3 Techniques for Calculating Percentiles in R
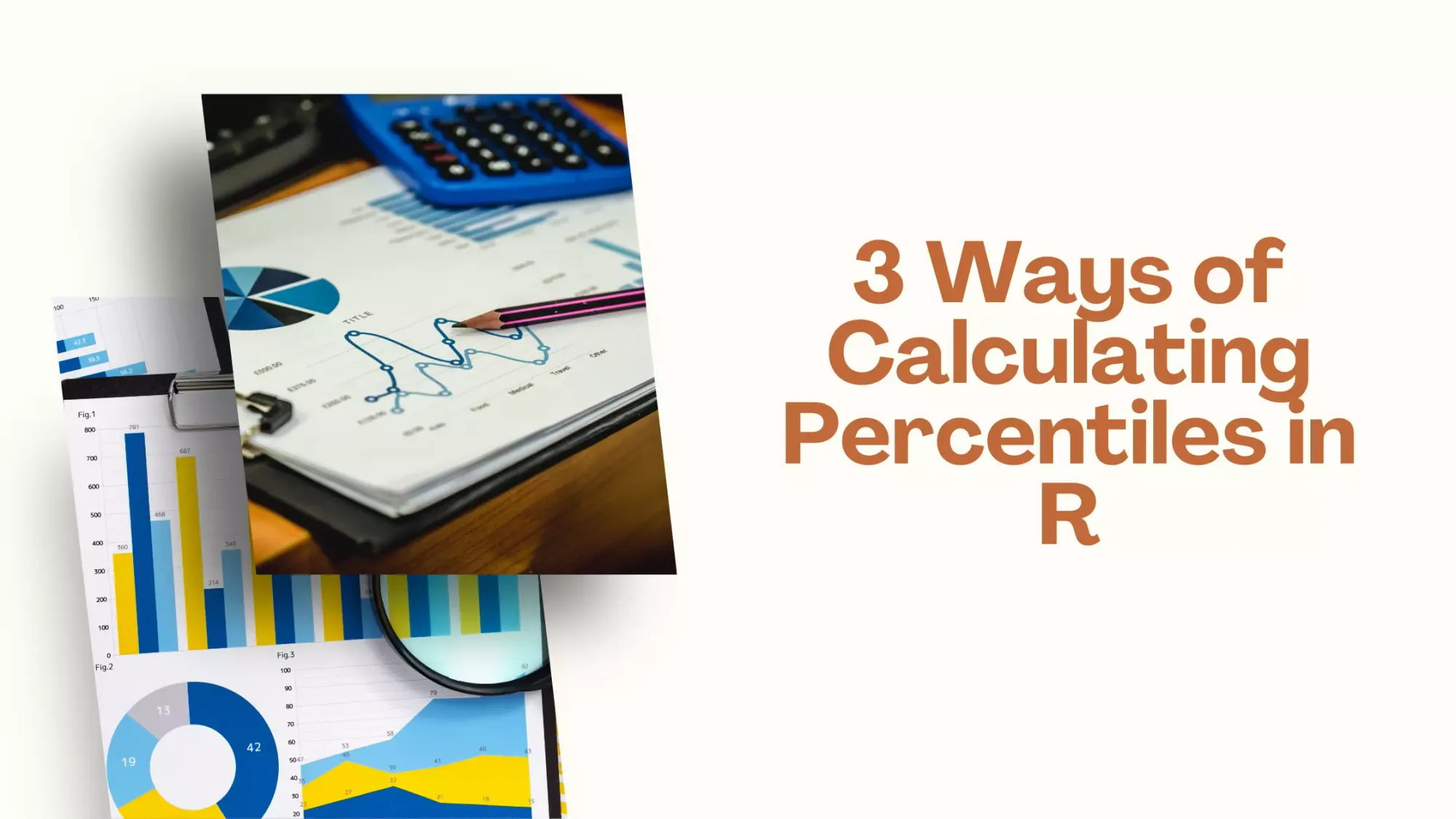
Percentiles, a pivotal tool in the world of statistics, represent a measure that tells us what proportion of a dataset falls below a particular value. In statistical analysis, percentiles are used to understand and interpret data by providing a means to compare individual data points to the rest of the dataset.
