Mastering DataFrame Comparisons in Pandas: Techniques & Tips
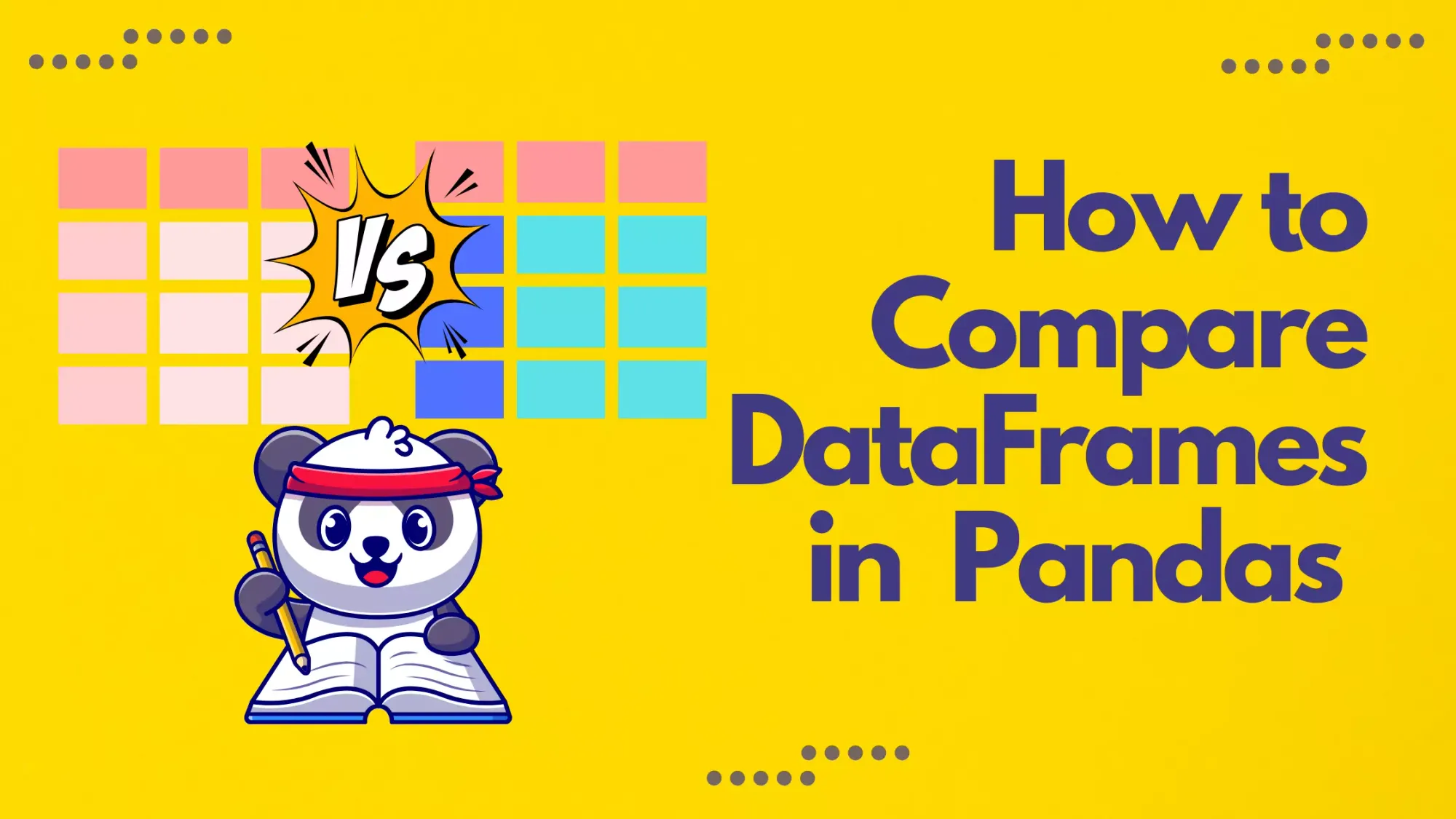
For data scientists and analysts, the ability to handle, analyze, and interpret data is paramount. A significant portion of these operations is performed using DataFrames, a 2-dimensional labeled data structure that is akin to tables in databases, Excel spreadsheets, or even statistical data sets. Pandas, a Python-based data analysis toolkit, provides an efficient and user-friendly way to manipulate these DataFrames. However as data operations scale and become more complex, professionals often encounter scenarios where they must compare two or more DataFrames. Whether it's to verify data consistency, spot anomalies, or simply align data sets, effective comparison techniques can save both time and effort.
