How to Calculate LU Decomposition in Python
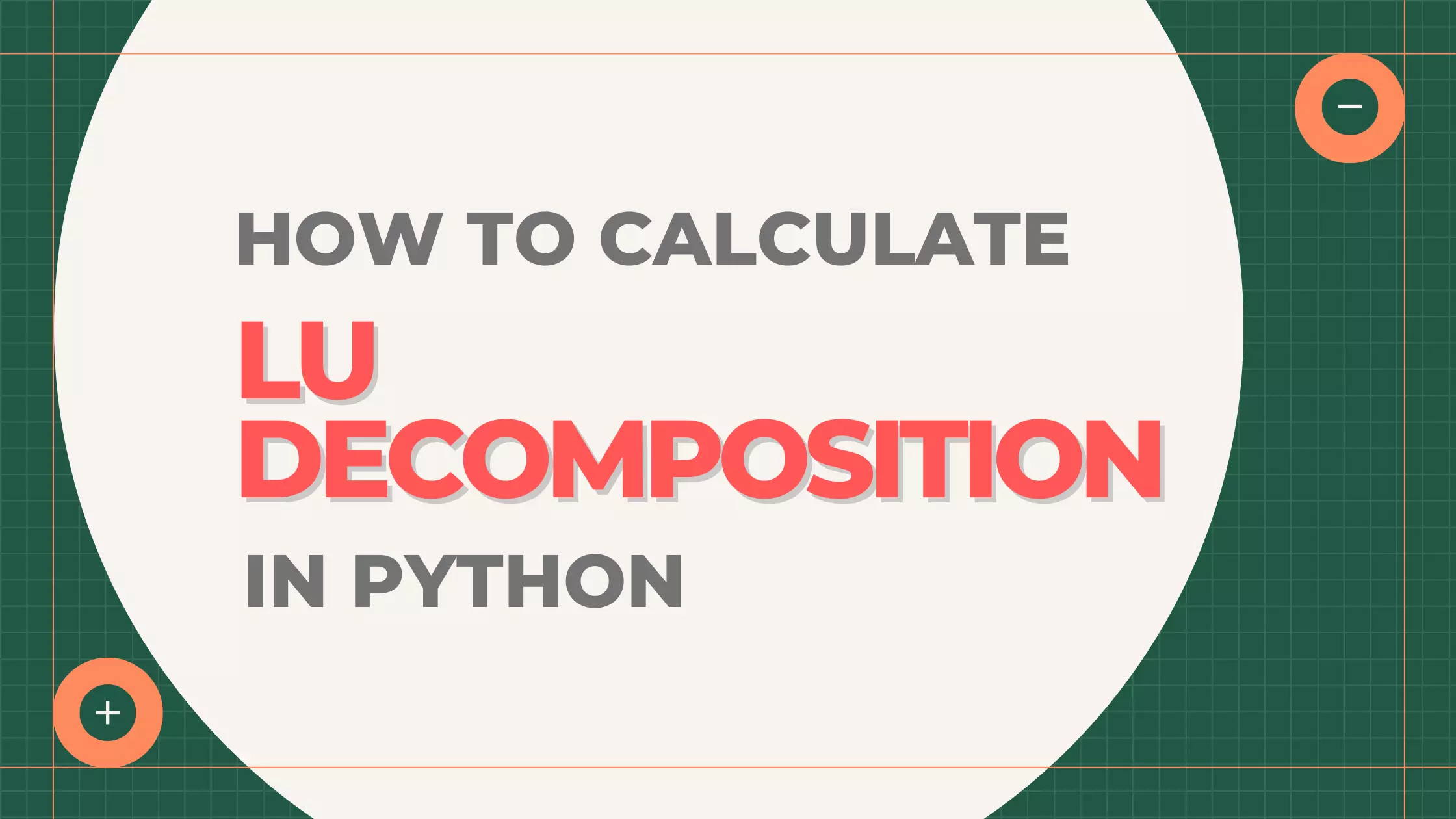
In the realm of numerical computing, it is often a necessity to find solutions to systems of linear equations. These are routinely encountered in fields like engineering, computer science, physics, and statistics. One way to solve these systems is through a method called LU decomposition. This blog post will explain how to compute LU decomposition using Python, the widely-used programming language known for its ease of use and extensive mathematical libraries.
What is LU Decomposition?
LU Decomposition, or factorization, is a method used in numerical linear algebra for solving linear equations, inverting matrices, and computing determinants. This technique essentially involves decomposing a matrix into the product of a lower triangular matrix \(\mathbf{L}\) and an upper triangular matrix \(\mathbf{U}\).
Mathematically, given a square matrix \(\mathbf{A}\), we seek matrices \(\mathbf{L}\) and \(\mathbf{U}\) such that:
\(\mathbf{A} = \mathbf{L} \mathbf{U}\)
where \(\mathbf{L}\) is a lower triangular matrix, and \(\mathbf{U}\) is an upper triangular matrix.
