Flipping Data: A Guide to Transposing in R
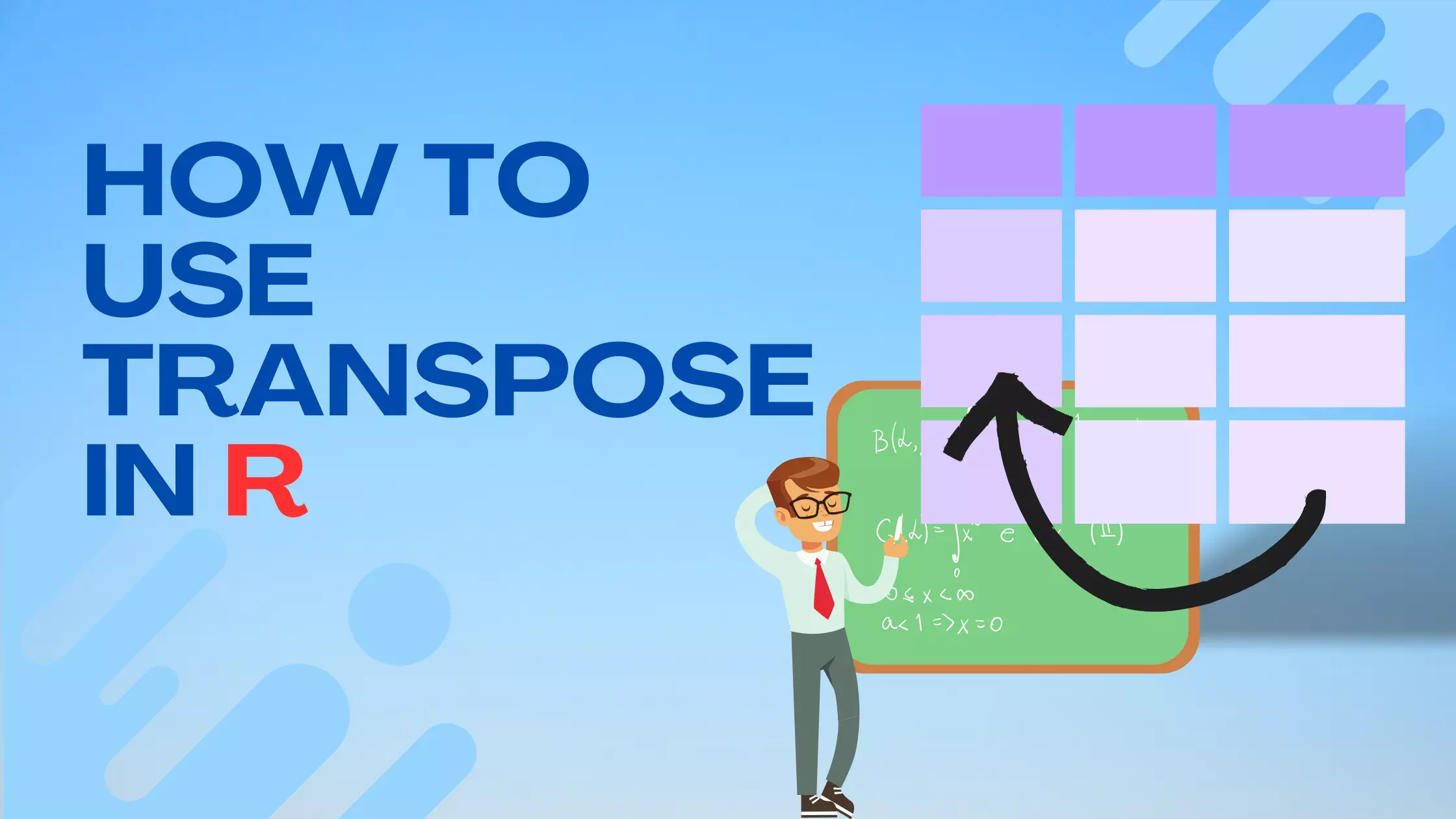
R is a versatile programming language widely used for statistical computing, data analysis, and graphics. Developed by statisticians, R offers a comprehensive range of statistical and graphical techniques. Its rich ecosystem, which includes numerous packages and libraries, ensures that R meets the needs of diverse data operations.
