How to Drop Columns in Pandas
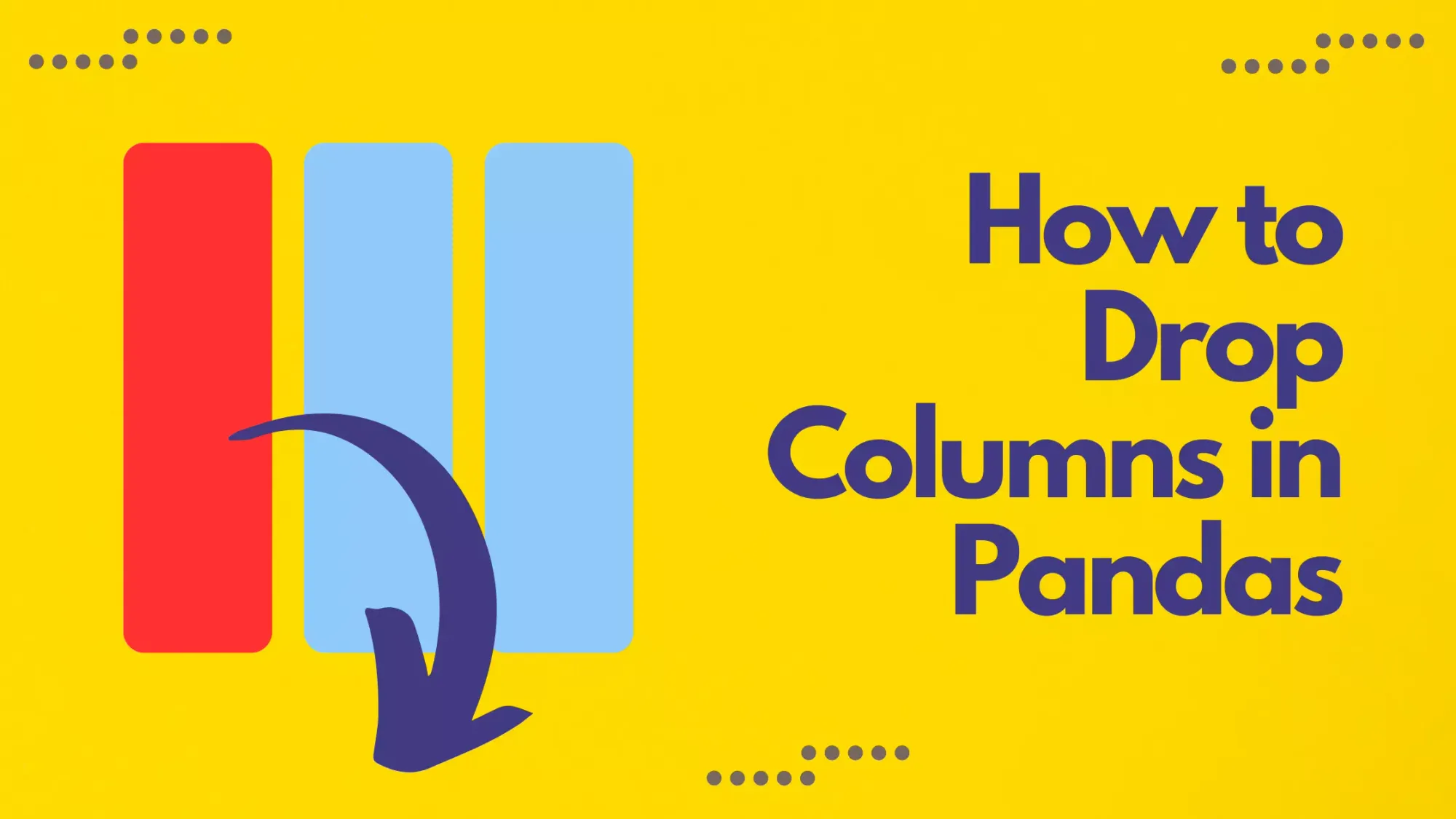
Working with large datasets often requires constant reshaping, cleaning, and manipulation. One of the most common operations, especially in the preliminary stages of data analysis, is dropping unnecessary columns. The Pandas library in Python offers a versatile set of tools to help data scientists and analysts accomplish this task efficiently. This article delves deep into various methods to drop columns from a Pandas DataFrame, emphasizing practical examples, performance considerations, and tips for handling large datasets.
