Mastering Column Renaming in Pandas: A Step-by-Step Guide
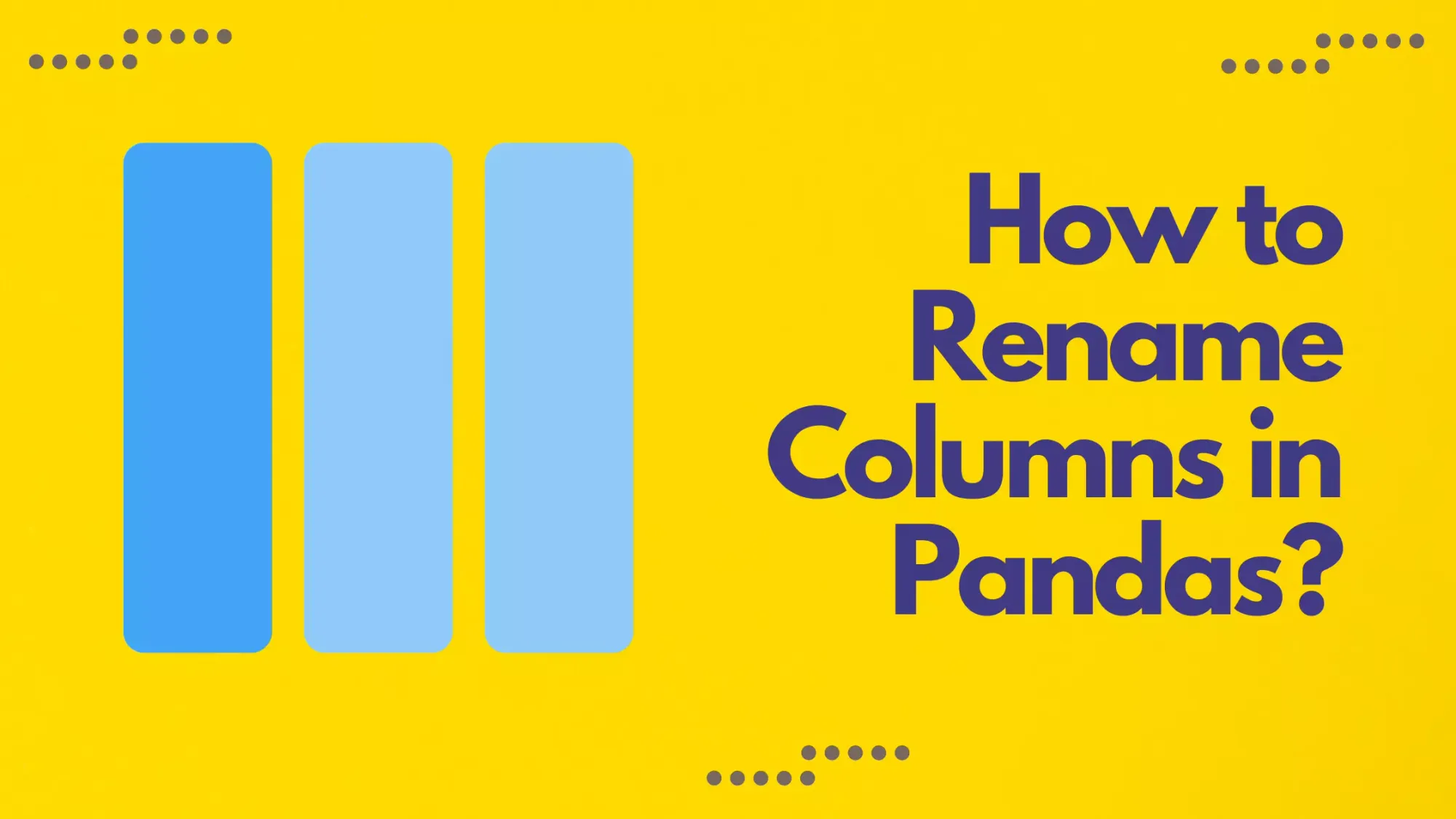
Pandas, an essential library in the Python ecosystem, has revolutionized the way data scientists and analysts handle and process data. This powerful tool provides an array of functionalities that enable seamless manipulation, transformation, and analysis of structured datasets.
