional compilation using attributes like cfg
. This allows you to include or exclude portions of code based on specific platform criteria.
Example:
#[cfg(target_os = "windows")]
fn platform_specific_function() {
println!("Running on Windows!");
}
#[cfg(target_os = "linux")]
fn platform_specific_function() {
println!("Running on Linux!");
}
fn main() {
platform_specific_function(); // Calls the appropriate function based on the target OS
}
2. Separate Platform Modules
You can also separate platform-specific code into different modules and include them conditionally. This helps keep your code organized and easy to maintain.
Example:
Create separate files like windows.rs
, linux.rs
, etc., and then conditionally include them in your main code.
// main.rs
#[cfg(target_os = "windows")]
mod windows;
#[cfg(target_os = "linux")]
mod linux;
fn main() {
#[cfg(target_os = "windows")]
windows::platform_specific_function();
#[cfg(target_os = "linux")]
linux::platform_specific_function();
}
3. Using Crates that Abstract Platform Differences
Sometimes, the Rust community may have already created crates (libraries) that abstract away platform-specific differences. Utilizing these crates can save time and effort.
For instance, if you need to deal with file paths across different platforms, you might use the std::path::Path
module from Rust's standard library, which handles platform-specific differences automatically.
4. Leveraging build.rs
Rust's build system allows you to write custom build logic in a file named build.rs
. You can use this file to detect the target platform and configure your build accordingly. This might include setting certain compiler flags or including/excluding specific files or dependencies based on the platform.
5. Fallbacks and Defaults
In some cases, you may want to provide a default implementation that works across platforms but can be overridden by platform-specific code. You can achieve this by combining the cfg
attribute with the all
and not
logic.
Example:
#[cfg(all(target_os = "linux", feature = "special_feature"))]
fn special_function() {
// Linux-specific implementation with special feature
}
#[cfg(not(all(target_os = "linux", feature = "special_feature")))]
fn special_function() {
// Default implementation for other platforms or when the special feature is disabled
}
Rust provides a powerful and flexible system for managing platform-specific code through conditional compilation, modular organization, community crates, custom build logic, and logical fallbacks. By leveraging these features, you can write cross-platform code that's efficient, maintainable, and clear.
Conclusion
Rust is indeed a cross-platform language. Its modern syntax, memory safety, and robust standard library make it suitable for writing software that runs on multiple operating systems. While there are still challenges in specific areas like GUI development, the active Rust community and the ever-growing ecosystem of tools and libraries make Rust a compelling choice for cross-platform projects.
For educators, developers, and engineers looking to delve into a modern programming paradigm without sacrificing performance and broad applicability, Rust offers an exciting pathway. Its philosophy and design are continually pushing the boundaries of what is possible in cross-platform programming, promising a future where the dream of write-once-run-anywhere becomes an everyday reality.