Pandas is a robust library that has become synonymous with data manipulation in Python. While dropping columns might seem like a straightforward task, doing so efficiently and without pitfalls, especially with extensive datasets, requires a good understanding of the library's capabilities.
Methods for dropping data frame columns
Manipulating the structure of a DataFrame is a foundational skill in data analysis, and dropping columns is a fundamental operation within this skill set. The Pandas library, with its comprehensive and flexible features, has established itself as the go-to tool for such tasks. One of the primary methods to remove columns from a DataFrame in Pandas is using the drop
method. While it might seem straightforward initially, to leverage its full potential, one must understand its diverse parameters and their implications. In this section, we'll delve into the nuances of this method, ensuring you have a robust understanding of its capabilities. For a more exhaustive explanation and additional use-cases, the official Pandas documentation is an invaluable resource.
Syntax:
The syntax for the drop
method when dropping columns is:
pythonCopy codeDataFrame.drop(labels=None, axis=1, columns=None, inplace=False
)
labels
: The labels to drop. This could be a single label or a list of labels.axis
: The axis along which labels are dropped. For columns, this value is1
.columns
: An alternative to specifyingaxis=1
. You can provide the column name or names directly.inplace
: IfTrue
, the DataFrame is modified in place and nothing is returned. IfFalse
, a new DataFrame with the columns dropped is returned.
Example 1: Dropping a Single Column
import pandas as pd
# Sample DataFrame
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
})
# Drop column 'A'
df.drop(columns='A', inplace=True)
print(df)
The output DataFrame has successfully dropped the column 'A'.
B C
0 4 7
1 5 8
2 6 9
Example 2: Dropping Multiple Columns
Dropping more than one column at a time is just as easy.
# Sample DataFrame
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9],
'D': [10, 11, 12]
})
# Drop columns 'A' and 'D'
df.drop(columns=['A', 'D'], inplace=True)
print(df)
B C
0 4 7
1 5 8
2 6 9
Example 3: Dropping a Column Using axis
Instead of using the columns
parameter, you can use the axis
parameter to specify that you want to drop columns.
# Sample DataFrame
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
})
# Drop column 'A'
df.drop('A', axis=1, inplace=True)
print(df)
B C
0 4 7
1 5 8
2 6 9
Example 4: Dropping a Column Without the columns
Parameter
You can use the labels
and axis
parameters together as an alternative to using the columns
parameter.
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9],
'D': [10, 11, 12]
})
# Drop column 'B'
df.drop(labels='B', axis=1)
A C D
0 1 7 10
1 2 8 11
2 3 9 12
Example 5: Error Handling
It's essential to be aware of potential errors. If you try to drop a column that doesn't exist, Pandas will raise a KeyError
. To avoid this, you can use the errors
parameter and set it to 'ignore'
.
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9],
'D': [10, 11, 12]
})
# Attempting to drop a non-existent column 'Z'
df.drop(columns='Z', errors='ignore')
A B C D
0 1 4 7 10
1 2 5 8 11
2 3 6 9 12
With this setting, if the column doesn't exist, no error will be raised, and the original DataFrame will be returned.
Example 6: Dropping Columns Using Column Index
Instead of using column names, you can use column indices to drop columns. This approach can be useful when you don't know the column names or when the DataFrame has unnamed columns.
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9],
'D': [10, 11, 12]
})
# Drop the first and third columns
df.drop(df.columns[[0, 2]], axis=1)
B D
0 4 10
1 5 11
2 6 12
In this method, df.columns
returns an Index object containing all column names. By providing a list of indices, you can select specific columns to drop.
Example 7: Using Conditional Logic
At times, you might want to drop columns based on specific conditions, such as columns with a certain prefix or columns with a certain data type.
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9],
'D': [10, 11, 12]
})
# Drop columns with names starting with 'B'
df.drop(columns=[col for col in df if col.startswith('B')])
A C D
0 1 7 10
1 2 8 11
2 3 9 12
The basic method of dropping columns in Pandas is facilitated by the versatile drop
method. By understanding its parameters and how they interact, you can fine-tune your column removal tasks to cater to a variety of scenarios. Whether you're working with column names, indices, or conditional logic, the drop
method offers a straightforward and effective approach.