Among the myriad of operations that Pandas offers, renaming DataFrame columns stands out as a fundamental yet crucial task. Whether you're tidying up your dataset for better readability, preparing it for integration with another dataset, or simply making it more interpretable for presentation, understanding the nuances of renaming columns is indispensable. In this guide, we'll delve into 4 different methods to rename columns in a pandas DataFrame, ensuring that you have the skills necessary to manage your data with finesse and precision.
Brief introduction to Pandas DataFrame
Pandas, often described as the "Swiss Army knife" for data manipulation in Python, is built upon the foundation of two primary data structures: the Series
and the DataFrame
. While a Series
represents a one-dimensional labeled array, a DataFrame
is a two-dimensional, size-mutable, and heterogeneous tabular data structure. Essentially, a DataFrame
can be visualized as a table with rows and columns, where each column can contain data of a different type (e.g., numeric, string, boolean, etc.).
The beauty of DataFrames lies in their versatility. They allow for a variety of operations, including but not limited to data indexing, slicing, aggregation, and pivoting. This makes them especially useful for tasks ranging from simple data exploration to more complex data transformation and analysis. The underlying structure of a DataFrame is designed to handle large datasets efficiently, making pandas a preferred choice for data analysis in Python.
One of the primary sources of the DataFrame's power is its ability to read from and write to a multitude of data sources, including CSV files, Excel spreadsheets, SQL databases, and many more. Furthermore, its integration with other Python libraries like NumPy, Matplotlib, and Scikit-learn makes the transition between data preprocessing and other tasks like visualization or machine learning seamless.
For a more in-depth understanding and exploration of DataFrames and their functionalities, the official pandas documentation serves as an invaluable resource. It offers detailed explanations, examples, and best practices that can enhance one's proficiency in handling data with pandas.
import pandas as pd
data = {
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
}
df = pd.DataFrame(data)
print(df)
1. Basic Renaming with the rename
Method
The rename
method in pandas is a versatile function designed to allow users to alter axis labels, which includes changing column and row names. Its primary strength lies in the fact that you don't need to rename all columns or rows; you can selectively choose which ones to modify.
Syntax:
DataFrame.rename(mapper=None, index=None, columns=None, axis=None, copy=True, inplace=False, level=None, errors='raise')
Here, the relevant parameters for our discussion are:
mapper
,index
, orcolumns
: These are alternative ways to specify the renaming logic. Typically, you would use a dictionary forcolumns
where the keys are the old names and the values are the new names.inplace
: A boolean that decides whether to return a new DataFrame (False) or modify the existing one (True).axis
: Set it to 1 or 'columns' to rename columns. By default, it's set to 0 or 'index', which would rename rows.
For a complete list of parameters and further details, one should refer to the official pandas documentation on the rename
method.
Renaming Specific Columns:
Suppose you have a dataset with columns named 'Age', 'Name', and 'Country'. You want to change 'Age' to 'Years' and 'Country' to 'Nation'.
df_example1 = pd.DataFrame({
'Age': [25, 30, 35],
'Name': ['Alice', 'Bob', 'Charlie'],
'Country': ['USA', 'UK', 'Canada']
})
df_renamed_example1 = df_example1.rename(columns={'Age': 'Years', 'Country': 'Nation'})
Original DataFrame:
Age Name Country
0 25 Alice USA
1 30 Bob UK
2 35 Charlie Canada
After Renaming:
Years Name Nation
0 25 Alice USA
1 30 Bob UK
2 35 Charlie Canada
Using the axis
Parameter:
In this example, we'll rename the 'Name' column to 'First Name', using the axis
parameter.
df_example2 = pd.DataFrame({
'Name': ['David', 'Eva', 'Frank'],
'Score': [85, 90, 78]
})
df_renamed_example2 = df_example2.rename({'Name': 'First Name'}, axis=1)
Original DataFrame:
Name Score
0 David 85
1 Eva 90
2 Frank 78
After Renaming:
First Name Score
0 David 85
1 Eva 90
2 Frank 78
The rename
method is truly versatile and essential for dataframe manipulation. By referring to the official pandas documentation on the rename
method, you can explore even more use cases and nuances of this function.
