Mastering Column Renaming in R: Techniques and Examples
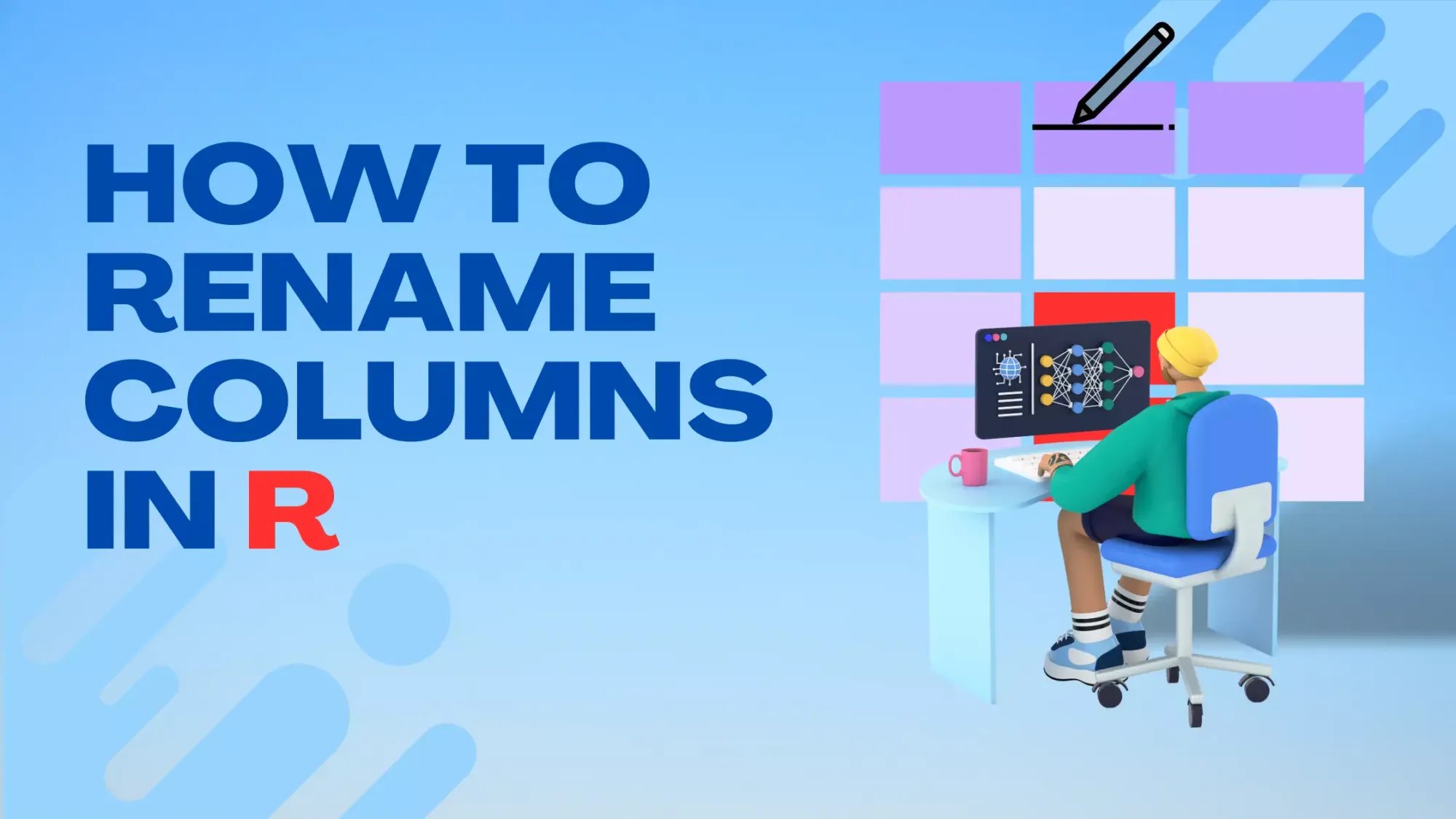
R offers a plethora of ways to rename columns in a dataframe. Renaming columns not only helps in better readability but can also be essential in streamlining data processing tasks, especially when working with large datasets or integrating multiple data sources. It's imperative for data scientists, analysts, and researchers to be adept at this operation to maintain data integrity and clarity.
