Calculating Matrix Exponentials Using Python
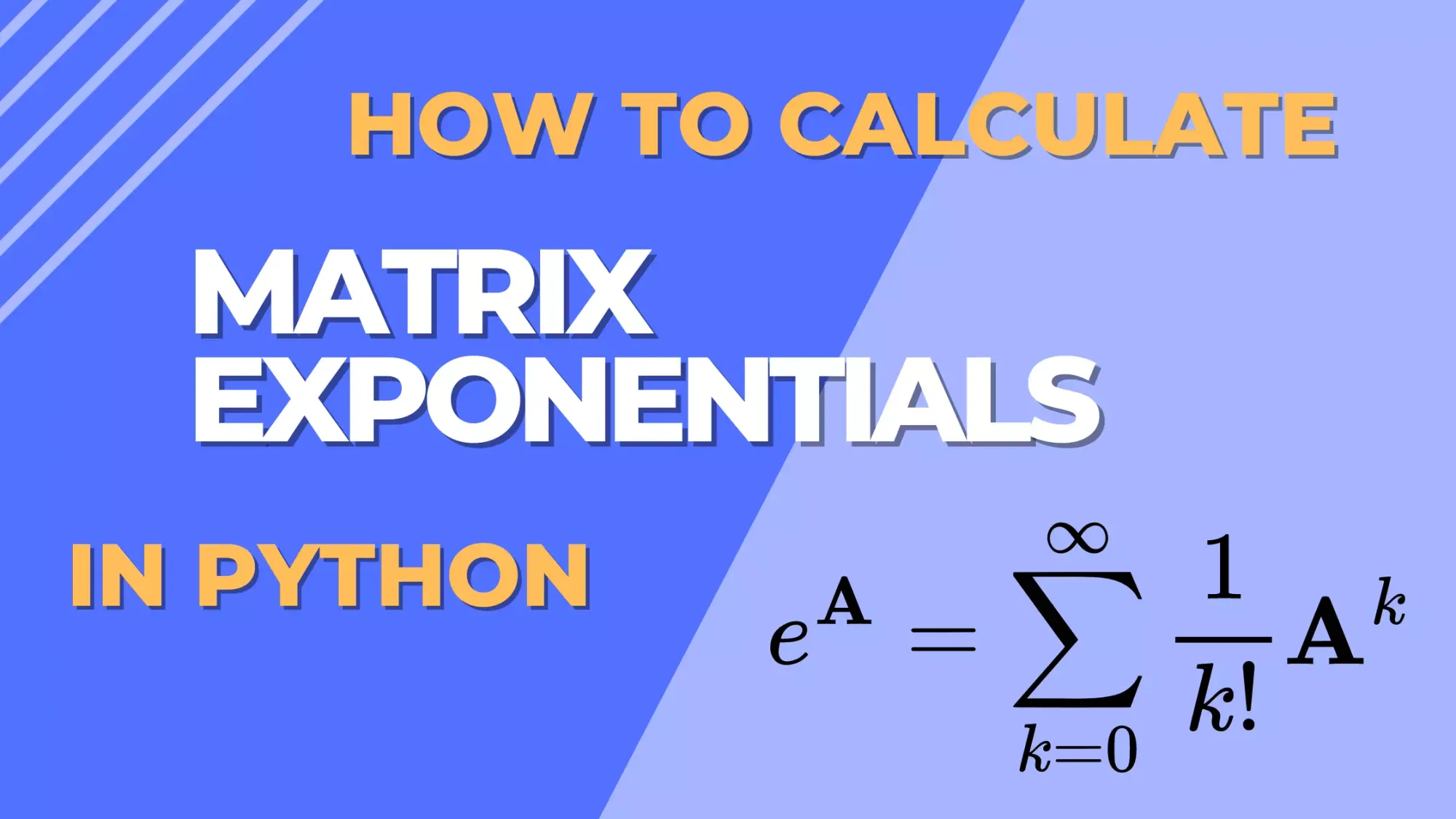
Matrix exponentials, an enthralling intersection of linear algebra and complex analysis, are ubiquitous in the annals of mathematics, physics, and engineering. These constructs, extending the concept of exponential functions to matrices, serve as a linchpin in myriad applications, from the quantum oscillations of particles to the dynamic behaviors in control systems. However, the actual computation of matrix exponentials, particularly for large or intricate matrices, presents a fascinating challenge.
In our expedition into this mathematical landscape, we'll embark on a journey through three distinct yet interconnected pathways to compute matrix exponentials: the Direct Computation rooted in the essence of infinite series, the Eigenvalue Approach that capitalizes on the inherent properties of matrices, and the cutting-edge computational prowess of the scipy.linalg.expm
method. As we traverse these routes, we'll not only unravel the theoretical underpinnings but also witness the harmonious dance of theory and computation, enabling us to harness the true potential of matrix exponentials in diverse applications.