2. Renaming All Columns
In many scenarios, you might want to rename all columns of a DataFrame to maintain consistency, improve readability, or prepare the dataset for integration with another DataFrame. Pandas provides an incredibly straightforward way to achieve this: by reassigning the columns
attribute of the DataFrame.
Syntax:
DataFrame.columns = ['new_col_name1', 'new_col_name2', ...]
While this method is direct, it's crucial to remember that the list of new column names should match the number of columns in the DataFrame. Otherwise, a ValueError
will be raised.
For further exploration on DataFrame attributes, the official pandas documentation is an excellent resource.
Renaming Columns for Consistency:
Imagine a dataset with columns named in an inconsistent manner: 'ID_', 'First_name', and 'last-name'. For consistency, you might want to rename them to 'ID', 'First Name', and 'Last Name'.
df_example3 = pd.DataFrame({
'ID_': [101, 102, 103],
'First_name': ['Greg', 'Hannah', 'Ian'],
'last-name': ['Jones', 'Smith', 'Doe']
})
df_example3.columns = ['ID', 'First Name', 'Last Name']
Original DataFrame:
ID_ First_name last-name
0 101 Greg Jones
1 102 Hannah Smith
2 103 Ian Doe
After Renaming:
ID First Name Last Name
0 101 Greg Jones
1 102 Hannah Smith
2 103 Ian Doe
Adapting to a New Data Standard:
Consider a DataFrame with columns 'Name', 'Age', and 'Country'. For integration purposes, you need to change them to 'Full Name', 'Years Old', and 'Nation'.
df_example4 = pd.DataFrame({
'Name': ['Julia', 'Kevin', 'Liam'],
'Age': [29, 32, 27],
'Country': ['Germany', 'Australia', 'Ireland']
})
df_example4.columns = ['Full Name', 'Years Old', 'Nation']
Original DataFrame:
Name Age Country
0 Julia 29 Germany
1 Kevin 32 Australia
2 Liam 27 Ireland
After Renaming:
Full Name Years Old Nation
0 Julia 29 Germany
1 Kevin 32 Australia
2 Liam 27 Ireland
Directly reassigning the columns
attribute is a straightforward approach for renaming all columns in a DataFrame. This method is both concise and effective, but care should be taken to ensure the new column names list matches the DataFrame's column count. For more intricate renaming needs or when renaming only specific columns, other methods such as the rename
function might be more appropriate. As always, the official pandas documentation provides extensive details on DataFrame attributes and functionalities.
3. Using set_axis
for Renaming
The set_axis
method in pandas is a lesser-known but highly versatile method to rename either the rows or the columns of a DataFrame. While the rename
method is often the go-to for many users, set_axis
can be especially useful when renaming all labels on a specific axis in one go.
Syntax:
DataFrame.set_axis(labels, axis=0, inplace=False)
Relevant parameters for our discussion:
labels
: The list of labels to set, must be of the same length as the current labels.axis
: The axis to set the labels for. Can be either 0/'index' (default) or 1/'columns'.inplace
: If set to True, it modifies the original DataFrame in-place. By default, it returns a new DataFrame.
For a more detailed explanation of the parameters and their possible values, one should refer to the official pandas documentation on the set_axis
method.