Understanding how to perform these comparisons in Pandas is, therefore, an essential skill for any data enthusiast. Whether you're a seasoned data scientist, an analyst starting your journey, or a developer looking to refine data processing skills, this guide offers a deep dive into various techniques for DataFrame comparison. By exploring the gamut of these methods, from basic element-wise checks to intricate merging strategies, you'll gain the confidence to tackle any data challenge thrown your way.
Basic Comparison with equals()
in Pandas
In the world of data analysis, determining if two DataFrames are identical is a fundamental task. This is where the equals()
method in Pandas becomes invaluable. It allows users to check whether two DataFrames are the same in terms of shape (i.e., same number of rows and columns) and elements.
Syntax Overview:
DataFrame.equals(other)
other
: The other DataFrame to be compared with.
If both DataFrames are identical in terms of shape and elements, the method returns True
; otherwise, it returns False
.
For a comprehensive look into this function and its underlying mechanics, the official Pandas documentation offers in-depth insights.
Example 1
Suppose we have two DataFrames df1
and df2
:
A | B | |
---|---|---|
0 | 1 | 4 |
1 | 2 | 5 |
2 | 3 | 6 |
Comparing df1
and df2
import pandas as pd
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
print(df1.equals(df2))
Output:
True
Example 2
Now, suppose df3
has a slight variation:
A | B | |
---|---|---|
0 | 1 | 4 |
1 | 2 | 5 |
2 | 4 | 6 |
Comparing df1
and df3
:
df3 = pd.DataFrame({'A': [1, 2, 4], 'B': [4, 5, 6]})
print(df1.equals(df3))
Output:
False
In this case, even though both DataFrames have the same shape, their elements are not entirely identical.
When is equals()
Beneficial?
- Simplicity and Speed: For a quick and straightforward yes-or-no answer about whether two DataFrames are identical, it's hard to beat
equals()
. - Exact Matching: If you want to ensure every element, column, and row position matches between the two DataFrames without any nuanced control over the comparison,
equals()
is your function. - Memory Efficiency: Since it returns just a boolean, it's more memory-efficient than methods that return new DataFrames or subsets of DataFrames.
Key Takeaway: The equals()
method provides a concise way to check for DataFrame equality. However, it's worth noting that it's strict in its comparison – both shape and elements must match perfectly. For more flexible or detailed differences, other methods in Pandas might be more suitable.
Element-wise Comparison with compare()
in Pandas
While the equals()
method lets us know if two DataFrames are identical, there are scenarios where we need a more detailed breakdown of differences between DataFrames. The compare()
method, introduced in Pandas 1.1.0, offers this granularity, enabling an element-wise comparison to identify where two DataFrames differ.
Syntax Overview:
DataFrame.compare(other, align_axis='columns')
other
: The other DataFrame to be compared with.align_axis
: {‘index’, ‘columns’}, default ‘columns’. Determine which axis to align the comparison on.
The result of compare()
is a new DataFrame that shows the differences side by side. For a complete understanding of the parameters and options, you can refer to the official Pandas documentation.
Example 1:
Given two DataFrames df1
and df4
:
df1
A | B | |
---|---|---|
0 | 1 | 4 |
1 | 2 | 5 |
2 | 3 | 6 |
df4
A | B | |
---|---|---|
0 | 1 | 4 |
1 | 2 | 7 |
2 | 3 | 8 |
Let's find the differences:
df4 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 7, 8]})
diff = df1.compare(df4)
print(diff)
Output:
B
self other
1 5 7
2 6 8
Here, the result showcases the differences between df1
and df4
are in the 'B' column for rows 1 and 2.