One such operation, fundamental to data manipulation and transformation, is the transposition of a matrix or data frame. Transposing data can often unveil hidden patterns and is a common requirement for various analytical algorithms. In this article, we'll provide a deep dive into the mechanics of using the transpose function in R, exploring a variety of techniques ranging from basic applications to more advanced methods, all complemented by hands-on examples.
What is Transposition?
Transposition is a fundamental operation performed on matrices and data frames. At its core, transposition involves flipping a matrix over its diagonal, which results in the interchange of its rows and columns. This seemingly simple operation is crucial in various mathematical computations, especially in linear algebra where it's used in operations like matrix multiplication, inversion, and finding determinants.
To visualize, consider a matrix:
1 | 2 | 3 |
4 | 5 | 6 |
When transposed, it becomes:
1 | 4 |
2 | 5 |
3 | 6 |
The main diagonal, which starts from the top left and goes to the bottom right, remains unchanged. All other elements are mirrored across this diagonal.
Beyond the mathematical perspective, transposition has practical significance in data analysis. For example, in time series data, where rows could represent dates and columns could represent metrics, transposing can help in comparing metrics across different dates. Similarly, in data visualization, transposing data can aid in switching the axes of a plot to provide a different perspective or to better fit a specific visualization technique.
Transposition is not just a mathematical operation but a powerful tool that aids in reshaping data, making it more suitable for various analyses, visualizations, and computations. Understanding the intricacies of transposition can greatly enhance one's ability to manipulate and interpret data effectively.
Basic Transposition in R
In R, the process of transposing is straightforward but extremely powerful. The core function for this operation is t()
. This function is primarily designed for matrices, but it also works seamlessly with data frames. When used, the t()
function effectively switches rows with columns, resulting in the transposed version of the given matrix or data frame.
Example 1: Transposing a Matrix
Let's start with a basic matrix:
mat <- matrix(1:6, nrow=2)
print(mat)
This matrix looks like:
1 | 3 | 5 |
2 | 4 | 6 |
Now, applying the t()
function:
t_mat <- t(mat)
print(t_mat)
The transposed matrix is:
1 | 2 |
3 | 4 |
5 | 6 |
Example 2: Transposing a Data Frame
Data frames can also be transposed in a similar fashion. Consider the following data frame:
df <- data.frame(Name = c("Alice", "Bob"), Age = c(25, 30), Score = c(85, 90))
print(df)
This data frame appears as:
Name | Age | Score |
---|---|---|
Alice | 25 | 85 |
Bob | 30 | 90 |
Upon transposition:
t_df <- as.data.frame(t(df))
print(t_df)
The transposed data frame will be:
V1 | V2 | |
---|---|---|
Name | Alice | Bob |
Age | 25 | 30 |
Score | 85 | 90 |
Note: When transposing a data frame, it's often necessary to convert the result back into a data frame using as.data.frame()
since the t()
function will return a matrix.
For an in-depth look at the t()
function, its applications, and other related details, one can refer to the official R documentation. This documentation provides a thorough overview, touching on various aspects of the function and its usage scenarios.
Advanced Techniques
While the basic t()
function provides an easy and efficient way to transpose matrices and data frames in R, there are scenarios where more advanced techniques become necessary. Especially when dealing with large datasets, complex data structures, or specific reshaping needs, R offers a plethora of advanced methods to facilitate transposition. These techniques not only optimize performance but also offer greater flexibility in manipulating data structures. In this section, we will delve into these advanced transposition methods, exploring their intricacies and showcasing their prowess through hands-on examples.
Transposing with data.table
Package
The data.table
package in R is a high-performance version of data.frame
, particularly designed for larger datasets. It offers a variety of functionalities optimized for faster data manipulation and aggregation. One of the features it provides is a more efficient transposition method, especially useful when working with extensive data.
