Modifying Data Frames in R: apply()
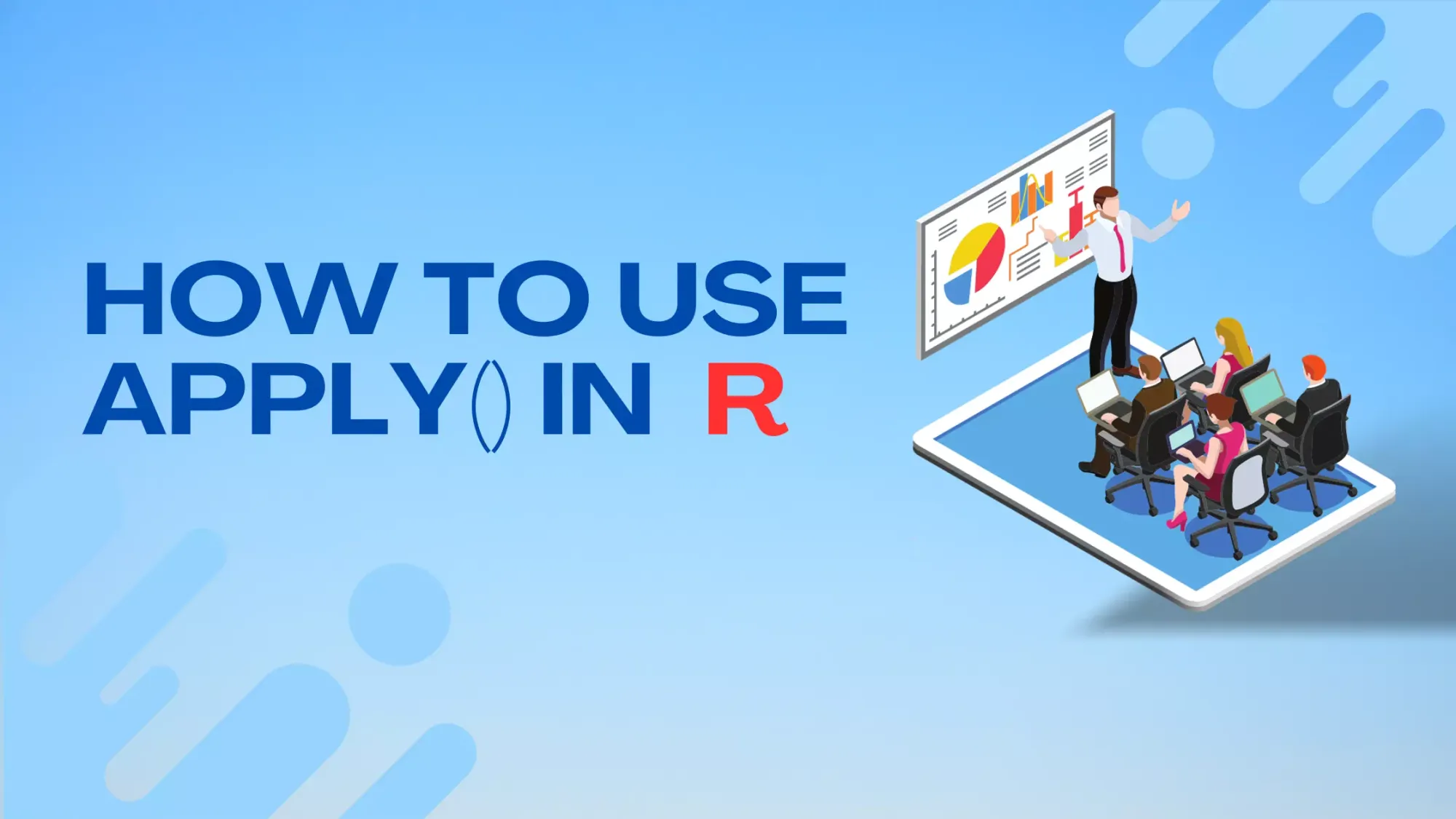
R, a language and environment for statistical computing and graphics, has gained prominence in the data science community for its rich ecosystem and diverse set of tools. It offers an unparalleled combination of flexibility, power, and expressiveness, making it a go-to language for statisticians, data analysts, and researchers alike. A significant aspect of R's appeal is its vast array of built-in functions tailored for efficient data manipulation. Among these, the apply()
function is particularly noteworthy.