Example 2:
Let's have another set of DataFrames, df1
(from the previous example) and df5
:
df5
A | B | |
---|---|---|
0 | 1 | 4 |
1 | 3 | 5 |
2 | 3 | 6 |
Comparing df1
and df5
:
df5 = pd.DataFrame({'A': [1, 3, 3], 'B': [4, 5, 6]})
diff = df1.compare(df5)
print(diff)
Output:
A
self other
1 2 3
The difference is in the 'A' column of row 1.
When is compare()
Beneficial Over Others?
- Detailed Diffs: If you need a detailed breakdown of where exactly two DataFrames differ,
compare()
is tailor-made for this purpose. - Consistency Checks: In scenarios where you've applied some operations to a DataFrame and want to validate that only specific parts of the DataFrame have changed (and how they've changed),
compare()
provides a clear view. - Visualization: The output format of
compare()
is particularly conducive to visualizing differences, making it easier for human inspection. - Handling Aligned DataFrames: It assumes that the two DataFrames are aligned (i.e., they have the same shape). This is beneficial when comparing two DataFrames that should have the same structure, like before-and-after scenarios.
Key Takeaway: The compare()
method is a valuable tool when a detailed comparison is desired. It allows for quick visualization of differences and can be especially useful in data cleaning and validation processes where spotting discrepancies is essential.
Using isin()
for Row-wise Comparison
The isin()
method in Pandas is another powerful tool for comparisons, but its primary purpose diverges slightly from the previously discussed methods. While equals()
and compare()
focus on DataFrames as a whole or element-wise differences, isin()
is used to filter data frames. It is mainly applied to a Series to check which elements in the series exist in a list. However, when used creatively, it can be leveraged for row-wise comparisons between DataFrames.
Syntax Overview:
DataFrame.isin(values)
values
: Iterable, Series, DataFrame or dictionary. The result will only be true at locations which are contained invalues
.
You can dig deeper into this method by referring to the official Pandas documentation.
Example 1:
Suppose we have two DataFrames df1
and df6
:
df1
A | B | |
---|---|---|
0 | 1 | 4 |
1 | 2 | 5 |
2 | 3 | 6 |
df6
A | B | |
---|---|---|
0 | 1 | 7 |
1 | 3 | 5 |
2 | 2 | 8 |
To check if rows in df1
exist in df6
:
print(df1.isin(df6.to_dict(orient='list')))
Output:
A B
0 True False
1 True True
2 True False
Example 2:
Given df1
and another DataFrame df7
:
df7
A | B | |
---|---|---|
0 | 4 | 7 |
1 | 5 | 8 |
2 | 6 | 9 |
Comparing df1
and df7
:
print(df1.isin(df7.to_dict(orient='list')))
Output:
A B
0 False False
1 False False
2 True False
Only the 'A' column value of row 2 in df1
matches a value in df7
.
When is isin()
Beneficial?
- Filtering & Masking: If you have a list or another DataFrame and you want to filter rows based on values from that list/DataFrame,
isin()
is the way to go. - Row Existence: When the objective is to identify if certain rows (or elements) exist in another DataFrame.
- Flexibility: Can be used both on a Series or DataFrame level, which gives it versatility in different scenarios.
- Non-aligned DataFrames: When the two DataFrames are not aligned, i.e., the rows you want to compare are not in the same order.
Key Takeaway: While isin()
is not specifically designed for comparison like equals()
or compare()
, it's a versatile method for specific scenarios, especially for row-wise existence checks and filtering. Understanding its strengths can make certain tasks much more straightforward.
Advanced Comparisons with merge()
in Pandas
Pandas’ merge()
function offers a powerful way to combine DataFrames, akin to SQL joins. While its primary use case is to combine datasets based on common columns or indices, it can be ingeniously applied for comparisons, particularly when identifying overlapping or unique rows between DataFrames.