The motivation behind LU decomposition is the simplification of complex linear systems. By breaking down a problem into more manageable components, we can greatly reduce the computational complexity involved in solving large systems of equations.
The process is somewhat analogous to factoring integers: just as any composite number can be factored into prime numbers, any non-singular (invertible) matrix can be factored into a lower triangular and an upper triangular matrix. This fundamental insight allows us to solve complex linear systems in a systematic and computationally efficient manner.
Relation to Other Matrix Decompositions
Matrix decompositions, including LU, QR, Eigenvalue, and Singular Value Decomposition (SVD), are all strategies for simplifying matrices to make various numerical computations more manageable.
While LU decomposition involves breaking a matrix into lower and upper triangular matrices, QR decomposition involves an orthogonal and a right triangular matrix, and SVD consists of a product of orthogonal matrices and a diagonal matrix. Eigenvalue decomposition, on the other hand, uses diagonal and invertible matrices.
Despite these differences, they all share the same core principle of facilitating the solution of complex problems in numerical computing by simplifying the involved matrices.
Practical Applications of LU Decomposition
LU Decomposition is widely used in numerical analysis for solving a variety of problems. Here, we elaborate on some of its major applications:
1. Systems of Linear Equations:
This is perhaps the most common application of LU Decomposition. Linear systems are ubiquitous in scientific computing, and they usually take the form \(\mathbf{Ax} = \mathbf{b}\), where \(\mathbf{A}\) is a matrix of coefficients, \(\mathbf{x}\) is the vector of variables, and \(\mathbf{b}\) is the vector of numbers on the right side of the equations. If \(\mathbf{A}\) can be decomposed into \(\mathbf{L}\) and \(\mathbf{U}\), we can solve this system in two steps:
First, solve \(\mathbf{Ly} = \mathbf{b}\) for \(\mathbf{y}\) by forward substitution, and then solve \(\mathbf{Ux} = \mathbf{y}\) for \(\mathbf{x}\) by back substitution. This process is computationally efficient compared to directly solving the original system, especially for large systems.
2. Matrix Inversion:
LU Decomposition is useful in calculating the inverse of a matrix. If \(\mathbf{A}\) can be decomposed into \(\mathbf{L}\) and \(\mathbf{U}\), the inverse \(\mathbf{A}^{-1}\) can be computed by solving \(\mathbf{Ax} = \mathbf{I}\), where \(\mathbf{I}\) is the identity matrix, for each column of the identity matrix. This means that the inverse of a matrix can be found without having to compute determinants, which can be computationally expensive for large matrices.
3. Determinant Calculation:
The determinant of a matrix can be calculated from its LU Decomposition. Since the determinant of a triangular matrix is simply the product of its diagonal entries, the determinant of \(\mathbf{A}\) can be found by multiplying the diagonal entries of \(\mathbf{L}\) and \(\mathbf{U}\).
4. Eigenvalues and Eigenvectors:
LU Decomposition is also used in iterative methods for finding eigenvalues and eigenvectors. Given an estimate of an eigenvalue, LU Decomposition can be used to deflate the original matrix, thus facilitating the computation of other eigenvalues.
5. Data Analysis and Machine Learning:
In data analysis and machine learning, solving linear systems and matrix inversion are common tasks, particularly in methods like linear regression, principal component analysis (PCA), and the computation of similarity measures.
In conclusion, LU Decomposition is a fundamental operation in scientific computing and numerical analysis. By decomposing a complex system into simpler parts, LU Decomposition can make difficult mathematical problems much easier to handle.
Essential Libraries for LU Decomposition in Python
Usually, we first resort to NumPy as a fundamental package for numerical computation in Python. It provides support for arrays, matrices, and essential mathematical functions. However, for LU decomposition, there is no support in NumPy.
Luckily, SciPy is a powerful library for scientific computation and it provides the scipy.linalg
module, which includes an LU decomposition function.
Calculating LU Decomposition Using SciPy
Using SciPy's linalg.lu
function, we can easily perform LU decomposition:
import numpy as np
from scipy.linalg import lu
# Define a square matrix A
A = np.array([[4, 3], [6, 3]])
# Perform the LU Decomposition
L, U = lu(A, True)
print("L: \n", L)
print("U: \n", U)
The output L and U are the lower and upper triangular matrices respectively. The second tells the LU decomposition algorithm to permute the L matrix. Otherwise, we would have to collect the permutation matrix also P, L, U = lu(A)
.
How to verify the LU Decomposition
You can then verify the decomposition by multiplying L and U, and checking if it equals the original matrix A, just like in the case of NumPy.
