Dropping Columns in R: Multiple Approaches with Examples
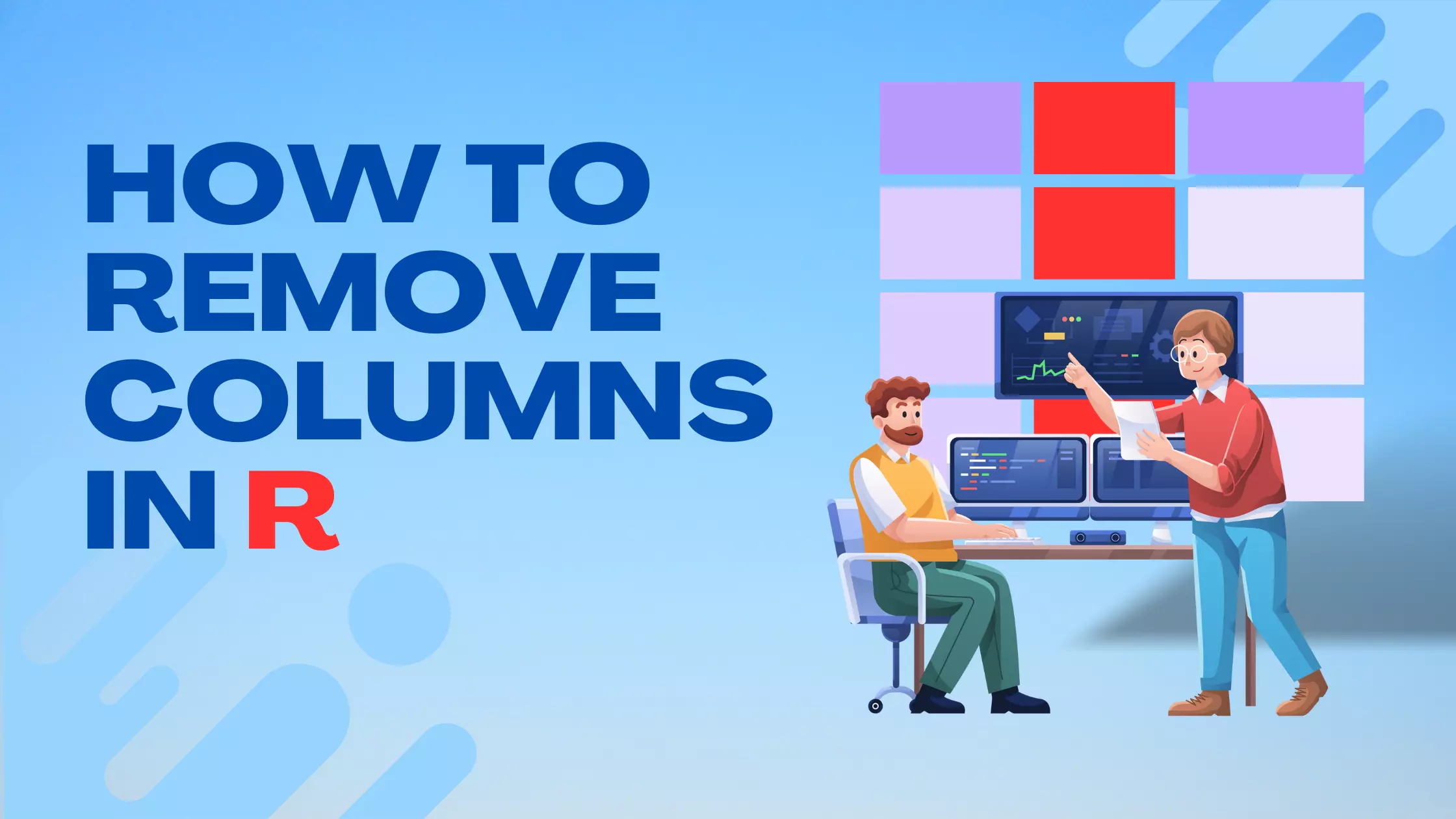
R is a powerful language and environment for statistical computing and graphics. As researchers and data analysts often deal with large datasets, it becomes essential to modify and adjust the dataset's structure to cater to specific analytical needs. Removing or dropping columns is one such frequent operation, especially when dealing with datasets that contain redundant or unnecessary information.
In this guide, we will delve into the multiple techniques available in R for column removal. Whether you are a beginner just starting with R or an advanced user seeking a quick refresher, this article provides step-by-step instructions, accompanied by examples, to make the column removal process seamless and efficient.