We'll traverse through the avenues of R's capabilities, discussing different methods to rename columns. Whether you're a beginner getting acquainted with dataframes or a seasoned professional looking for efficient techniques, this guide has something for everyone. We'll present rich examples for each method and provide references to the official documentation, ensuring a holistic understanding of column renaming in R.
Basic Approach using the colnames()
function
The colnames()
function in R provides a direct way to access or set the names of columns in a dataframe. At its core, this function relies on the structure of R's dataframe, which maintains names for its columns as attributes.
Using colnames()
to Retrieve Column Names
Before renaming columns, you can retrieve the current column names:
df <- data.frame(
A = 1:5,
B = 6:10
)
# Retrieve column names
current_names <- colnames(df)
print(current_names)
This will output:
[1] "A" "B"
Renaming Columns
When renaming columns using colnames()
, it's essential to ensure that the new names' vector length matches the total number of columns. Failing to do so will lead to errors.
# Rename columns
colnames(df) <- c("One", "Two")
print(df)
The result after renaming:
One | Two |
---|---|
1 | 6 |
2 | 7 |
3 | 8 |
4 | 9 |
5 | 10 |
Advantages & Caveats
- Simplicity: The primary advantage of this method is its simplicity. It's direct and doesn't require any additional packages.
- Full Rename Required: One of the limitations of this method is that you need to provide names for all columns, even if you intend to rename only a subset. This can be cumbersome for dataframes with a large number of columns.
- Positional Dependency: Since the renaming relies on the order of columns, you need to be cautious when working with datasets where column positions might change.
While colnames()
offers a quick way to rename columns, it's essential to use it judiciously, especially when dealing with complex or large datasets. Being aware of its strengths and limitations will help you choose the right method for your task.
Using the dplyr
package
The dplyr
package is part of the tidyverse
, a collection of R packages designed for data science. Renaming columns becomes particularly intuitive with dplyr
due to its chainable operations and clear syntax. Let's delve deeper into using dplyr
for renaming dataframe columns.
Installation and Setup
If you haven't already installed dplyr
, you can do so using:
install.packages("dplyr")
To use its functions, load the package:
library(dplyr)
Renaming Columns with rename()
The rename()
function from dplyr
allows you to selectively rename columns without needing to specify names for every column:
df <- data.frame(
A = 1:5,
B = 6:10
)
# Rename columns
df_renamed <- df %>%
rename(
One = A,
Two = B
)
print(df_renamed)
The result after renaming:
One | Two |
---|---|
1 | 6 |
2 | 7 |
3 | 8 |
4 | 9 |
5 | 10 |
Benefits of Using dplyr
for Renaming
- Selective Renaming: Unlike the
colnames()
approach, withdplyr
, you can selectively rename columns without addressing every column in the dataframe. - Chainable Operations:
dplyr
functions, includingrename()
, can be chained together using the%>%
operator. This promotes clearer, more readable code. - Clear Syntax: The syntax of
rename()
is very intuitive. It essentially reads as "rename 'this' to 'that'", making your code more self-explanatory.
Potential Caveats
- Dependency on External Package: Unlike the base R approach, you'll need to ensure that
dplyr
is installed and loaded. - Overhead: For very large datasets,
dplyr
operations might introduce some overhead. However, in most real-world scenarios, the benefits of clarity and functionality far outweigh this minor concern.
The dplyr
package brings a combination of flexibility and clarity to column renaming tasks in R. It's especially beneficial for those who prioritize code readability and for tasks that involve a series of data manipulation steps. By understanding its features and potential limitations, you can effectively harness its capabilities for a wide range of data tasks.
Using the data.table
package for renaming columns
The data.table
package in R is a high-performance variant of data.frame
that allows for efficient data manipulation, especially with large datasets. When it comes to renaming columns, data.table
offers a straightforward method via the setnames()
function.