Syntax Overview:
DataFrame.merge(right, how='inner', on=None, left_on=None, right_on=None, left_index=False, right_index=False)
right
: DataFrame to merge with.how
: Type of merge to be performed. Includes 'left', 'right', 'outer', and 'inner'.on
: Columns (names) to join on.- And other parameters for more advanced merging.
For an in-depth look at all available parameters, the official Pandas documentation offers comprehensive guidance.
Example 1: Finding common rows between two DataFrames.
Given two DataFrames df1
and df8
:
df1
A | B | |
---|---|---|
0 | 1 | 4 |
1 | 2 | 5 |
2 | 3 | 6 |
df8
A | B | |
---|---|---|
0 | 1 | 4 |
1 | 3 | 6 |
2 | 4 | 7 |
Finding overlapping rows:
common_rows = df1.merge(df8, how='inner')
print(common_rows)
Output:
A | B | |
---|---|---|
0 | 1 | 4 |
1 | 3 | 6 |
Example 2: Identifying rows in df1
that are not present in df8
.
Using the same DataFrames from the previous example:
unique_df1_rows = df1.merge(df8, how='left', indicator=True).query('_merge == "left_only"').drop('_merge', axis=1)
print(unique_df1_rows)
Output:
A | B | |
---|---|---|
1 | 2 | 5 |
When is merge()
Beneficial?
- Relational Dataset Operations:
merge()
is ideal when you have relational data and you want to combine datasets based on certain keys. - Identifying Unique and Common Rows: If you want to find rows that are unique to one DataFrame or common between two,
merge()
with the right parameters can make this process very intuitive. - Multiple Column Conditions: When comparisons need to be based on multiple columns,
merge()
is more efficient than manual loops or conditional checks. - Preserving Information: Unlike some other comparison methods, merging can maintain other columns' information to give context about the differences or similarities.
- Versatility: Just like SQL joins, merge offers versatility with inner, left, right, and outer joins, which can be tailored for various comparison scenarios.
Key Takeaway: The merge()
function, while primarily used for joining operations, is a potent tool for comparison tasks, especially in scenarios where DataFrames have relational aspects. Its ability to quickly identify overlaps and discrepancies makes it invaluable in a data analyst's toolkit. However, it's essential to remember that merge()
is computationally more expensive, so for large datasets, considerations on performance need to be taken into account.
By merging on all columns and checking if the resultant DataFrame has the same length as the originals, you can deduce if the DataFrames are the same.
merged = pd.merge(df1, df3, how='outer', indicator=True)
diff_rows = merged[merged['_merge'] != 'both']
diff_rows
contains the differing rows between the DataFrames.
Utilizing the assert_frame_equal
Function
the assert_frame_equal
is a function provided by Pandas primarily for testing purposes. It allows you to assert that two DataFrames are equal, meaning they have the same shape and elements. If they are not equal, this function raises an assertion error, which is helpful in debugging or during unit tests to ensure that the data manipulations yield the expected results.
Syntax Overview:
pandas.testing.assert_frame_equal(left, right, check_dtype=True, check_index=True, check_column_type=True, check_frame_type=True, check_less_precise=False, check_exact=False, check_datetimelike_compat=False, check_categorical=True, check_category_order=True, check_freq=True, check_flags=True, check_index_type=True, check_column_index=False, check_datetimelike_dtype=True, check_categorical_dtype=True, check_category=True, check_index_type=False, check_frame_type=False, check_like=False, check_exact=False, check_datetimelike_compat=False, check_categorical=True, check_category_order=True, check_freq=True, check_index=True)
left
,right
: The two DataFrames to compare.check_dtype
,check_index
, etc.: Various parameters to control the types of checks made during the comparison.
The official Pandas documentation provides an in-depth understanding of all available parameters.
Example 1: Simple exact match.
Given two identical DataFrames, df1
and df9
:
df1
and df9
A | B | |
---|---|---|
0 | 1 | 4 |
1 | 2 | 5 |
2 | 3 | 6 |
Testing their equality:
from pandas.testing import assert_frame_equal
try:
assert_frame_equal(df1, df9)
print("DataFrames are equal!")
except AssertionError:
print("DataFrames are not equal!")