One commonly used percentile is the median, which is the 50th percentile and splits the data into two equal parts. The 25th and 75th percentiles, also known as the first and third quartiles, demarcate the interquartile range, which contains the central 50% of the data.
R, a powerful and popular language for statistical computing, offers a wide range of functions and packages for calculating percentiles. In this article, we'll explore the different ways of calculating percentiles in R, including base R functions and packages such as Hmisc and quantile function, as well as user-defined functions for unique percentile calculations.
What is a percentile?
Percentiles are a statistical measure that represents a position in a sorted, numerical dataset. They split the data into 100 equal parts and are thus used to understand and compare the relative standing of a value within a dataset.
The concept of percentiles is commonly used in a wide array of statistical applications, including the social, business, health, and physical sciences. It helps in determining the relative position of a value, whether it's a student's grade among their classmates, a company's profit relative to its competitors, or a person's blood pressure compared to a population sample.
Defining Percentiles
The Nth percentile of a dataset is a value such that at least N percent of the data values are less than or equal to it, and at least (100 - N) percent of the data values are greater than or equal to it. For instance, if a student's score is in the 85th percentile, it means that 85% of students scored less than or equal to this student's score, and 15% of students scored more than this student's score.
Key Percentiles: Quartiles and Median
While percentiles can range from 1 to 99, there are a few key percentiles that are often used in statistical analysis:
- Median (50th percentile): The median is a measure of central tendency, it splits the dataset into two equal halves. Half of the data falls below the median, and half of the data falls above it. It is less affected by outliers and skewed data compared to the mean (average).
- First Quartile (25th percentile) and Third Quartile (75th percentile): The first and third quartiles, often denoted as Q1 and Q3, respectively, split the data into four equal parts. Q1 represents the median of the lower half of the dataset (excluding the median if the dataset has an odd number), and Q3 represents the median of the upper half of the dataset (again excluding the median if the dataset has an odd number).
Interquartile Range (IQR)
The Interquartile Range (IQR) is a measure of statistical dispersion and is calculated as the difference between the 75th and 25th percentiles, i.e., Q3 - Q1. It provides a measure of where the "middle half" of the data falls, i.e., it gives the range for the middle 50% of the data. It is often used in conjunction with the box-and-whisker plot and is robust against outliers.
Calculating Percentiles
There are different methods for calculating percentiles, and they can sometimes give slightly different results. These methods typically involve sorting the dataset, identifying a specific position or index based on the desired percentile, and potentially interpolating between data points. The precise approach can depend on the specific characteristics of the dataset and the requirements of the analysis. The base R quantile()
function, for example, provides nine different methods for calculating percentiles, based on various statistical texts.
Base R Function: quantile()
The quantile()
function is the simplest and most straightforward way to calculate percentiles in R. Here's an example of how it's used:
# Create a numeric vector
data_vector <- c(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
# Calculate the 30th percentile
perc_30 <- quantile(data_vector, probs = 0.30)
# Print the result
print(perc_30)
In this example, the quantile()
function calculates the 30th percentile of the data_vector. The probs argument specifies the percentiles to compute in decimal form.
In R, the quantile()
function offers nine types of methods to compute quantiles, also known as percentiles. Each type represents a different algorithm for percentile calculation. These methods give different results especially when dealing with smaller datasets. Here's a brief overview of each type:
- Type 1: This method uses the inverse of the empirical cumulative distribution function (ECDF), with jumps in the ECDF occurring to the left of the data points. This is also known as the "inverted empirical distribution function" method.
- Type 2: Similar to type 1, but with jumps in the ECDF occurring to the right of the data points. This method results in the same output as type 1 for ordered v, except that type 2 is continuous from the right but not the left.
- Type 3: This method corresponds to SAS definition of quantile, labeled as "SAS-3" or "OBS=5". The ECDF jumps at the data points and the function is continuous from the left.
- Type 4: It is similar to type 3 but the ECDF is continuous from the right. This method corresponds to the method labeled "OBS=8" in SAS.
- Type 5: This method follows the piecewise linear function where the distances to the nearest data points are tied at the median. This method is similar to "Excel", "Ranks", and "Weibull" methods.