Basic Approach: Using the subset
function
The subset
function in R provides a way to extract subsets of rows from a matrix, array, or data frame based on certain conditions. While it's often used to filter rows, it can also be used effectively to select or drop columns.
Syntax
The basic syntax for using the subset
function to drop columns is:
subset(data_frame, select = -column_name)
Here, data_frame
is the name of your data frame and column_name
is the name of the column you wish to drop. The minus sign before the column name indicates that you want to exclude that column.
Example 1: Removing a Single Column
Suppose you have the following data frame:
Name | Age | Gender | Occupation |
---|---|---|---|
Alice | 25 | F | Engineer |
Bob | 30 | M | Doctor |
Carol | 29 | F | Teacher |
To remove the "Gender" column:
data <- data.frame(Name = c("Alice", "Bob", "Carol"),
Age = c(25, 30, 29),
Gender = c("F", "M", "F"),
Occupation = c("Engineer", "Doctor", "Teacher"))
new_data <- subset(data, select = -Gender)
Result:
Name | Age | Occupation |
---|---|---|
Alice | 25 | Engineer |
Bob | 30 | Doctor |
Carol | 29 | Teacher |
Example 2: Removing Multiple Columns
Continuing with the previous dataset, suppose you want to remove both "Gender" and "Occupation" columns:
new_data <- subset(data, select = -c(Gender, Occupation))
Result:
Name | Age |
---|---|
Alice | 25 |
Bob | 30 |
Carol | 29 |
Why use subset
?
The subset
function is a part of base R, meaning it doesn't require any additional packages to be installed. It's intuitive for those who are used to SQL-like syntax, and it also allows for easy row filtering based on conditions.
For a deeper understanding of the subset
function and its various applications, you can refer to the official R documentation. This documentation provides detailed explanations and additional examples for using the function. Access it within R using:
?subset
Or, visit the online documentation at R Documentation.
Using Negative Subset
In R, the process of selecting or excluding columns can be achieved using indexing. Specifically, using negative indexing allows you to exclude certain columns from a data frame. This method leverages the power of R's base indexing system and offers a more direct approach to column removal.
Syntax
The basic syntax to remove columns using negative subset is:
data_frame[,-column_index]
Here, data_frame
is the name of your data frame, and column_index
is the numeric index of the column you want to remove. The negative sign before the index indicates exclusion.
Example 1: Removing a Single Column by Index
Consider the following data frame:
Name | Age | Gender | Occupation |
---|---|---|---|
Alice | 25 | F | Engineer |
Bob | 30 | M | Doctor |
Carol | 29 | F | Teacher |
To remove the "Age" column (which is the 2nd column):
data <- data.frame(Name = c("Alice", "Bob", "Carol"),
Age = c(25, 30, 29),
Gender = c("F", "M", "F"),
Occupation = c("Engineer", "Doctor", "Teacher"))
new_data <- data[,-2]
Expected Result:
Name | Gender | Occupation |
---|---|---|
Alice | F | Engineer |
Bob | M | Doctor |
Carol | F | Teacher |
Example 2: Removing Multiple Columns by Indices
Using the same dataset, let's remove the 1st ("Name") and 3rd ("Gender") columns:
new_data <- data[,-c(1,3)]
Expected Result:
Age | Occupation |
---|---|
25 | Engineer |
30 | Doctor |
29 | Teacher |
Advantages of Negative Subset
- Simplicity: This method uses base R functionality, avoiding the need for any additional packages.
- Directness: By working directly with column indices, you have a clear understanding of which columns are being excluded.
Points to Consider
While the negative subset method is straightforward, it requires you to know the exact index of the columns you want to remove. This can be a limitation if the structure of the data frame changes, as relying on hardcoded indices might lead to errors.
For more insights on indexing in R and its various applications, the official R documentation is a valuable resource. You can access it within R using:
?Extract
Alternatively, you can visit the online documentation on R Documentation to learn more about data extraction and subsetting.
Using the select
function from the dplyr
package
The dplyr
package, a member of the tidyverse
suite, is one of R's most popular packages for data manipulation. The select
function within dplyr
provides a flexible and readable way to choose which columns to retain or remove from a data frame or tibble.
Syntax
To use the select
function from the dplyr
package:
select(data_frame, columns_to_include_or_exclude)
To exclude columns, prefix the column name with a negative sign (-).
