How to Compare Data Frames in R
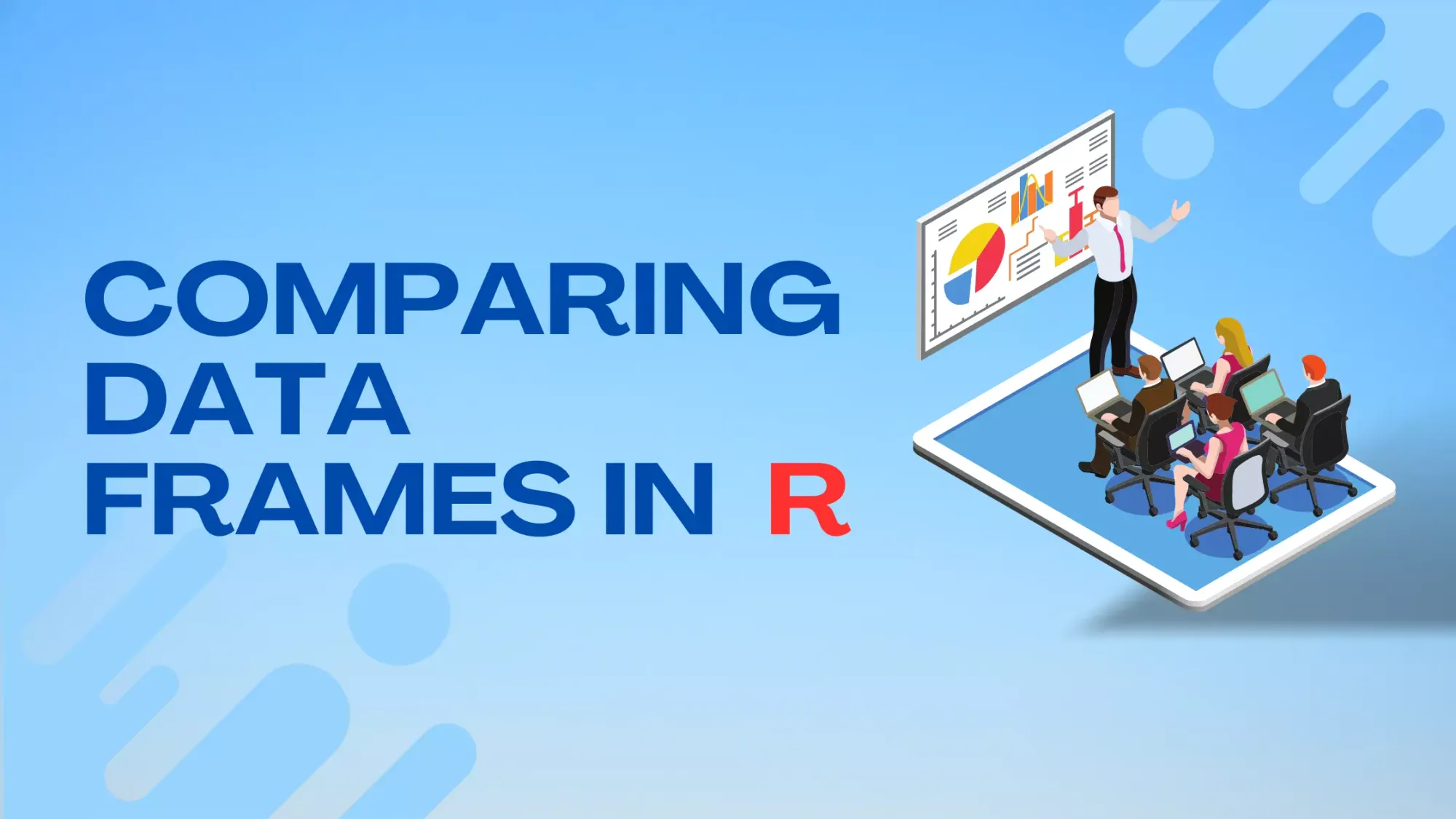
The ability to efficiently compare data frames is paramount for data analysts. Data frames, being the primary data structure for storing data tables in R, often need to be compared for tasks such as data cleaning, validation, and analysis. Whether it's to identify changes over time, ensure data consistency, or detect anomalies, understanding the nuances of data frame comparison is crucial for any data scientist or analyst working with R.