- Type 6: This method follows a piecewise linear function where the ratio of the distances to the nearest data points is tied at the median. This method is the recommended one by Hyndman and Fan (1996) and is used by the R function
quantile()
as the default. - Type 7: Similar to type 6, but with averaging at discontinuities. This method is based on the mode of the density of order statistics.
- Type 8: This method follows the piecewise linear function where the ratio of the distances to the nearest data points on the left and right are tied at one. This method is also known as "Median-Unbiased Estimation".
- Type 9: Similar to type 8 but with averaging at discontinuities. This is based on maximizing symmetry in the density of the order statistics.
When calculating percentiles, it's crucial to understand the nuances of each method and choose the most suitable one for your specific analysis. For instance, if you are working with a small dataset and need to minimize bias, type 8 or 9 might be the best choice. On the other hand, for large datasets, the differences between the methods become less significant, and the default type 6 could be sufficient.
The Hmisc Package: wtd.quantile()
While the base R quantile()
function is quite robust, it doesn't support the calculation of weighted percentiles. For this, we can use the wtd.quantile()
function from the Hmisc package.
# Install and load the Hmisc package
install.packages("Hmisc")
library(Hmisc)
# Create a numeric vector and a weights vector
data_vector <- c(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
weights_vector <- c(1, 2, 3, 4, 5, 5, 4, 3, 2, 1)
# Calculate the weighted 30th percentile
perc_30 <- wtd.quantile(data_vector, weights = weights_vector, probs = 0.30)
# Print the result
print(perc_30)
In this example, each data point in data_vector is assigned a weight from the weights_vector, affecting the calculation of the percentile.
This function can be particularly useful in scenarios where the data points are not equally representative or important. For instance, in survey data where responses are adjusted to reflect the demographic makeup of a population, or in financial data where certain transactions carry more weight than others.
Here's a basic example of using the wtd.quantile()
function:
# Install and load the Hmisc package
install.packages("Hmisc")
library(Hmisc)
# Create a numeric vector and a weights vector
data_vector <- c(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
weights_vector <- c(1, 2, 3, 4, 5, 5, 4, 3, 2, 1)
# Calculate the weighted 30th percentile
perc_30 <- wtd.quantile(x = data_vector, weights = weights_vector, probs = 0.30)
# Print the result
print(perc_30)
In this example, each data point in data_vector
is assigned a weight from the weights_vector
, affecting the calculation of the percentile. The probs
argument specifies the percentile to compute as a decimal.
It's important to remember that the weights should correspond to the data points in the dataset. That is, if you have n
data points, you should also have n
weights.
The wtd.quantile()
function provides a flexible and powerful tool for percentile calculation when dealing with weighted data. It is also equipped to handle missing data (NA values) in the dataset or the weights. By default, these are omitted with a warning, but this behavior can be modified with the na.rm
argument. For example, wtd.quantile(x, weights, na.rm = TRUE)
will silently omit any missing values.
User-Defined Function for Percentile Calculation
If the built-in R functions don't satisfy your needs, you can define your own function for percentile calculations. Here's an example:
# Define a function to calculate the nth percentile
calc_percentile <- function(data_vector, percentile) {
data_sorted <- sort(data_vector)
index <- percentile * (length(data_vector) + 1)
lower <- floor(index)
upper <- ceiling(index)
if (lower == upper) {
return(data_sorted[lower])
}
else {
fractional <- index - lower
return(data_sorted[lower] + fractional * (data_sorted[upper] - data_sorted[lower]))
}
}
# Create a numeric vector
data_vector <- c(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
# Use the function to calculate the 30th percentile
perc_30 <- calc_percentile(data_vector, 0.30)
# Print the result
print(perc_30)
This function sorts the input data, then calculates the index of the percentile based on the length of the data. If the index isn't an integer, the function linearly interpolates between the nearest two values.
Conclusion
Whether it's understanding the spread of a dataset, comparing individual values to a larger context, or simply calculating the median, percentiles are a critical tool for anyone conducting statistical analysis. The R programming language provides various techniques to calculate percentiles, each serving different needs and offering different levels of flexibility. The built-in quantile()
function, the wtd.quantile()
function from the Hmisc package, and user-defined functions each have their own strengths and use cases. Understanding when to use each one can greatly enhance your statistical analysis in R.