Installation and Setup
To start with, if data.table
isn't already installed, you can add it with:
install.packages("data.table")
Once installed, it's time to load the package:
library(data.table)
Converting a Data Frame to Data Table
It's worth noting that to use the functionalities of data.table
, your dataframe should be a data table. If starting with a dataframe, it can easily be converted:
df <- data.frame(
A = 1:5,
B = 6:10
)
# Convert to data.table
dt <- as.data.table(df)
Renaming Columns with setnames()
The setnames()
function provides a straightforward method to rename columns:
setnames(dt, old = c("A", "B"), new = c("One", "Two"))
print(dt)
The result after renaming
One | Two |
---|---|
1 | 6 |
2 | 7 |
3 | 8 |
4 | 9 |
5 | 10 |
Key Features and Benefits
- In-place Modification: Unlike
dplyr
, which creates a new dataframe with the changes,setnames()
modifies the original data table in-place. This can be more memory-efficient, especially with large datasets. - Performance:
data.table
is optimized for speed, making it a go-to choice for massive datasets. - Selective Renaming: Similar to
dplyr
, you can rename select columns without addressing every column.
Caveats to Consider
- In-place Changes: Since
setnames()
modifies the data table in-place, it's essential to be aware of this side effect, especially if you intend to retain the original column names elsewhere. - Learning Curve: If you're accustomed to data frames and
dplyr
, there might be a slight learning curve when transitioning to thedata.table
syntax.
The data.table
package offers an efficient alternative to both base R and dplyr
methods for renaming columns. Its performance benefits shine with large datasets, and its syntax, once mastered, allows for rapid data manipulations. Being cognizant of its in-place modifications and adopting the necessary precautions can help users leverage data.table
to its fullest potential.
Tips and Best Practices for Renaming Columns in R
The process of renaming columns, while seemingly straightforward, can introduce complexities, especially in larger projects or when collaborating with others. Below are some tips and best practices to ensure this operation is smooth and consistent.
Consistent Naming Conventions
- CamelCase vs. snake_case: Decide on a naming convention early on. Whether you prefer
CamelCase
,snake_case
, or any other format, consistency helps in reading and understanding the data. - Avoid Special Characters: Column names with characters like spaces, hyphens, or symbols can introduce unnecessary complexity. For instance, column names with spaces often require backticks (`) when referenced, making them cumbersome.
Documentation
- Maintain a Change Log: Especially in shared projects, it's beneficial to maintain a change log when columns are renamed. This ensures that all team members are aware of changes and prevents confusion.
- Comment Your Code: If you're renaming columns based on certain conditions or external information, ensure you comment on your rationale. This assists both your future self and any other collaborators.
Testing
- Unit Tests: If you're using a more advanced setup like an R package or a Shiny application, implement unit tests to check column renaming, ensuring that the final column names are as expected.
- Visual Checks: After renaming, always visually inspect the first few rows of your dataframe using functions like
head()
. This quick check can catch unforeseen issues.
Utilize Tools Efficiently
- Right Tool for the Right Job: While tools like
dplyr
anddata.table
are powerful, understand their strengths and weaknesses. For instance, if you're working with an extremely large dataset,data.table
might offer better performance. - Stay Updated: The R ecosystem is vibrant and ever-evolving. Periodically check if there are updates or new packages that offer better functionality or ease in renaming columns.
Backup Original Data
- Never Modify Raw Data: It's a golden rule in data analysis to never directly modify your raw data files. Always work on a copy or an imported version in R, ensuring that the original data remains untouched.
- Use Version Control: Tools like Git can be invaluable in tracking changes, including column renaming. If something goes awry, version control allows you to revert to a previous state easily.
Consider Performance
- Preallocate: If you know you'll be renaming or adding many columns, especially in a loop, preallocating space can improve performance.
- Profile Your Code: If renaming columns is part of a larger data transformation pipeline, use tools like
profvis
to profile your R code. This can help identify bottlenecks or inefficiencies.
Collaboration and Communication
- Align with Team: If you're part of a larger team, align on naming conventions, tools, and methodologies. Consensus reduces confusion and ensures everyone can seamlessly work with the data.
- Feedback Loop: Especially in iterative projects, establish a feedback loop. If column names are found to be confusing or misleading, they can be adjusted in subsequent iterations.
While renaming columns might seem like a basic task, the implications of doing it right are profound, especially in larger projects or shared work environments. By adopting these best practices, you not only ensure that your data remains clear and consistent but also foster an environment that's conducive to efficient and error-free data analysis.
Conclusions
Renaming columns in R is more than just a cosmetic exercise; it plays a crucial role in data preparation, integration, and analysis. As we've navigated through various methods ranging from base R to the extended capabilities of packages like dplyr
and data.table
, it becomes evident that R offers a rich tapestry of tools tailored for a range of needs. Whether you're working with small datasets requiring quick edits or massive ones demanding efficient operations, understanding these techniques is paramount.