Yet, like many operations in R, there's no one-size-fits-all solution. Depending on the specific task and the nature of your data, different methods might be more suitable. This guide aims to demystify the various techniques available for comparing data frames in R. We'll walk through the basic approaches, delve into more advanced methods, and even touch upon external libraries that can supercharge this process. So, whether you're a novice R user or a seasoned expert, there's something in this guide for you.
Basic DataFrame Comparison in R
When working with data frames in R, it's common to need to compare them. This can be done to check if they are identical or to find differences in their content. R provides several built-in functions that allow for efficient comparison of data frames. Here, we'll explore some of the foundational methods.
Using identical()
The identical()
function is a simple yet powerful tool in base R that checks if two objects are exactly the same, including their attributes.
Example 1:
Let's start with two data frames that are identical:
df1 <- data.frame(A = c(1, 2), B = c(3, 4))
df2 <- data.frame(A = c(1, 2), B = c(3, 4))
identical(df1, df2)
Result: TRUE
Example 2:
However, if there's even a slight difference, such as a change in one value, the function will return FALSE
.
df3 <- data.frame(A = c(1, 2), B = c(3, 5))
identical(df1, df3)
Result: FALSE
For more on identical()
, refer to the official R documentation.
Using all.equal()
Another useful function for comparing data frames is all.equal()
. Unlike identical()
, all.equal()
provides more flexibility by not considering minor differences like attribute order or row names as long as the content is the same. Additionally, it gives descriptive messages about the differences.
Example 1:
When the data frames are the same:
df4 <- data.frame(A = c(1, 2), B = c(3, 4), row.names = c("row1", "row2"))
df5 <- data.frame(A = c(1, 2), B = c(3, 4), row.names = c("rowA", "rowB"))
all.equal(df4, df5)
Result:
Attributes: < Component \"row.names\": 2 string mismatches >
Example 2:
If there are differences, all.equal()
will describe them:
df6 <- data.frame(A = c(1, 2), B = c(3, 5))
all.equal(df4, df6)
Result:
[1] "Attributes: < Component \"row.names\": Modes: character, numeric >"
[2] "Attributes: < Component \"row.names\": target is character, current is numeric >"
[3] "Component \"B\": Mean relative difference: 0.25"
For a deeper dive into all.equal()
, please consult the official R documentation.
While identical()
offers a strict comparison, all.equal()
is more forgiving and descriptive. Depending on the specific requirements of your task, you might find one more appropriate than the other. Always consider the nature of your data and the context of your comparison when choosing a method.
Row and Column Wise Comparison
In many situations, comparing entire data frames might not be necessary. Instead, you may be interested in comparing specific rows or columns. R offers great flexibility in this regard, allowing for granular comparisons that can be tailored to specific needs. Here, we'll explore methods to compare data frames on a row-by-row or column-by-column basis.
Row-wise Comparison
When it comes to row-wise comparison, you can compare specific rows between two data frames by indexing.
Example 1:
Comparing the first row of two identical data frames:
df1 <- data.frame(A = c(1, 2), B = c(3, 4))
df2 <- data.frame(A = c(1, 2), B = c(3, 4))
all(df1[1, ] == df2[1, ])
Result: TRUE
Example 2:
Comparing the first row of two different data frames:
df3 <- data.frame(A = c(1, 2), B = c(5, 4))
all(df1[1, ] == df3[1, ])
Result: FALSE
The function all()
is used here to check if all elements of the logical comparison are TRUE
. More details about the all()
function can be found in the official R documentation.
Column-wise Comparison
For comparing specific columns between two data frames, you can use the $
operator or the double square bracket [[
to extract the column and then compare.
Example 1:
Comparing the "A" column of two identical data frames:
all(df1$A == df2$A)
Result: TRUE
Example 2:
Comparing the "A" column of two different data frames:
all(df1$A == df3$A)
Result: TRUE
This result is TRUE
because the "A" column in both df1
and df3
is identical, even though the "B" column differs.
The column extraction can also be done using the double square bracket:
all(df1[["A"]] == df3[["A"]])
Result: TRUE
For more on column extraction and indexing in data frames, refer to the official R documentation.
Row and column-wise comparisons are essential tools when working with data frames in R. By understanding how to effectively compare specific parts of your data, you can pinpoint differences and anomalies with greater precision, making your data analysis tasks more efficient and accurate.