The apply()
function in R serves as a cornerstone for many data operations, especially when one wishes to circumvent the use of explicit loops. Loops, while straightforward, can sometimes lead to verbose and slow-executing code. With apply()
, users can achieve more concise code that often runs faster, making it an essential tool in any R programmer's toolkit. This guide seeks to unpack the intricacies of the apply()
function, its diverse applications, and the numerous techniques revolving around it.
Uses case for apply()
apply()
function is a versatile tool for matrix and array manipulations, allowing users to efficiently conduct operations across rows, columns, or both. Its wide-ranging utility spans from statistical computations and data transformations to intricate matrix operations and data-cleaning tasks. Grasping the diverse use cases of apply()
not only streamlines data analysis but also enhances code readability and efficiency. Here, we delve into five notable applications of this powerful function, showcasing its pivotal role in the R data manipulation toolkit.
- Statistical Summaries:
- Calculating row-wise or column-wise means, medians, standard deviations, or any other statistical measure.
- Data Transformation:
- Normalizing or scaling the data row-wise or column-wise.
- Applying a transformation (e.g., logarithmic, square root) to every element in a matrix or specific rows/columns.
- Data Inspection:
- Checking for missing values in each row or column.
- Counting the number of occurrences of a specific value or condition in each row or column.
- Matrix Operations:
- Calculating row or column sums or products.
- Finding the maximum or minimum value in each row or column.
- Data Cleaning:
- Removing or replacing outlier values in each row or column based on a specific criterion.
- Applying a custom function to impute missing values for each row or column.
Basic Usage of apply()
in R
The apply()
function in R is a cornerstone of matrix and array operations. It allows users to apply a function (either built-in or user-defined) across rows, columns, or both of a matrix or array. By leveraging apply()
, you can perform operations without resorting to explicit for-loops, which often results in more concise and readable code.
Syntax:
apply(X, MARGIN, FUN, ...)
X
: an array or matrix.MARGIN
: a vector indicating which margins should be "retained". 1 indicates rows, 2 indicates columns, andc(1,2)
indicates both.FUN
: the function to be applied....
: optional arguments toFUN
.
The official R documentation provides a detailed overview of the apply()
function, which can be found here.
Examples:
1. Sum of each column:
Given a matrix, compute the sum of each column.
mat <- matrix(1:6, nrow=2)
print(mat)
apply(mat, 2, sum)
Output:
[,1] [,2] [,3]
[1,] 1 3 5
[2,] 2 4 6
[1] 3 7 11
2. Sum of each row:
Using the same matrix, compute the sum of each row.
apply(mat, 1, sum)
Output:
[1] 9 12
3. Using built-in functions:
Calculate the range (min and max) for each column.
apply(mat, 2, range)
Output:
[,1] [,2] [,3]
[1,] 1 3 5
[2,] 2 4 6
4. Using a custom function:
Define a custom function to calculate the difference between the maximum and minimum of each row, and then apply it.
diff_range <- function(x) max(x) - min(x)
apply(mat, 1, diff_range)
Output:
[1] 4 4
5. Using apply()
with more than one argument:
To subtract a value from every element of a matrix:
subtract_value <- function(x, val) x - val
apply(mat, c(1,2), subtract_value, val=2)
Output:
[,1] [,2] [,3]
[1,] -1 1 3
[2,] 0 2 4
Remember, while apply()
is a powerful tool for matrix and array manipulations, it's essential to understand the data structure you're working with. For data frames or lists, other functions in the apply
family, like lapply()
or sapply()
, might be more appropriate. Always refer to the official documentation to ensure the correct usage and to explore additional details.
Advanced Usage of apply()
in R
While the basic usage of the apply()
function is straightforward, R provides a depth of versatility that allows for more complex operations. Advanced usage often involves working with multiple arguments, more intricate functions, and understanding potential nuances and pitfalls.
Using apply()
with Additional Arguments:
You can pass extra arguments to the function you're applying by including them after the function name in the apply()
call.
Example:
To raise every element of the matrix to a specified power:
mat <- matrix(1:6, nrow=2)
apply(mat, c(1,2), `^`, 3)
Output:
[,1] [,2] [,3]
[1,] 1 27 125
[2,] 8 64 216
Using apply()
with Custom Functions:
You're not limited to using built-in functions with apply()
. Any user-defined function can be utilized.