# Multiply L and U
LU = np.dot(L, U)
print("LU: \n", LU)
print("A: \n", A)
# Check if A and LU are equal within a tolerance
if np.allclose(A, LU):
print("LU decomposition is valid")
else:
print("LU decomposition is not valid")
Again, the np.allclose
function is used to check whether the LU decomposition is correct.
Implementing LU Decomposition without External Packages
In our custom Python implementation of LU decomposition, we're essentially following the Doolittle Algorithm. This algorithm describes how to solve for the \(\mathbf{L}\) and \(\mathbf{U}\) matrices step-by-step.
First, we initialize two matrices \(\mathbf{L}\) (lower triangular) and \(\mathbf{U}\) (upper triangular) with sizes equal to the input square matrix \(\mathbf{A}\), both filled with zeroes. The matrix \(\mathbf{L}\) has diagonal elements set to 1.
The algorithm uses two nested loops to iterate over each element in the \(\mathbf{A}\) matrix. The outer loop \(i\) represents the current pivot row, while the inner loop \(j\) is for the remaining rows (from \(i\) to \(n\)).
For each pivot \(i\), the algorithm first calculates the (\mathbf{U}\) matrix and then the \(\mathbf{L}\) matrix using the following formulas:
Calculating U:
For every row \(i\) and for each column \(j\) from \(i\) to \(n\), we compute the element in the \(\mathbf{U}\) matrix as:
\[ U_{ij} = A_{ij} - \sum_{k=1}^{i-1} L_{ik}U_{kj} \]
Here, \(U_{ij}\) is the element in the \(\mathbf{U}\) matrix at the \(i^{\text{th}}\) row and \(j^\text{th}\) column, and \(A_{ij}\) is the corresponding element in the original matrix. The summation part of the equation involves elements from \(\mathbf{L}\) and \(\mathbf{U}\) that have already been computed.
Calculating L:
We then compute the elements of the \(\mathbf{L}\) matrix using a similar method:
\[ L_{ji} = \frac{1}{U_{ii}} (A_{ji} - \sum_{k=1}^{i-1} L_{jk}U_{ki}) \]
The computation for \(L_{ji}\) is done for each row \(j\) from \(i\) to \(n\). The difference here is that we divide the result by \(U_{ii}\), the diagonal element in the \(\mathbf{U}\) matrix.
Following this procedure, we eventually fill up the \(\mathbf{L}\) and \(\mathbf{U}\) matrices. These two matrices are then the LU decomposition of the original matrix \(\mathbf{A}\).
One crucial thing to note is that not all matrices can be decomposed using the Doolittle Algorithm. If during the process any diagonal element \(U_{ii}\) is zero (which we need for the division when calculating the L matrix), the algorithm fails. In such cases, a variation of the algorithm that includes partial pivoting is used.
In Python, we can implement LU Decomposition from scratch. Below is a simple implementation:
def lu_decomposition(A):
# Initialize L and U matrices
n = len(A)
L = [[0]*n for _ in range(n)]
U = [[0]*n for _ in range(n)]
# Compute L and U
for i in range(n):
for j in range(i, n): # U
U[i][j] = A[i][j] - sum(L[i][k]*U[k][j] for k in range(i))
for j in range(i, n): # L
if i == j:
L[i][i] = 1 # Diagonal as 1
else:
L[j][i] = (A[j][i] - sum(L[j][k]*U[k][i] for k in range(i))) / U[i][i]
return L, U
L, U = lu_decomposition(A)
print("L: \n", L)
print("U: \n", U)
References to LU Decomposition
To delve deeper into the theoretical underpinnings of LU decomposition, consider the following references:
- Matrix Analysis and Applied Linear Algebra by Carl D. Meyer - A comprehensive resource on matrix computations, covering topics including LU Decomposition.
- Numerical Linear Algebra by Lloyd N. Trefethen, David Bau III - This book offers a fresh approach to numerical linear algebra, including a detailed look at LU Decomposition.
Conclusion
LU Decomposition is a cornerstone in numerical analysis, enabling efficient solutions to systems of linear equations, matrix inversions, and determinants computation. By unpacking complex systems into simpler components, LU Decomposition offers an approachable method for tackling advanced mathematical problems. We explored this concept in depth, detailing its intuition, relationship to other matrix decompositions, and practical applications. We also examined how Python's powerful libraries, like SciPy, facilitate LU Decomposition, and demonstrated how to implement it without relying on external packages.
However, it's worth noting that LU Decomposition isn't a one-size-fits-all solution. Other techniques such as QR or Cholesky Decomposition might be more suitable depending on your specific problem. Also, the LU decomposition might not always exist for all matrices and may not be unique. Understanding the underlying mathematics, including the Doolittle algorithm, is invaluable for troubleshooting and optimizing performance. Thus, while Python libraries simplify LU Decomposition, a comprehensive understanding of its principles greatly enhances your ability to solve numerical problems effectively.