Renaming All Columns:
Suppose you have a dataset with columns 'id', 'first', and 'country'. You'd like to rename them to 'ID', 'First Name', and 'Country'.
df_example5 = pd.DataFrame({
'id': [104, 105, 106],
'first': ['Mike', 'Nancy', 'Olivia'],
'country': ['Spain', 'France', 'Italy']
})
df_renamed_example5 = df_example5.set_axis(['ID', 'First Name', 'Country'], axis=1)
Original DataFrame:
id first country
0 104 Mike Spain
1 105 Nancy France
2 106 Olivia Italy
After Renaming:
ID First Name Country
0 104 Mike Spain
1 105 Nancy France
2 106 Olivia Italy
Renaming Rows:
Using set_axis
isn't limited to columns; you can rename rows too. Here, we'll rename the index of a DataFrame.
df_example6 = pd.DataFrame({
'Fruit': ['Apple', 'Banana', 'Cherry'],
'Quantity': [5, 6, 7]
})
df_renamed_example6 = df_example6.set_axis(['A', 'B', 'C'], axis=0)
Original DataFrame:
Fruit Quantity
0 Apple 5
1 Banana 6
2 Cherry 7
After Renaming:
Fruit Quantity
A Apple 5
B Banana 6
C Cherry 7
The set_axis
method provides a neat and efficient way to rename the labels of an axis, be it rows or columns. It's particularly handy when you have the need to rename all labels on a specific axis. As with other pandas functionalities, the official pandas documentation offers a comprehensive breakdown of the method, ensuring you can use it to its full potential.
4. In-Place vs. New DataFrame
When working with pandas, you'll often come across methods that have an inplace
parameter. This parameter decides whether the operation should modify the existing DataFrame (inplace=True
) or return a new DataFrame with the changes (inplace=False
, which is often the default).
Understanding this distinction is crucial because:
- Modifying in-place can be memory efficient, as it doesn't create a new object.
- However, in-place modifications can be dangerous because they change the original data, potentially leading to data loss.
Using inplace=True
is akin to making permanent changes, while avoiding it allows you to keep the original DataFrame intact and experiment with variations in a new DataFrame.
For a deep dive into the inplace
parameter and its implications, the official pandas documentation provides insights, especially in the context of methods like rename
.
Using inplace=True
:
Here, we'll rename columns of a DataFrame using the rename
method with inplace=True
.
df_example7 = pd.DataFrame({
'name': ['Peter', 'Quincy', 'Rachel'],
'age': [24, 31, 29]
})
df_example7.rename(columns={'name': 'Full Name', 'age': 'Age'}, inplace=True)
Full Name Age
0 Peter 24
1 Quincy 31
2 Rachel 29
Notice that the original df_example7
was modified in-place and now reflects the new column names.
Avoiding inplace
(Default Behavior):
In this example, the original DataFrame remains unaltered, and the changes are reflected in a new DataFrame.
df_example8 = pd.DataFrame({
'product': ['Shirt', 'Shoe', 'Hat'],
'price': [50, 80, 20]
})
df_renamed_example8 = df_example8.rename(columns={'product': 'Product', 'price': 'Price'})
Original DataFrame:
product price
0 Shirt 50
1 Shoe 80
2 Hat 20
New DataFrame after renaming:
Product Price
0 Shirt 50
1 Shoe 80
2 Hat 20
In this case, the original df_example8
remains unaltered, while the changes are present in the new DataFrame df_renamed_example8
.
The choice between modifying in-place or creating a new DataFrame depends on the specific use case and requirements. In-place modifications can be efficient but come with the risk of unintentional data alteration. On the other hand, working with a new DataFrame keeps the original data safe, allowing for more experimentation without permanent consequences. As always, consulting the official pandas documentation helps ensure you're leveraging the inplace
parameter correctly and effectively.
Wrapping Up
The ability to rename columns in pandas DataFrames is more than just a syntactic operation; it's an essential step in ensuring that your data is clean, readable, and ready for analysis. The various methods provided by pandas, from the straightforward rename
method to the versatile set_axis
approach, allows users to adjust column names based on specific requirements.
Having a firm grasp of these techniques streamlines the data preprocessing phase and ensures that subsequent steps, whether they involve data visualization, statistical analysis, or machine learning, are built on a solid foundation. As with many tasks in data science, mastering the basics, such as renaming columns, can significantly enhance the efficiency and accuracy of your overall data analysis pipeline.