Fundamentals of Matrix Exponentials
The definition of matrix exponential is usually given using the Power series. Let \(\mathbf{X}\) denote a \(5 \times 5\) square matrix. Then, the matrix exponential is defined as
\[
e^\mathbf{X} = \sum_{k=0}^\infty {1 \over k!}\mathbf{X}^k,
\]
where \(\mathbf{X}^0 = \mathbf{I}\) and \(\mathbf{I}\) denotes the identity matrix.
The power series definition is fairly complex and does not give us too much insight. We can take a more in-depth look by taking the eigenvalue decomposition of \(\mathbf{X} = \mathbf{U}\mathbf{D}\mathbf{U}^{-1}\), where \[
\mathbf{D} = \left[
\begin{array}{cccc}
\lambda_1 & 0 & \cdots & 0 \\
0 & \lambda_2 & \cdots & 0 \\
\vdots & \vdots & \ddots & \vdots \\
0 & 0 & \cdots & \lambda_n
\end{array}
\right]
\] and \(\lambda_i\) denotes the \(i^{th}\) eigenvalue.
Now, we can rewrite the matrix exponential as
\[
e^\mathbf{X}
= \sum_{k=0}^\infty {1 \over k!}(\mathbf{U}\mathbf{D}\mathbf{U}^{-1})^k
= \sum_{k=0}^\infty {1 \over k!}(\mathbf{U}\mathbf{D}\mathbf{U}^{-1})^k
\]
\[
\hphantom{e^\mathbf{X}}
= \sum_{k=0}^\infty {1 \over k!}\mathbf{U}\mathbf{D}^k\mathbf{U}^{-1}
= \mathbf{U}\left(\sum_{k=0}^\infty {1 \over k!}\mathbf{D}^k\right)\mathbf{U}^{-1}
\]
By noting that \[
\sum_{k=0}^\infty {1 \over k!} \lambda_i^k = e^\lambda_i.
\]
We can finally write the matrix exponential as
\[
e^\mathbf{X} = \mathbf{U} \bar{\mathbf{D}} \mathbf{U}^{-1},
\]
where \[
\bar{\mathbf{D}} = \left[
\begin{array}{cccc}
e^{\lambda_1} & 0 & \cdots & 0 \\
0 & e^{\lambda_2} & \cdots & 0 \\
\vdots & \vdots & \ddots & \vdots \\
0 & 0 & \cdots & e^{\lambda_n}
\end{array}
\right].
\]
We can see how the matrix exponential pertains to the "shape" of the matrix (eigenvectors) and exponential scales the proportions (eigenvalues).
Calculating matrix exponential using Python
Matrix exponentials, fundamental in various fields like quantum mechanics, control theory, and differential equations, hold the power to transform our mathematical computations. But how do we calculate them, especially when dealing with complex matrices? This section delves into three prominent methods to compute matrix exponentials:
- Using
scipy.linalg.expm
: A modern, sophisticated method powered by the SciPy library, this approach uses algorithms like the Pade approximation and scaling & squaring to efficiently compute matrix exponentials. - Eigenvalue Approach: Harnessing the properties of diagonalizable matrices, this method leverages eigenvalues and eigenvectors to simplify and efficiently compute the matrix exponential.
- Direct Computation: A method rooted in the very definition of matrix exponentials, this approach uses the infinite series expansion to approximate \( e^A \).
Each method has its own strengths, applications, and considerations. As we journey through each, we'll uncover their intricacies, explore their computations, and understand their relevance in various scenarios. Whether you're a budding mathematician, an engineer, or someone curious about the world of matrices, this section promises a deep dive into the captivating realm of matrix exponentials.
Using scipy.linalg.expm
The expm
function in scipy.linalg
is designed to compute the matrix exponential using the Al-Mohy and Higham's 2009 algorithm, which leverages the Pade approximation and scaling & squaring. This method is efficient and provides accurate results for a wide variety of matrices.
Basic Usage:
from scipy.linalg import expm
result = expm(A)
where A
is the matrix for which you want to compute the exponential.
Algorithm:
The algorithm behind scipy.linalg.expm
is based on the Pade approximation and the scaling & squaring technique:
- Pade Approximation: This is a method to approximate a function using rational functions. For matrix exponentials, it involves approximating \( e^A \) with a rational function of \( A \). This avoids the need to compute higher powers of \( A \) directly.
- Scaling & Squaring: Before applying the Pade approximation, the matrix \( A \) is scaled down by a factor (by powers of 2) so that its norm becomes sufficiently small. After the Pade approximation is applied to this scaled matrix, the result is squared repeatedly to undo the scaling and obtain \( e^A \).
Advantages:
- Efficiency: The algorithm is designed to be efficient for a wide range of matrices, making it faster than naive methods like the direct computation for many cases.