Practical Tips and Performance Considerations
Especially when handling vast datasets, the nuances in how you utilize Pandas can profoundly impact both memory usage and computational speed. This section offers practical insights and performance-centric strategies to optimize your column-dropping tasks, ensuring a harmonious balance between precision and performance. Dive in to uncover these tailored recommendations and elevate your Pandas proficiency.
Tip 1: Caution with inplace=True
Using inplace=True
modifies the DataFrame directly without returning anything. While it can be beneficial for memory savings, there are things to be wary of:
- Chaining: Avoid chaining operations after an
inplace
operation. It can lead to unexpected behavior or errors. - Debugging: If you mistakenly drop the wrong columns using
inplace=True
, you cannot undo the operation without reloading or reconstructing the DataFrame. This can be problematic during the iterative process of data exploration and analysis.
Tip 2: The Power of del
The del
statement offers a quick way to remove columns. It directly modifies the DataFrame and can be more intuitive for those coming from other programming languages.
- Memory Efficiency:
del
is memory-efficient as it modifies the DataFrame in place without any function overhead.
Tip 3: Optimize Data Types
Before or after dropping columns, consider optimizing the data types of your DataFrame. Pandas offers the astype()
method to convert column data types.
- Memory Savings: Columns with types like
float64
orint64
can often be converted tofloat32
orint32
, respectively, saving memory.
Tip 4: Check Before Dropping
Before dropping columns, especially if it's based on conditions, always verify what you're about to remove. Use the head()
or sample()
methods to inspect the data before making irreversible changes.
Consideration 1: Dropping Multiple Columns Simultaneously
If you plan to drop several columns, it's more efficient to drop them all at once rather than one by one. This reduces the overhead and computational cost of the operation.
Consideration 2: Column Contiguity
In memory, DataFrame columns are stored contiguously. When dropping non-contiguous columns, the operation might be slightly slower than dropping contiguous ones due to memory reallocation. While this isn't a significant concern for most use cases, it's good to be aware of when working with very large DataFrames.
Consideration 3: Use iloc
for Large Drops
If you're dropping a significant portion of your columns based on their position, using iloc
can be efficient:
# Drop all columns except the first two
df = df.iloc[:, :2]
This method avoids the overhead of column name lookups and can be faster for extensive drops.
Performance Consideration 4: Sparse DataFrames
If you're working with data that's largely missing or NaN, consider converting your DataFrame to a sparse format using pd.SparseDataFrame
(in older Pandas versions) or the astype
method in newer versions. Sparse formats can save memory and make dropping columns faster.
By combining practical knowledge with performance considerations, you can ensure that your data manipulation processes are both effective and efficient. Always tailor your approach to the specific needs of your dataset and the problem you're trying to solve.
Working with Large Data Sets
Immense datasets not only demand more memory but also introduce complexities that can slow down processing. Mastering the art of handling such colossal data in Pandas is essential for efficient data wrangling. This section delves into strategies and best practices tailored for these expansive datasets, ensuring you sail smoothly through the vast sea of data, even when performing seemingly simple tasks like dropping columns.
Strategy 1: Load Only Required Columns
Loading only the columns you need is the first line of defense against memory issues. It reduces memory overhead and makes the subsequent operations quicker.
- Using
usecols
: When reading a dataset, theusecols
parameter allows you to specify the columns you wish to load.
Strategy 2: Efficient Memory Usage
Pandas often defaults to data types that are more general but might be overkill for your data. For instance, it might use float64
when float32
would suffice.
- Inspect Data Types: Use
df.info()
to get an overview of data types and memory usage. - Convert Data Types: Use
astype()
to convert columns to more memory-efficient data types.
Strategy 3: Drop Columns Early
If you have a pipeline of operations to perform on your data, consider dropping unnecessary columns as early as possible. It ensures that subsequent operations are faster and more memory-efficient.
Working with large datasets in Pandas requires a blend of the right techniques, awareness of memory and computational constraints, and a focus on efficiency. By adopting these strategies, you can handle vast datasets effectively, making your data processing tasks smoother and more scalable.
Wrapping Up
The art of data manipulation lies in understanding the nuances of your tools. With Pandas, dropping columns is more than a mere operation—it's a strategy. It not only declutters your dataset but also enhances performance, especially when dealing with large volumes of data.