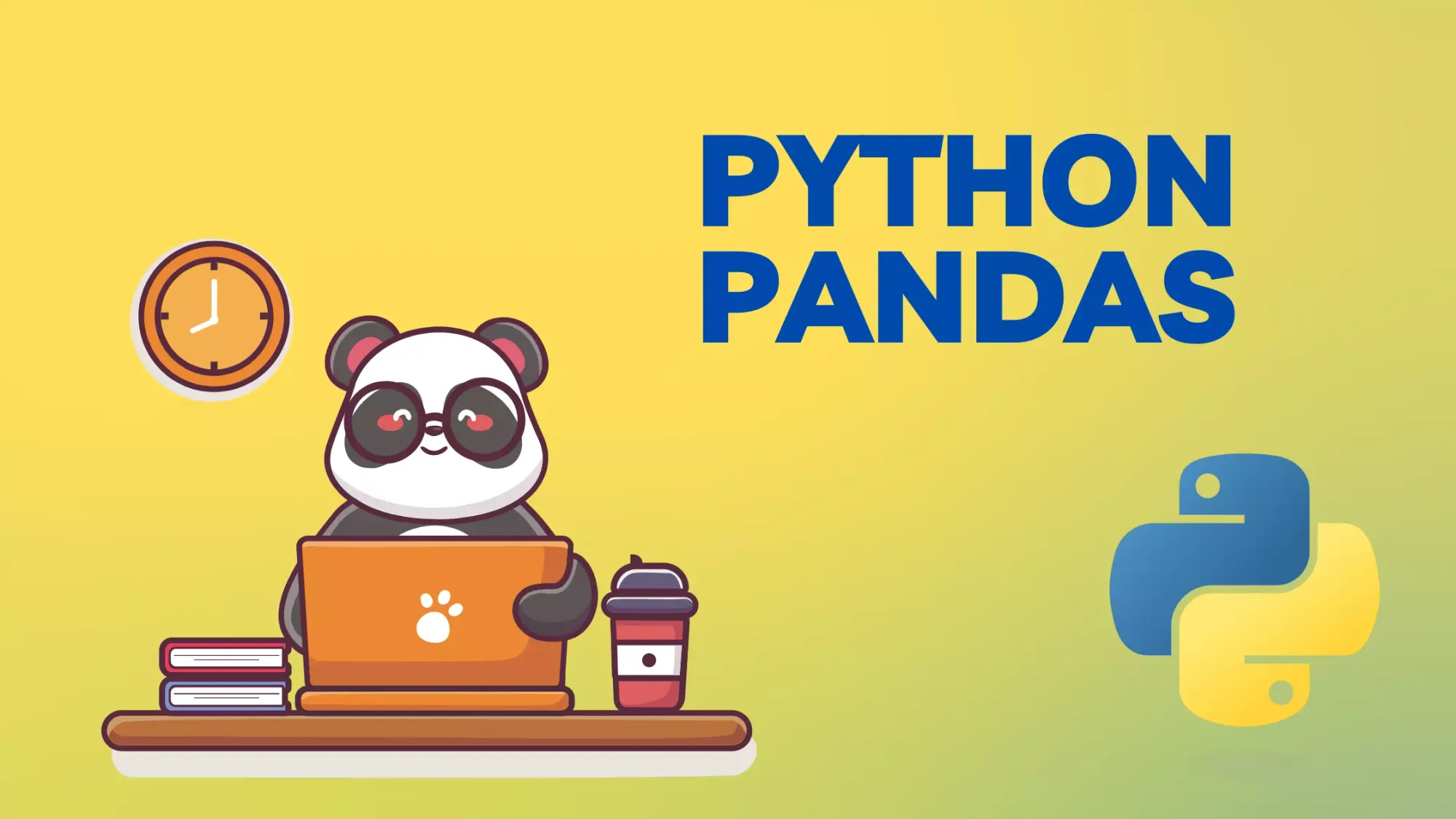