Using External Libraries for DataFrame Comparison
While base R offers an array of tools for comparing data frames, the expansive R ecosystem provides numerous external packages that can aid in more intricate or specialized comparisons. These libraries often simplify the comparison process and provide enhanced insights into data frame differences. Here, we'll delve into some popular external libraries and demonstrate their capabilities.
Using dplyr
The dplyr
package, part of the tidyverse, is one of the most widely used packages for data manipulation in R. Among its numerous functions, dplyr
provides the all_equal()
function for data frame comparisons.
Example 1:
Comparing identical data frames:
library(dplyr)
df1 <- data.frame(A = c(1, 2), B = c(3, 4))
df2 <- data.frame(A = c(1, 2), B = c(3, 4))
all_equal(df1, df2)
Expected Result: TRUE
Example 2:
For data frames with differences, all_equal()
offers a descriptive message:
df3 <- data.frame(A = c(1, 3), B = c(3, 4))
all_equal(df1, df3)
Result: "Rows in x but not in y: 2\n- Rows in y but not in x: 2"
For more on the capabilities of dplyr
and its comparison functions, refer to the official dplyr
documentation.
Leveraging external libraries can drastically enhance the efficiency and depth of data frame comparisons in R. While base R functions are powerful, these specialized libraries offer refined tools tailored for specific comparison needs, especially in complex projects or large-scale analyses. It's always beneficial to be acquainted with a mix of both base and external tools to choose the most apt method for a given task.
Best Practices and Tips for Comparing Data Frames
Comparing data frames is more than just executing a function. It requires a holistic understanding of your data, the context, and the specific requirements of your analysis. As with many operations in R, there are caveats and intricacies that, if overlooked, can lead to incorrect conclusions. Here, we'll dive deeper into some best practices and tips to ensure that your data frame comparisons are both accurate and meaningful.
1. Ensure Matching Dimensions
Before diving into the actual comparison, it's a good practice to ensure that the data frames you're comparing have matching dimensions. This quick check can save computational time and prevent potential errors.
Example:
dim(df1) == dim(df2)
Result: TRUE
if the dimensions match, otherwise FALSE
.
The dim()
function returns the dimensions of an object. For more details, refer to the official documentation.
2. Verify Data Types
Mismatched data types can lead to unexpected comparison results. Always ensure that corresponding columns in the data frames being compared have the same data type.
Example:
Comparing a character column with a factor column:
df1 <- data.frame(A = c("apple", "banana"))
df2 <- data.frame(A = factor(c("apple", "banana")))
identical(df1$A, df2$A)
Result: FALSE
because one is character and the other is a factor.
To inspect data structures and types, use the str()
function. For more, see the official documentation.
3. Address Precision Issues
When dealing with floating-point numbers, be cautious of precision issues. Direct comparison might not yield expected results due to the way computers represent floating-point numbers.
Example:
x <- 0.3 - 0.1
y <- 0.2
identical(x, y)
Result: FALSE
due to floating-point precision issues.
In such cases, consider using functions like all.equal()
that allow for a certain tolerance.
4. Sort Data Before Comparison
If row order isn't crucial for your analysis, consider sorting data frames by key columns before comparing. This ensures rows are aligned correctly, making the comparison meaningful.
Example:
df1 <- data.frame(A = c(2, 1), B = c(4, 3))
df2 <- data.frame(A = c(1, 2), B = c(3, 4))
identical(df1, df2)
df1[order(df1$A), ] == df2[order(df2$A), ]
Result:
[1] FALSE
A B
2 TRUE TRUE
1 TRUE TRUE
Here, the direct comparison is FALSE
but after sorting by column "A", the data frames are identical.
While R offers robust tools for data comparison, the onus is on the user to ensure that the comparisons are meaningful and accurate. By following best practices and being cognizant of potential pitfalls, you can make more informed decisions and produce more reliable results in your data analyses. Always remember to refer back to official documentation to understand the nuances and intricacies of the functions you use.
Conclusion
Understanding how to effectively compare data frames in R is a key skill that can greatly aid in this endeavor. As we've explored in this guide, R offers a plethora of techniques, each tailored for specific situations and requirements. Whether you're using base R functions or leveraging the power of external libraries, the right tools are at your disposal. But as always, the tool is only as good as the craftsman. It's vital to comprehend the underlying principles of these methods to apply them effectively.