However, remember that each data column you drop is a piece of information lost. Always ensure you are discarding columns based on robust data understanding and not just for convenience. With the practical tips and performance considerations discussed, you're now well-equipped to handle column removal tasks in Pandas proficiently.
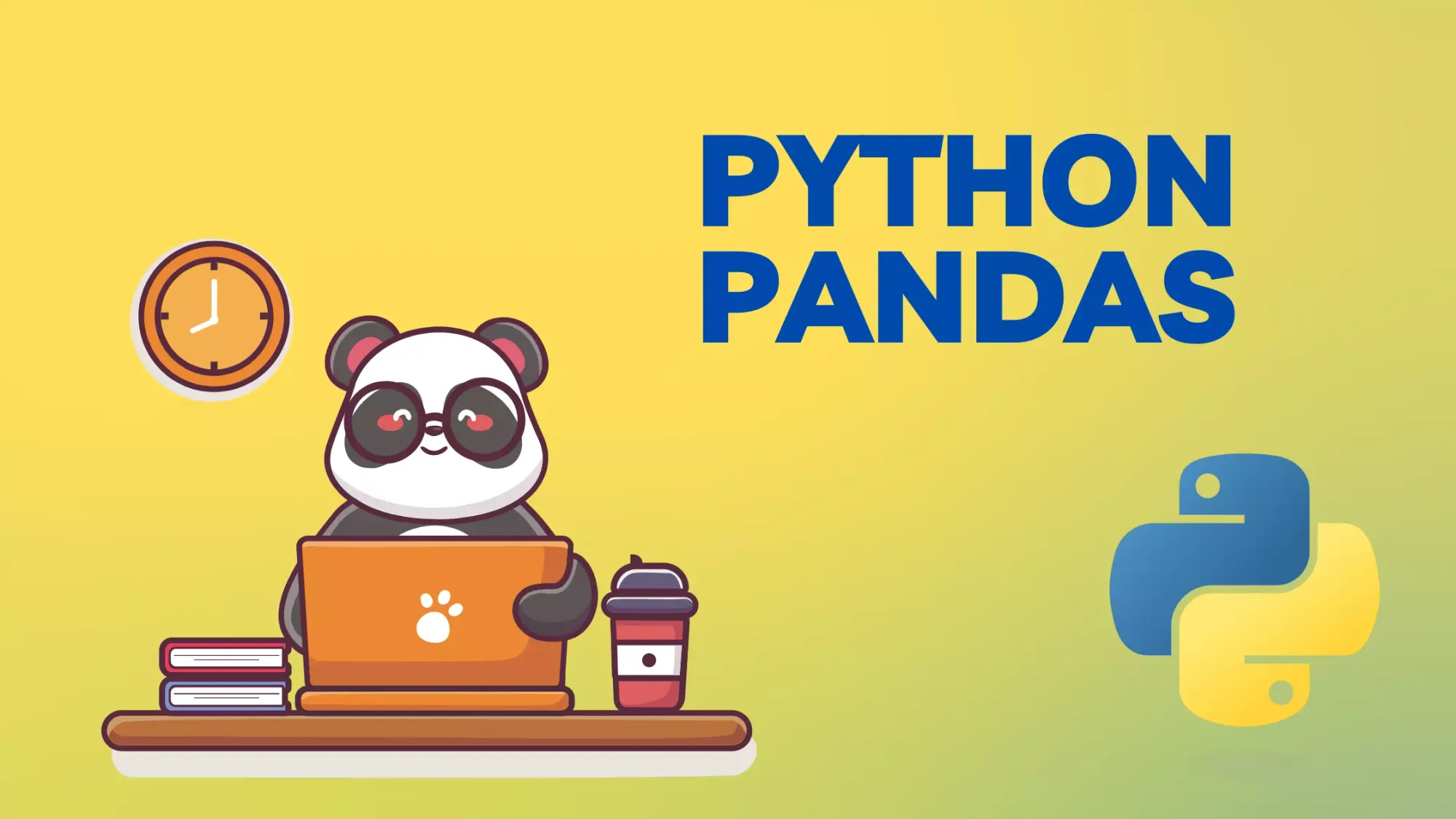