Output:
DataFrames are equal!
Example 2: Discrepancy in data.
Given df1
and another DataFrame df10
:
df10
A | B | |
---|---|---|
0 | 1 | 4 |
1 | 2 | 5 |
2 | 3 | 7 |
Comparing df1
and df10
:
try:
assert_frame_equal(df1, df10)
print("DataFrames are equal!")
except AssertionError:
print("DataFrames are not equal!")
Output:
DataFrames are not equal!
When is assert_frame_equal
Beneficial?
- Unit Testing: It's designed mainly for testing. If you're writing unit tests for data processing functions, it provides an easy way to check if your function's output matches the expected result.
- Debugging: Helpful during the debugging process, as the raised assertion errors can provide insight into where and how the data differs.
- Strict Comparisons: It checks both data and metadata (like dtypes). If you want to be sure not only that two DataFrames have the same data but also that they have the same structure, it's invaluable.
- Customizable Checks: With a variety of parameters available, you can customize what gets checked, such as ignoring dtypes or indexes.
Key Takeaway: assert_frame_equal
isn't typically used for general DataFrame comparisons in data analysis workflows but shines in development and testing environments. When ensuring exactitude and conformity is a priority, especially in an automated testing scenario, this function proves indispensable.
What is the most efficient way to compare DataFrames
Comparing DataFrames efficiently depends on what you specifically want to achieve from the comparison.
Checking If DataFrames are Identical:
- Method:
equals()
- Why: It quickly gives a boolean answer — either the DataFrames are identical or they're not. It's straightforward and doesn't create a new DataFrame.
Identifying Differences Between DataFrames Element-wise:
- Method:
compare()
- Why: It provides a concise DataFrame showing only the cells that are different between two DataFrames.
Finding Rows in One DataFrame That Are Not in Another DataFrame:
- Method:
merge()
with theindicator=True
option. - Why: It's similar to a SQL left outer join and can quickly identify rows that don't have counterparts in another DataFrame. Using the indicator will add a column showing which DataFrame(s) each row comes from.
For Testing Purposes:
- Method:
pandas.testing.assert_frame_equal()
- Why: Designed primarily for unit testing, it raises an AssertionError if two DataFrames are not identical, making it easy to use within testing frameworks.
Comparing Specific Columns:
- Method: Direct Boolean Indexing
- Why: If you only care about specific columns, direct comparison is efficient.
is_equal = df1['column_name'] == df2['column_name']
Comparing Large DataFrames for Approximate Matching:
- Method: Sampling & then using
equals()
- Why: Sometimes, for extremely large datasets, it may be impractical to compare every single row, especially if you're looking for a quick, approximate answer. In such cases, you can sample a fraction of the DataFrame and compare the samples.
Performance Considerations:
- If you have DataFrames with multi-level indices, ensure they're lexsorted; this can improve performance in various operations.
- For large datasets, consider using tools like Dask which parallelizes operations and works seamlessly with Pandas-like syntax.
The efficiency of a comparison not only depends on the size of the DataFrames but also on the nature of the comparison you need to perform and the hardware on which you're operating.
Conclusion
Comparing DataFrames in Pandas goes well beyond a surface-level search for exact matches. As we've explored, the intricacies of data comparison require a myriad of techniques, each tailored to specific scenarios and objectives. Some methods like equals()
offer quick, all-encompassing checks, while others like compare()
and merge()
provide a more granular perspective. But beyond just the techniques, understanding the 'why' and 'when' of using them is the mark of a seasoned analyst. The context in which you're comparing data, the scale of the operation, and the desired outcome all influence the choice of method.
It's this flexibility and range of options that make Pandas an invaluable tool for data professionals. Whether it's ensuring data consistency after a major migration, validating data after a cleansing operation, or simply wanting to find the nuances between two seemingly similar data sets, mastering DataFrame comparison techniques equips you with a sharper lens to view and process data. And as with any tool or technique, consistent practice and real-world application will refine your skills further.