To utilize the data.table
package for transposition, one would typically use the transpose()
function it offers. This function is designed to quickly switch rows with columns, making it a valuable tool when dealing with larger datasets.
Example: Transposing a Data Table
To start, you'd first need to install and load the data.table
package:
install.packages("data.table")
library(data.table)
Let's create a sample data table:
dt <- data.table(Name = c("Alice", "Bob", "Charlie"), Age = c(25, 30, 28), Score = c(85, 90, 88))
print(dt)
This data table appears as:
Name | Age | Score |
---|---|---|
Alice | 25 | 85 |
Bob | 30 | 90 |
Charlie | 28 | 88 |
Now, let's transpose it using the transpose()
function:
transposed_dt <- transpose(dt)
print(transposed_dt)
The transposed data table will be:
V1 | V2 | V3 | |
---|---|---|---|
Name | Alice | Bob | Charlie |
Age | 25 | 30 | 28 |
Score | 85 | 90 | 88 |
Note: The column names (V1, V2, V3, etc.) are automatically assigned during the transposition. Depending on your needs, you might want to rename them for clarity.
For those interested in diving deeper into the functionalities provided by the data.table
package, including its transposition capabilities, the official data.table
documentation serves as a comprehensive resource. This documentation covers a broad spectrum of topics, ensuring users can harness the full potential of the package in their data operations.
Transposing a Subset of Columns
At times, in data analysis and manipulation, there's a need to transpose only a specific subset of columns rather than the entire dataset. R, with its versatile functions, allows users to easily subset and transpose specific columns from matrices and data frames.
Example: Transposing Selected Columns from a Data Frame
Consider a data frame that contains information about students' scores in different subjects:
df <- data.frame(Name = c("Alice", "Bob", "Charlie"), Math = c(85, 78, 90), Physics = c(88, 80, 86), Chemistry = c(80, 89, 92))
print(df)
This data frame appears as:
Name | Age | Score |
---|---|---|
Alice | 25 | 85 |
Bob | 30 | 90 |
Charlie | 28 | 88 |
Suppose we're only interested in transposing the scores for "Math" and "Physics". We can achieve this by subsetting these columns and then using the t()
function:
subset_df <- df[, c("Math", "Physics")]
transposed_subset <- t(subset_df)
print(transposed_subset)
The transposed result will be:
V1 | V2 | V3 | |
---|---|---|---|
Name | Alice | Bob | Charlie |
Age | 25 | 30 | 28 |
Score | 85 | 90 | 88 |
The ability to subset columns in R is fundamental and is extensively discussed in the official R documentation for data extraction.
Alternative Methods
While the conventional tools in R offer robust solutions for transposition, it's often beneficial to explore alternative techniques that can provide unique advantages or cater to niche requirements. These alternative methods, stemming from various packages or innovative uses of base R functions, can sometimes offer more efficient, intuitive, or flexible ways to transpose data. In this section, we will journey through some of these lesser-known yet powerful approaches, broadening our toolkit for data transposition in R.
Using apply
Function
The apply
function in R is a versatile tool primarily used for applying a function to the rows or columns of a matrix (and, to some extent, data frames). Its flexibility makes it a handy alternative for transposing data, especially when you want to maintain data types or apply additional transformations during the transposition process.
Example: Transposing a Matrix with apply
Consider the following matrix:
mat <- matrix(c(1, 2, 3, 4, 5, 6), ncol=3)
print(mat)
This matrix appears as:
1 | 3 | 5 |
2 | 4 | 6 |
To transpose this matrix using the apply
function:
transposed_mat <- apply(mat, 2, as.vector)
print(transposed_mat)
The transposed result will be:
1 | 2 |
3 | 4 |
5 | 6 |
Here, the apply
function is set to operate on the matrix's columns (the '2' argument indicates this) and then converts each column into a vector using as.vector
, effectively transposing the matrix.
The apply
function is a core part of R's base package, making it a tool every R programmer should be familiar with. For a comprehensive understanding of its parameters, applications, and nuances, the official R documentation on apply
serves as an invaluable resource. This documentation sheds light on its diverse capabilities, from basic data transformations to more complex operations.