Example:
Calculate the median after removing values below a threshold:
mat <- matrix(1:6, nrow=2)
filter_median <- function(x, threshold) {
filtered <- x[x > threshold]
return(median(filtered))
}
apply(mat, 2, filter_median, threshold=2)
Output:
[1] NA 3.5 5.5
Using apply()
on Higher-dimensional Arrays:
While matrices are 2-dimensional, apply()
can be used on arrays of higher dimensions. The MARGIN
argument can take multiple values to specify over which dimensions the function should operate.
Example:
Working with a 3-dimensional array:
arr <- array(1:24, dim=c(2,3,4))
apply(arr, c(1,3), sum)
Output:
[,1] [,2] [,3] [,4]
[1,] 9 27 45 63
[2,] 12 30 48 66
Dealing with Returned Data Structure:
When the result is a single value for each margin (like sum or mean), apply()
returns a simple vector or array. However, if the result is more complex (like quantile
), the result can be multi-dimensional.
Example:
Compute two quantiles (0.25 & 0.75) for each column:
mat <- matrix(1:6, nrow=2)
apply(mat, 2, quantile, probs=c(0.25, 0.75))
Output:
[,1] [,2] [,3]
25% 1.25 3.25 5.25
75% 1.75 3.75 5.75
The official R documentation provides insights into more advanced nuances and potential edge cases. Always reference it when in doubt or when attempting to harness the full power of the apply()
function. Remember, while apply()
is versatile, ensure that it's the right tool for the task at hand and that the returned data structure aligns with your expectations.
Alternatives to apply()
While the apply()
function is a powerful tool for matrix and array manipulations, R provides a family of related functions designed to offer similar functionality across different data structures. Depending on the specific data structure and desired operation, one of these alternative functions may be more appropriate.
Function Name | Description |
---|---|
lapply() |
List Apply - applies a function to each element of a list. |
sapply() |
Simplified lapply - returns a vector or matrix. |
mapply() |
Multivariate lapply - applies a function to multiple list or vector arguments. |
tapply() |
Table Apply - applies a function over a ragged array. |
vapply() |
Similar to sapply(), but you specify the output type. |
Tips and Pitfalls
In the rich landscape of R's data manipulation functions, the apply()
family is versatile and powerful. However, to harness their full potential and avoid common mistakes, it's crucial to understand some tips and potential pitfalls.
Tips:Know Your Data Structure:The apply()
function is primarily designed for matrices and arrays. If you use it with a data frame, it might coerce it into a matrix, potentially leading to unexpected results due to type conversion.For data frames or lists, consider using lapply()
, sapply()
, or other alternatives.Simplify When Needed: The sapply()
function tries to simplify the result to the simplest data structure possible (e.g., from a list to a vector or matrix). If you want more predictable behavior, consider using vapply()
where you can specify the expected return type.Opt for Explicitness with vapply()
: It allows you to explicitly specify the expected return type, adding an extra layer of safety by ensuring the function's output matches your expectations.Avoid Unintended Dimension Reduction: Functions like sapply()
can sometimes reduce the dimension of the output when you might not expect it. If you always want to preserve the output as a list, lapply()
is a safer bet.Pitfalls:Performance Misconceptions:While the apply()
family can lead to cleaner code, it doesn't always guarantee better performance than well-written loops, especially for large datasets.Consider benchmarking your code with larger datasets to ensure performance meets your needs. If not, you might want to explore optimized packages like data.table
or dplyr
.Unexpected Data Type Coercion: Using apply()
on data frames can lead to unexpected type coercions. This is especially problematic when your data frame contains different data types across columns.Overhead with Large Lists: Functions like lapply()
can have overhead when dealing with large lists. In such cases, more optimized approaches or packages might be more suitable.Loss of Data Frame Attributes: When applying certain functions to data frames, you might lose some attributes or metadata. Always check the structure of your output and ensure that no critical information is lost.Misunderstanding Margins: When using apply()
, the MARGIN
argument can sometimes be a source of confusion. Remember, 1 refers to rows, 2 refers to columns, and c(1,2)
refers to both.Complex Output Structures: Functions like tapply()
can produce complex output structures, especially when working with multiple grouping variables. Always inspect the output to ensure you understand its structure and can work with it in subsequent steps.The official R documentation remains a crucial resource, not just for understanding the basic functionality but also for diving into nuances, edge cases, and performance considerations. Always keep it at hand, and when in doubt, refer back to ensure your R coding remains efficient and error-free.ConclusionThe apply()
function in R epitomizes the essence of R's design philosophy: providing powerful tools that simplify complex operations, allowing users to focus more on their data and less on the intricacies of the code. In the vast landscape of R functions designed for data manipulation, apply()
holds a special place due to its versatility in handling matrices and arrays. It offers a glimpse into the potential of R, where a single function can often replace multiple lines of looped code, leading to cleaner and more maintainable scripts.However, as with any tool, the true mastery of apply()
comes not just from understanding its basic mechanics but from recognizing when and how to use it effectively. This includes being aware of its best use cases, its limitations, and the availability of alternative functions that might be better suited for specific tasks. The journey of mastering R is filled with continuous learning, and we hope this guide has brought you one step closer to harnessing the full potential of the apply()
function and, by extension, R itself.