Setup
First, if you haven’t already, you'll need to install and load the dplyr
package:
install.packages("dplyr")
library(dplyr)
Example 1: Removing a Single Column with select
Consider the following data frame:
Name | Age | Gender | Occupation |
---|---|---|---|
Alice | 25 | F | Engineer |
Bob | 30 | M | Doctor |
Carol | 29 | F | Teacher |
To remove the "Occupation" column:
data <- data.frame(Name = c("Alice", "Bob", "Carol"),
Age = c(25, 30, 29),
Gender = c("F", "M", "F"),
Occupation = c("Engineer", "Doctor", "Teacher"))
new_data <- select(data, -Occupation)
Result:
Name | Age | Gender |
---|---|---|
Alice | 25 | F |
Bob | 30 | M |
Carol | 29 | F |
Example 2: Removing Multiple Columns with select
Using the previous dataset, to remove both "Name" and "Gender" columns:
new_data <- select(data, -c(Name, Gender))
Result:
Age | Occupation |
---|---|
25 | Engineer |
30 | Doctor |
29 | Teacher |
Additional Features of select
- Using column ranges:
select
allows you to specify a range of columns to retain or exclude using the:
operator. For instance,select(data, Name:Gender)
would only retain the "Name", "Age", and "Gender" columns. - Starts with, ends with, contains: You can use helper functions like
starts_with("prefix")
,ends_with("suffix")
, andcontains("part")
to select columns based on their names.
Advantages of Using select
- Readability: The
select
function offers a clear and concise syntax, making code easier to read and understand. - Flexibility: With helper functions and the ability to specify column ranges,
select
is extremely versatile.
For a more detailed exploration of the select
function and its many features, you should refer to the official dplyr
documentation. This can be accessed in R using:
?select
Or you can delve into the online documentation on R Documentation for an in-depth look at the function and its various applications.
Considerations When Removing Columns
Dropping columns from a dataset may seem like a straightforward operation, but it carries significant implications for data analysis. Before removing columns, one should weigh the reasons for doing so and consider potential pitfalls. This section will dive into some of these considerations, providing examples to illustrate the points raised.
Data Integrity
It's essential to maintain a backup of the original dataset or work with a copy. Once a column is removed, retrieving it without a backup can be challenging or impossible.
Example:
Suppose you're working with a dataset about employees:
ID | Name | Salary | Department |
---|---|---|---|
1 | Alice | 50000 | HR |
2 | Bob | 60000 | Finance |
You decide to remove the "Department" column but later realize it was needed for a department-wise analysis.
To avoid this, always keep an untouched version of your dataset:
original_data <- data
new_data <- data[,-4]
Data Context
Ensure that removing columns doesn't strip the data of valuable context, which might be needed for interpretation or further analysis.
Example:
Consider a dataset about products and their ratings:
Product Name | Rating | Reviews |
---|---|---|
Product A | 4.5 | 100 |
Product B | 3.8 | 5 |
Removing the "Reviews" column might make "Product A" and "Product B" seem closer in quality, even though "Product A" has a more substantial backing for its rating.
Understand Dependencies
Some columns might be required for further data processing or for specific functions to work.
Example:
Consider a dataset used in time series analysis:
Date | Value |
---|---|
2023-01-01 | 100 |
2023-01-02 | 105 |
If you remove the "Date" column, functions that require time series data will fail or give incorrect results.
Avoid Hardcoding Indices
When removing columns based on their indices, be cautious, especially if the dataset structure might change in the future.
Example:
You always remove the 3rd column from a dataset because it used to be an irrelevant "Comments" column. However, if the dataset structure changes and "Age" becomes the 3rd column, you'll inadvertently remove crucial data.
Verify Results
After removing columns, always inspect the resulting dataset to ensure the correct columns were removed and that no unexpected data loss occurred.
Example:
After removing a column, use the head()
function to quickly view the first few rows of the resulting data frame:
new_data <- data[,-3]
head(new_data)
By inspecting the output, you can verify that the desired columns were dropped correctly.
For a deeper understanding of data manipulation and the potential consequences, consider delving into the official R documentation and various R-based data science resources. Accessing the documentation for specific functions can often be done with:
?function_name
For broader topics, the R Documentation website or the CRAN Manuals are excellent places to start.