- Robustness: The method is robust and can handle ill-conditioned matrices and matrices with large norms.
For detailed specifics, options, and any updates to the function, you can refer to the official documentation of scipy.linalg.expm
.
Example:
For our matrix:
\[
A = \begin{bmatrix}
0 & 1 \\
-2 & -3 \\
\end{bmatrix}
\]
import numpy as np
from scipy.linalg import expm
# Matrix A
A = np.array([[0, 1], [-2, -3]])
# Results
scipy_result = expm(A)
scipy_result
Using scipy.linalg.expm
, we get
\[
e^A \approx \begin{bmatrix}
0.6004 & 0.2325 \\
-0.4651 & -0.0972 \\
\end{bmatrix}
\]
The scipy.linalg.expm
function is a reliable and efficient tool for computing matrix exponentials in Python. It abstracts away the complexities of advanced algorithms, providing users with an easy-to-use function that yields accurate results. If you're working on applications that require matrix exponentials, especially for larger matrices, this function is an invaluable asset.
Eigenvalue Approach to Matrix Exponential:
The fundamental idea behind this approach is to leverage the properties of diagonalizable matrices and their eigenvalues to simplify the computation of the matrix exponential.
If a matrix \( A \) is diagonalizable, then it can be expressed in the form:
\[
A = V D V^{-1}
\]
where:
- \( V \) is a matrix whose columns are the eigenvectors of \( A \).
- \( D \) is a diagonal matrix whose diagonal entries are the eigenvalues of \( A \).
Now, the matrix exponential \( e^A \) can be computed as:
\[
e^A = V e^D V^{-1}
\]
The beauty of this method is that the exponential of a diagonal matrix \( e^D \) is straightforward to compute: it is a diagonal matrix where each diagonal entry is the exponential of the corresponding diagonal entry of \( D \).
Steps to Compute Using the Eigenvalue Approach:
- Compute Eigenvectors and Eigenvalues: Decompose matrix \( A \) to get its eigenvectors (forming matrix \( V \)) and eigenvalues (forming diagonal matrix \( D \)).
- Compute the Exponential of the Diagonal Matrix: For matrix \( e^D \), take the exponential of each of its diagonal entries.
- Reconstruct the Matrix Exponential: Compute \( e^A = V e^D V^{-1} \).
Considerations:
- Diagonalizability: This method is applicable only if the matrix \( A \) is diagonalizable. Not all matrices are diagonalizable, but many that arise in practical applications are.
- Computational Cost: The eigen decomposition step can be computationally intensive for large matrices. However, once the eigenvectors and eigenvalues are known, the matrix exponential is relatively easy to compute.
- Numerical Stability: For certain matrices, computing the inverse of \( V \) can be numerically unstable. Special care or alternative techniques might be required in such cases.
Example:
Given the matrix:
\[
A = \begin{bmatrix}
0 & 1 \\
-2 & -3 \\
\end{bmatrix}
\]
We can compute \( e^A \) using the eigenvalue approach. Let's walk through the steps and compute the matrix exponential.
import numpy as np
# Matrix A
A = np.array([[0, 1], [-2, -3]])
# Eigenvalue Approach
def matrix_exponential_eigen(A):
eigvals, eigvecs = np.linalg.eig(A)
diag_exp = np.diag(np.exp(eigvals))
return eigvecs @ diag_exp @ np.linalg.inv(eigvecs)
# Results
eigen_result = matrix_exponential_eigen(A)
eigen_result
Let's walk through the eigenvalue approach for matrix \( A \):
Eigenvectors and Eigenvalues:
Eigenvalues (\( \lambda \)):
\[
\lambda_1 = -1, \quad \lambda_2 = -2
\]
Eigenvectors (\( v \)):
\[
v_1 = \begin{bmatrix}
0.7071 \\
-0.7071 \\
\end{bmatrix}
\]
\[
v_2 = \begin{bmatrix}
-0.4472 \\
0.8944 \\
\end{bmatrix}
\]
Exponential of the Diagonal Matrix:
\[
e^D = \begin{bmatrix}
e^{-1} & 0 \\
0 & e^{-2} \\
\end{bmatrix}
= \begin{bmatrix}
0.3679 & 0 \\
0 & 0.1353 \\
\end{bmatrix}
\]
Reconstructing the Matrix Exponential:
\[
e^A \approx \begin{bmatrix}
0.6004 & 0.2325 \\
-0.4651 & -0.0972 \\
\end{bmatrix}
\]
This result is consistent with what we observed from the scipy
method and the direct computation with sufficient terms.
Direct Computation of Matrix Exponential:
The direct computation of the matrix exponential using the infinite series is a straightforward approach based on the definition of the matrix exponential. Let's explore this method in more detail.