Using tidyr
Package
The tidyr
package is a member of the tidyverse
family in R, a collection of packages designed for data science and data manipulation. While tidyr
primarily focuses on reshaping and tidying data, some of its functions can be employed in a way that effectively transposes data, especially when moving from a 'wide' format to a 'long' format or vice versa.
Example: Pivoting Data with tidyr
Imagine a data frame that captures the sales of two products over three months:
library(tidyr)
df <- data.frame(Month = c("Jan", "Feb", "Mar"), ProductA = c(100, 110, 105), ProductB = c(90, 95, 92))
print(df)
This data frame looks like:
Month | ProductA | ProductB |
---|---|---|
Jan | 100 | 90 |
Feb | 110 | 95 |
Mar | 105 | 92 |
Now, let's transpose this data to see the sales by product across months. We can use the pivot_longer
function from tidyr
:
transposed_df <- df %>% pivot_longer(cols = c(ProductA, ProductB), names_to = "Product", values_to = "Sales")
print(transposed_df)
The transposed data frame will be:
Month | Product | Sales |
---|---|---|
Jan | ProductA | 100 |
Jan | ProductB | 90 |
Feb | ProductA | 110 |
Feb | ProductB | 95 |
Mar | ProductA | 105 |
Mar | ProductB | 92 |
Here, we've transformed the data to a 'long' format where each row represents sales for a product in a particular month.
The tidyr
package is a cornerstone in the tidyverse
collection, and its data reshaping capabilities are vast. For those eager to explore its full range of functions, intricacies, and potential applications, the official tidyr documentation serves as a comprehensive guide. This resource delves into the details of tidying data, providing users with a deep understanding of the package's capabilities and applications.
Performance and Best Practices in Data Transposition in R
Transposing data is a common operation in R, especially when dealing with datasets in statistical analyses, data visualization, or machine learning. But as with any operation, especially in a data-rich environment, it's essential to consider performance and adhere to best practices. Here's a guide to ensuring efficient and effective transposition in R:
1. Consider Data Size:
- Memory Usage: Transposing large datasets can be memory-intensive. Before transposing, ensure that your system has enough memory to handle the transposed data.
- Efficiency: Some methods are more efficient for large datasets. For instance, the
data.table
package can transpose data faster than the base R functions for bigger datasets.
2. Preserve Data Integrity:
- Data Types: Ensure that the transposition method retains the data types of variables. Some methods might convert factors to characters or integers to doubles.
- Column Names: When transposing, column names often become row names. Ensure that essential metadata is not lost in the process.
3. Use Appropriate Methods:
- For Matrices: If you're working with matrices, use the
t()
function or theapply()
function, which are optimized for matrix operations. - For Data Frames: For data frames, consider using
tidyr
ordata.table
, especially if you also need to reshape the data.
4. Avoid Unnecessary Transposition:
- Transpose data only when necessary. Sometimes, the objective can be achieved without actually changing the data structure.
5. Benchmarking:
- If unsure about which method to use, especially for large datasets, benchmark different methods using the
microbenchmark
package. This will give you insights into the speed of various methods and help you make an informed choice.
6. Test with Subsets:
- Before transposing a large dataset, test the transposition method on a subset of the data. This will help you catch potential issues without having to wait for a long computation.
R's comprehensive documentation and the CRAN repository are invaluable resources. They provide insights into the latest updates, optimized functions, and best practices, ensuring that you are always working with the most efficient and reliable tools at your disposal.
Conclusion
Transposing data is more than just a routine operation; it's an essential tool in a data scientist's or statistician's arsenal, allowing for more effective data analysis, visualization, and preparation for machine learning algorithms. Whether you're pivoting data for a report or pre-processing data for a neural network, understanding how to transpose efficiently can streamline your workflow and potentially unveil insights that might remain hidden in a traditional data layout.
In this guide, we've explored the myriad ways R facilitates transposition, from its in-built functions to powerful packages tailor-made for extensive data operations. With R's flexible environment and the techniques covered in this article, you're well-equipped to handle any transposition challenge that comes your way, ensuring your data is always primed for the insights you seek.