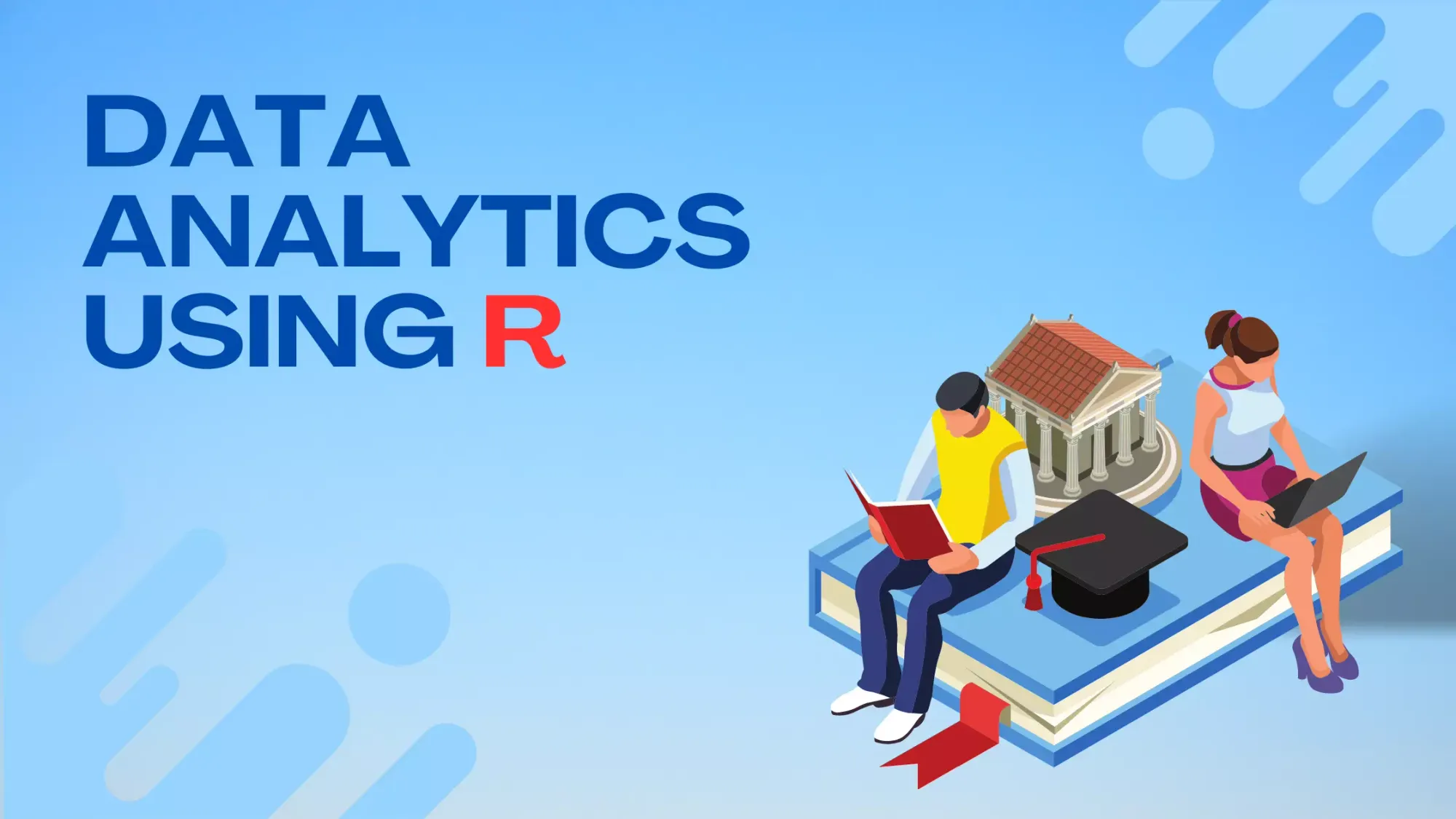