The matrix exponential of a matrix \( A \) is defined by the infinite series:
\[
e^A = I + A + \frac{A^2}{2!} + \frac{A^3}{3!} + \frac{A^4}{4!} + \dots
\]
Here:
- \( A \) is a matrix.
- \( I \) is the identity matrix of the same size as \( A \).
- \( A^2, A^3, \), etc., are powers of the matrix \( A \).
The series is an analogy to the Taylor series expansion of the exponential function, given by:
\[
e^x = 1 + x + \frac{x^2}{2!} + \frac{x^3}{3!} + \frac{x^4}{4!} + \dots
\]
For matrices, the scalar \( x \) is replaced by the matrix \( A \), and scalar multiplication is replaced by matrix multiplication.
Steps to Compute Using the Direct Method:
- Initialization: Start with the identity matrix, \( e^A = I \).
- Iteration: For each subsequent term, compute the next power of \( A \) and divide by the factorial of the current term's order. Add this term to the sum.
- Truncation: In practice, the infinite series is truncated after a certain number of terms to make computation feasible. The number of terms to consider depends on the required accuracy and the properties of matrix \( A \).
Considerations:
- Convergence: The series is guaranteed to converge for all matrices \( A \), meaning the terms will get smaller and approach zero as the series progresses.
- Accuracy vs. Computation: The more terms you consider in the series, the more accurate the approximation of \( e^A \) will be. However, computation becomes more intensive with each term due to matrix multiplication and increasing factorials.
Example:
Given the matrix:
\[
A = \begin{bmatrix}
0 & 1 \\
-2 & -3 \\
\end{bmatrix}
\]
Let's compute \( e^A \) using the direct method for different truncations of the series (say, up to 5 terms, 10 terms, and 20 terms) and see how the result evolves.
import numpy as np
from scipy.linalg import expm
# Matrix A
A = np.array([[0, 1], [-2, -3]])
# Direct Computation
def matrix_exponential(A, n=10):
expA = np.eye(A.shape[0])
matrix_power = np.eye(A.shape[0])
factorial = 1
for i in range(1, n):
matrix_power = np.dot(matrix_power, A)
factorial *= i
expA += matrix_power / factorial
return expA
# Results
direct_result = matrix_exponential(A)
direct_result
Here's how the matrix exponential \( e^A \) for matrix \( A \) evolves with different truncations of the series:
Using 5 terms:
\[
e^A \approx \begin{bmatrix}
0.4167 & 0.0417 \\
-0.0833 & 0.2917 \\
\end{bmatrix}
\]
Using 10 terms:
\[
e^A \approx \begin{bmatrix}
0.6007 & 0.2328 \\
-0.4656 & -0.0977 \\
\end{bmatrix}
\]
Using 20 terms:
\[
e^A \approx \begin{bmatrix}
0.6004 & 0.2325 \\
-0.4651 & -0.0972 \\
\end{bmatrix}
\]
Observations:
- As we increase the number of terms in the series, the computed matrix \( e^A \) gets closer to the more accurate results we obtained earlier.
- The difference between the results from 10 terms and 20 terms is quite small, suggesting that the series is converging and additional terms contribute less to the final result.
- The matrix exponential computed with just 5 terms is significantly different, indicating that a truncation after only a few terms may not be sufficiently accurate for many matrices.
In practical applications, the choice of the number of terms depends on the matrix properties and the required accuracy. For most cases, the direct computation method would only be used for theoretical purposes or for small matrices, as more efficient algorithms (like the ones in scipy
) are available for general use.
Certainly! The eigenvalue approach to compute the matrix exponential is a powerful method, especially when the matrix in question is diagonalizable. Let's dive deeper into this technique.
Summary
Navigating the world of matrix exponentials is akin to traversing a landscape rich with mathematical intricacies and computational challenges. These exponentials, pivotal in numerous scientific and engineering domains, demand a robust understanding and efficient computation techniques. Through our exploration of the three primary methods - the foundational Direct Computation, the insightful Eigenvalue Approach, and the state-of-the-art scipy.linalg.expm
- we've unveiled the nuances and strengths each brings to the table. The Direct Computation method, while conceptually straightforward, serves as a gateway to appreciate the complexity of the problem. The Eigenvalue Approach, by capitalizing on the properties of diagonalizable matrices, offers a harmonious blend of theory and computation. Meanwhile, the SciPy method, backed by modern algorithms, stands as a testament to the advancements in computational mathematics, ensuring accuracy and efficiency.
As we stand at the crossroads of theory and application, it becomes evident that the choice of method hinges on the specific requirements of the task at hand, be it the matrix's nature, the desired accuracy, or computational resources. While the journey through matrix exponentials is filled with mathematical rigor, the destination promises a deeper understanding of systems, from quantum realms to macroscopic systems in control theory. It's a journey that underscores the beauty of mathematics and its profound impact on understanding and shaping the world around us.