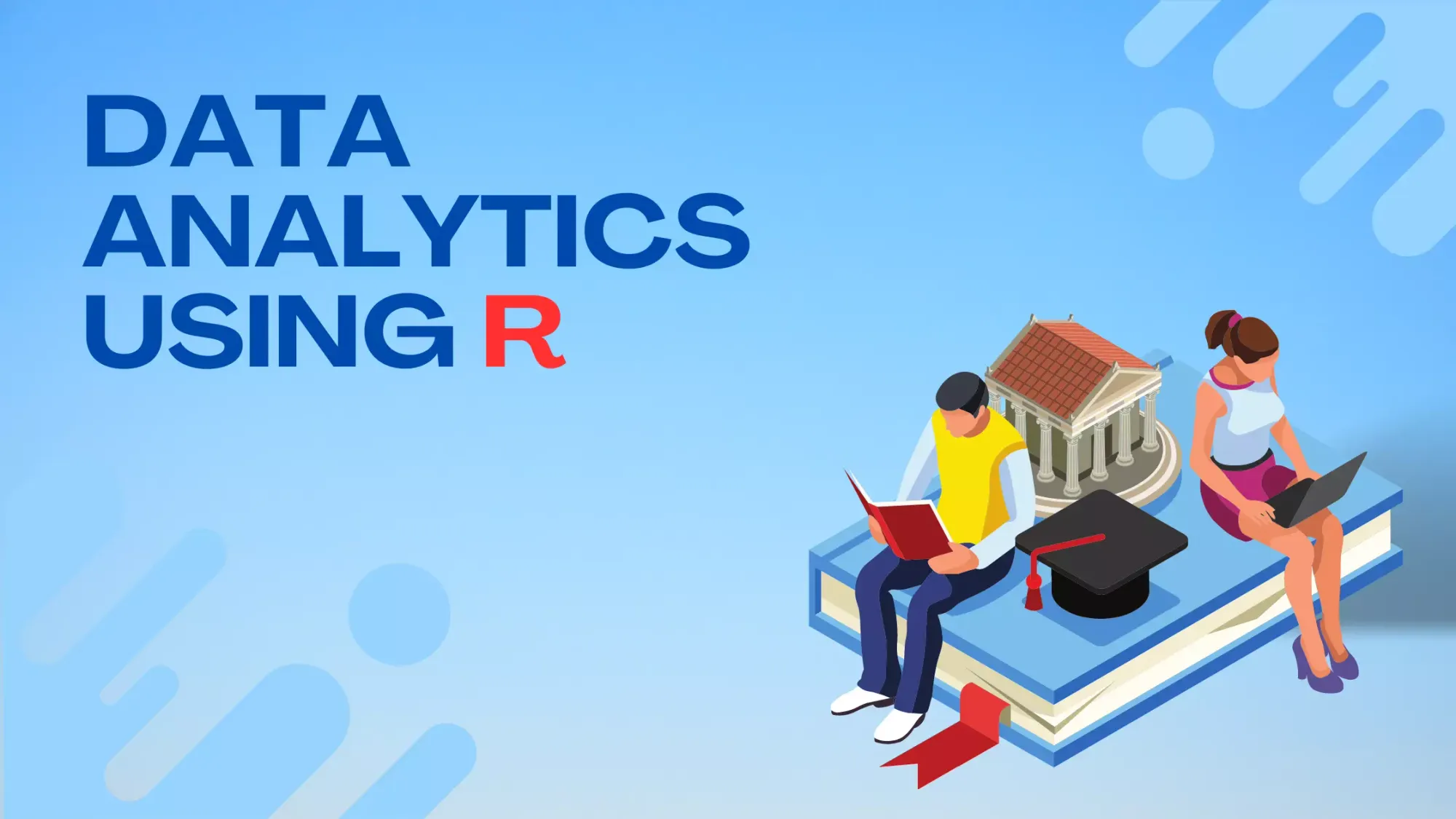