However, beyond the technical know-how, it's also essential to approach renaming with a strategic mindset. Always consider the broader context of your data project. Which method is more readable for your team? Which one aligns with the performance needs of your dataset? By coupling the insights from this guide with such considerations, you'll not only ensure clean, well-named dataframes but also foster a more efficient and collaborative data analysis environment.
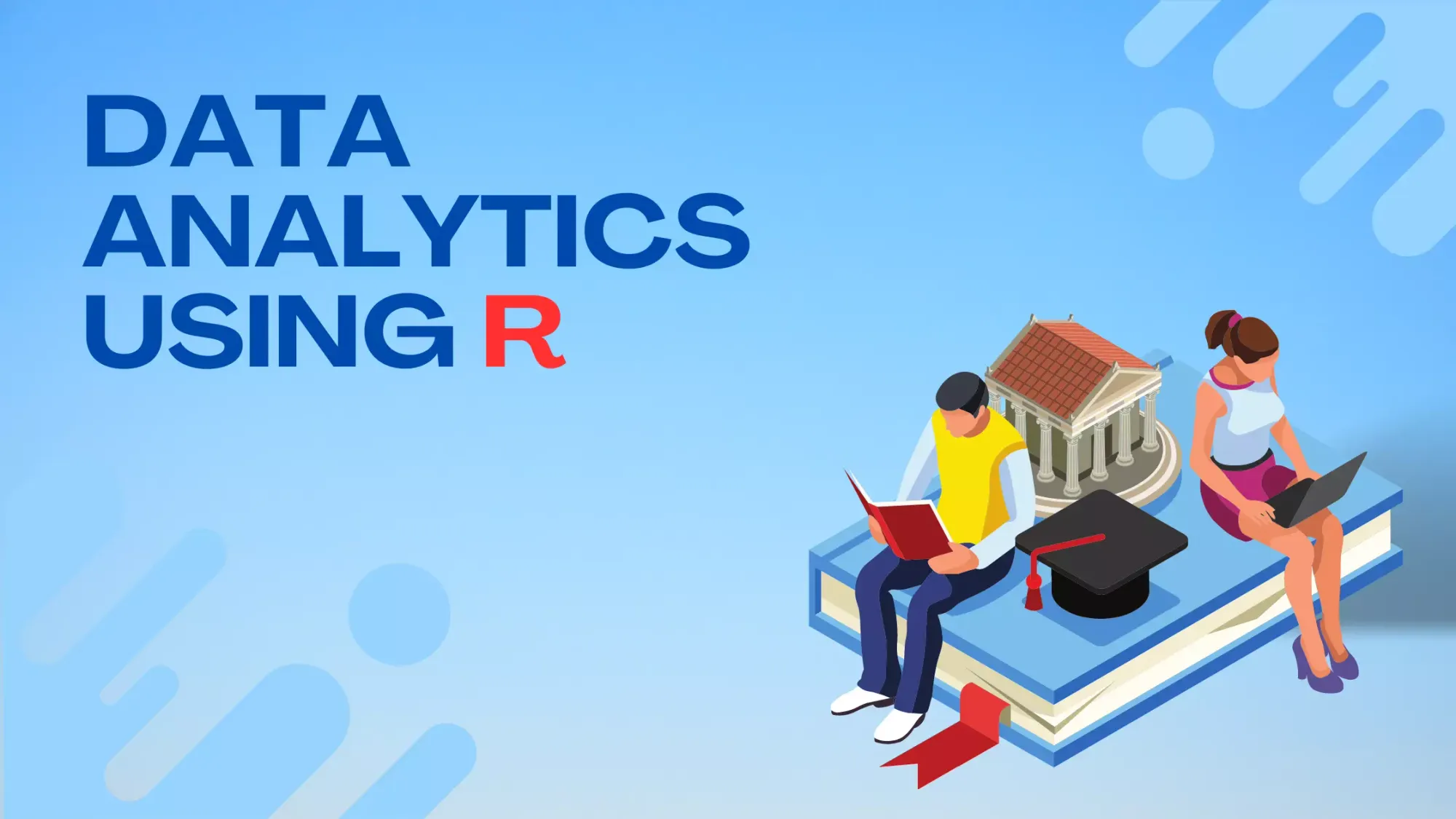