Always remember to keep the official Pandas documentation handy, for it's an ever-evolving treasure trove of insights and updates.
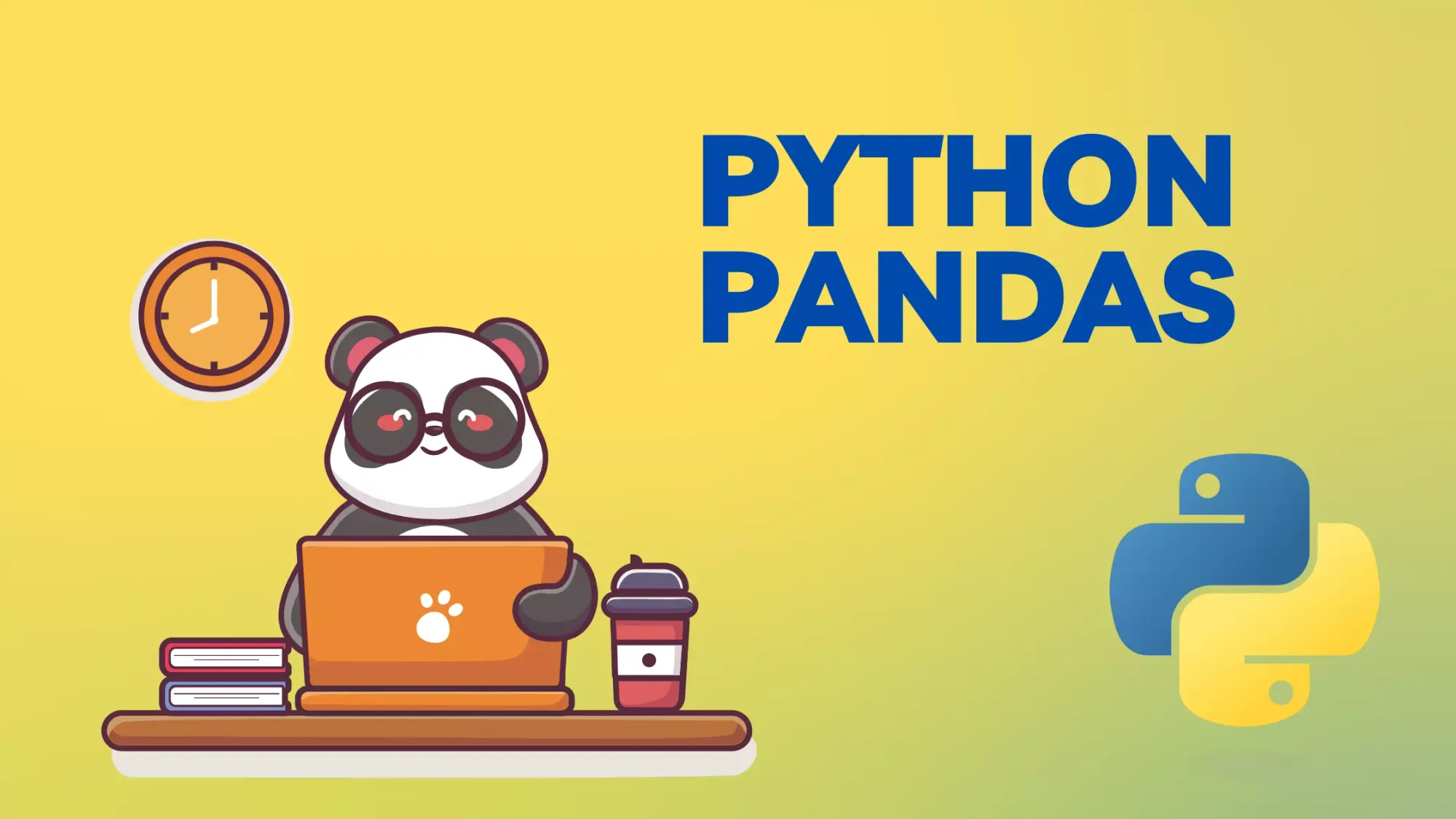