As you continue your journey in R and data science, let this guide serve as a foundational reference. Remember to always remain curious and continue exploring. The R community is vibrant and constantly evolving, with new methods and packages emerging regularly. Always refer back to official documentation for the most recent advancements and best practices. By staying informed and honing your skills, you'll be well-equipped to handle any data comparison challenge that comes your way.
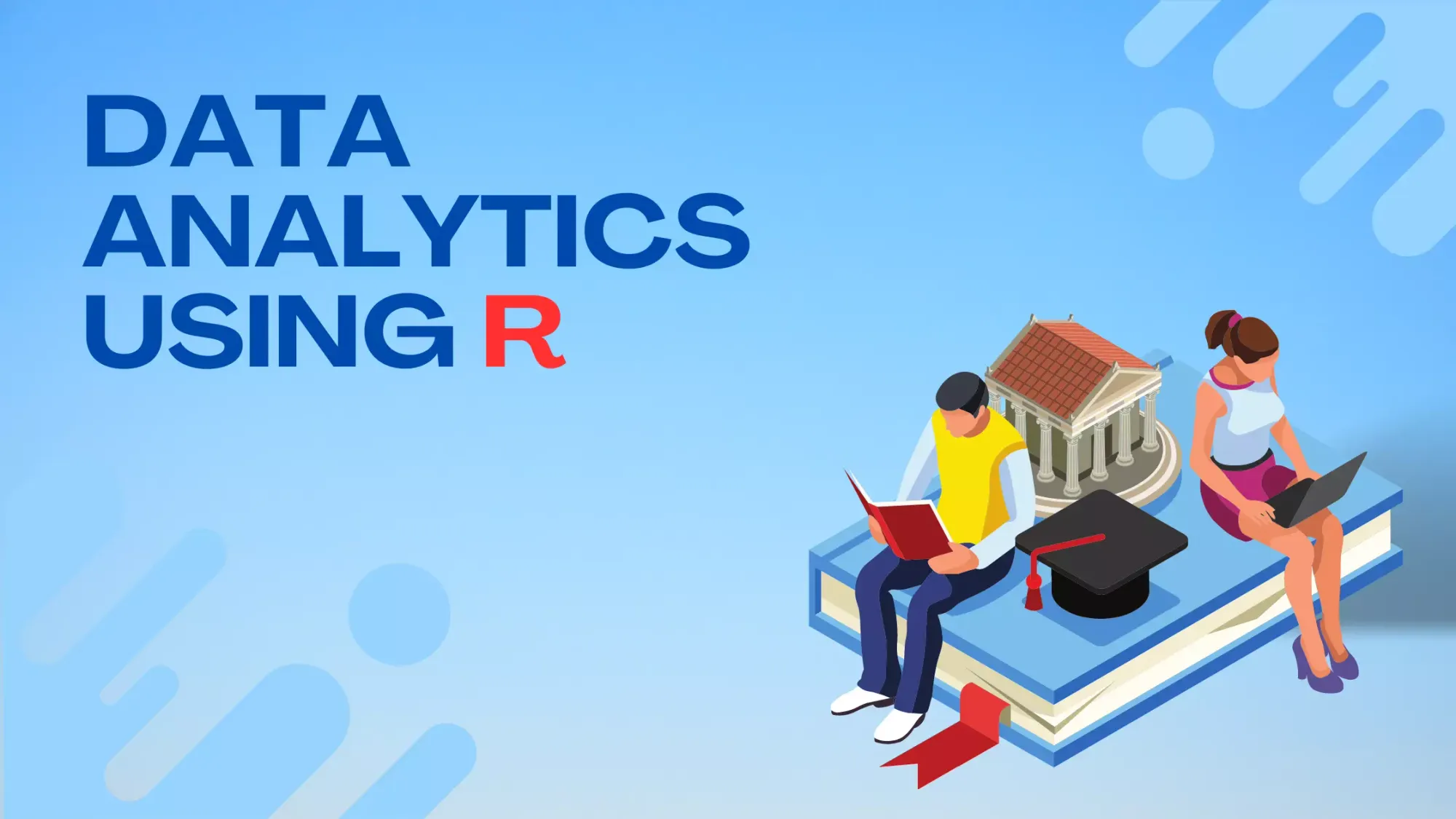