Conclusions
Removing columns in R is a critical operation, especially when refining datasets for focused analysis. The various techniques showcased in this guide, ranging from the foundational functions in base R to the advanced capabilities of the dplyr
package, demonstrate the flexibility and robustness of R in handling data manipulation tasks. It's essential to choose a method that aligns best with your dataset's structure and your familiarity with R's functionalities.
As you continue to work with R, you'll likely develop a preference for certain methods over others. Regardless of the approach chosen, always prioritize the integrity of your data. Remember to frequently back up your original data, double-check the results after column removal, and always be vigilant of the potential pitfalls in data manipulation to ensure accurate and meaningful analyses.
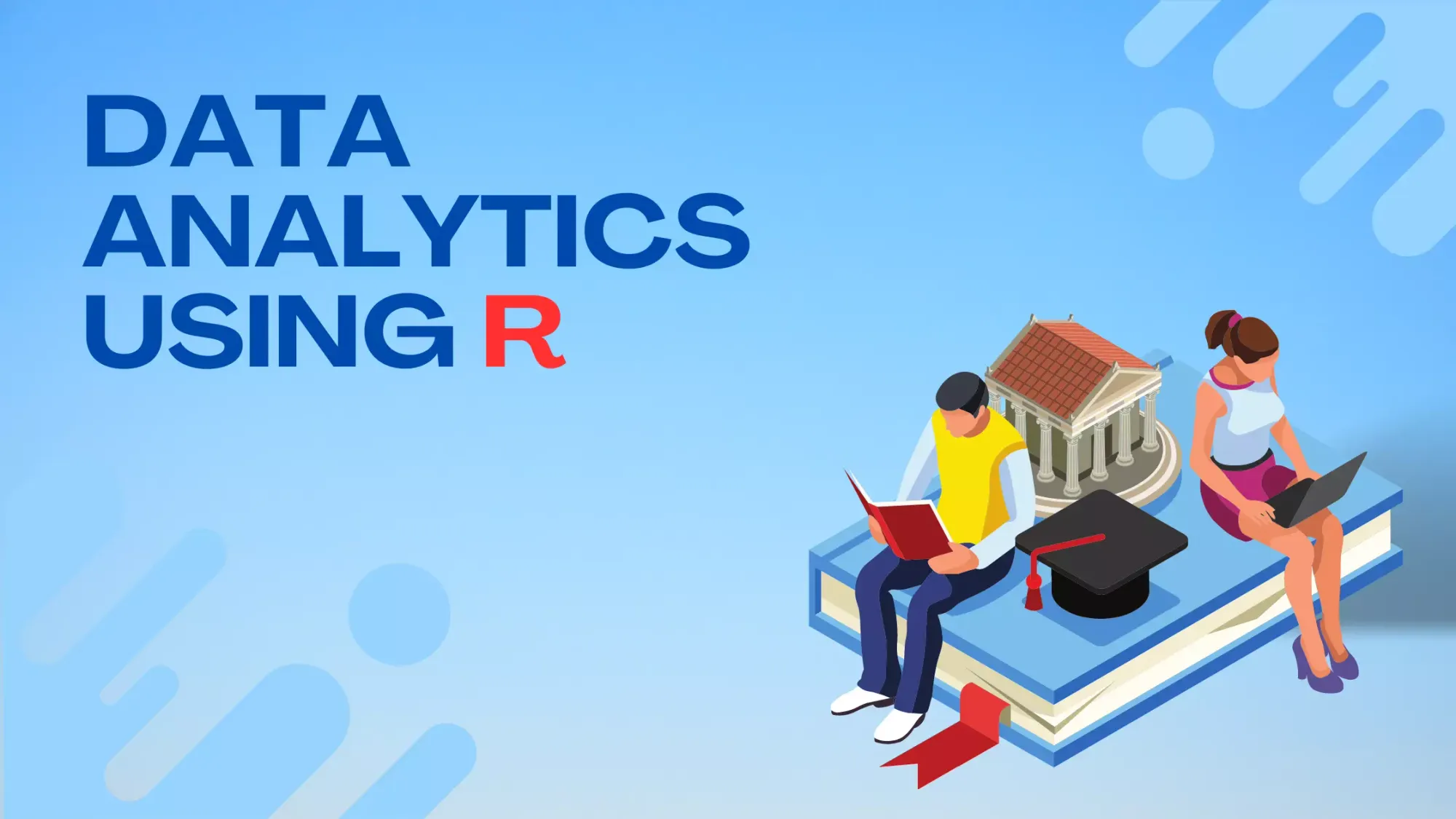