Further reading
quantile()
function: This page provides detailed information about thequantile()
function in R, including a description of the function, its usage, arguments, details, and examples.- Hmisc package: This page provides an overview of the Hmisc package, including a description of the package and links to the documentation for the individual functions within the package.
wtd.quantile()
function: This page provides detailed information about thewtd.quantile()
function in the Hmisc package, including a description of the function, its usage, arguments, details, and examples.- How to calculate percentiles in Python
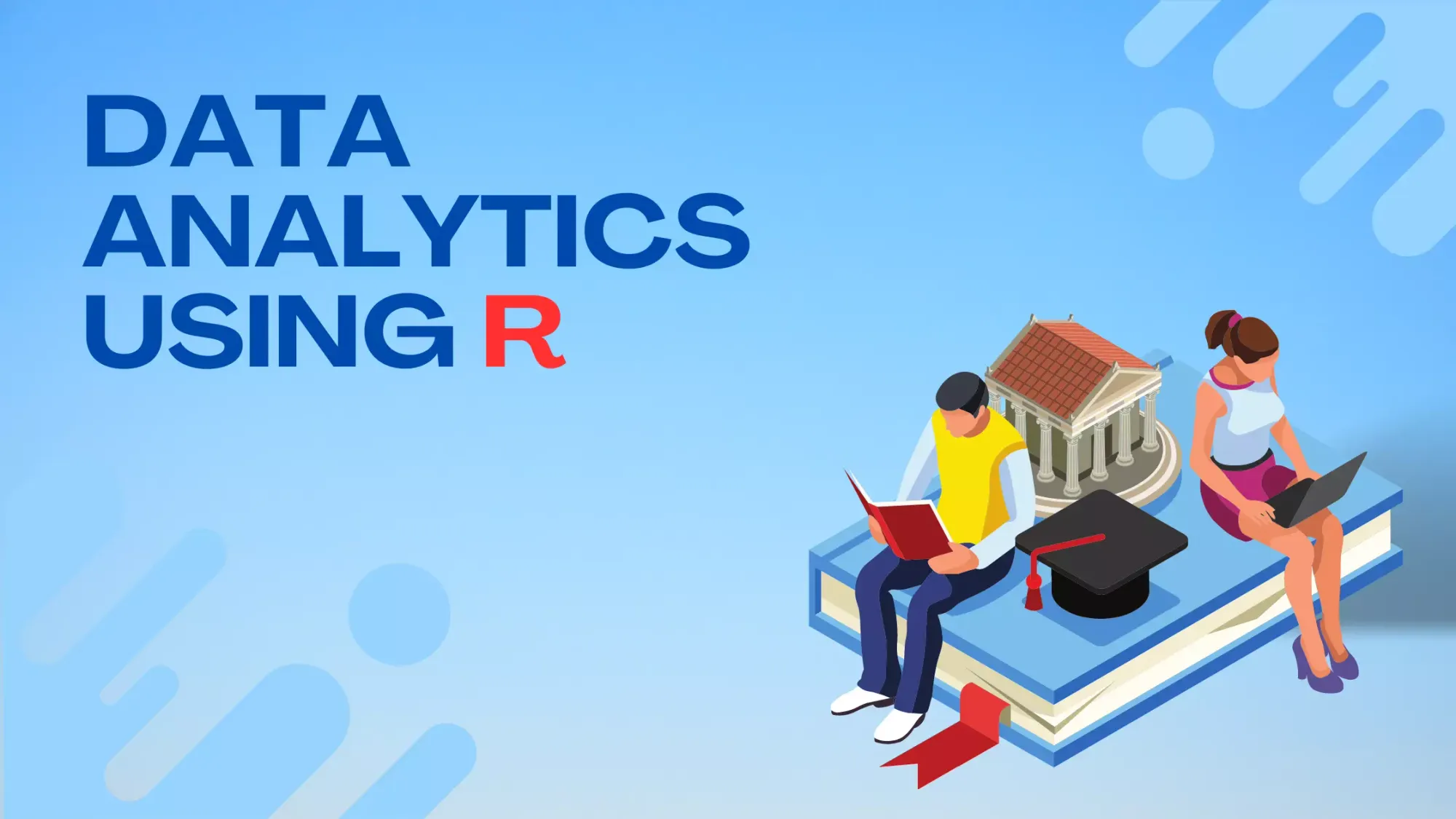